How to Fix the NameError: Input Name Is Not Defined in Python
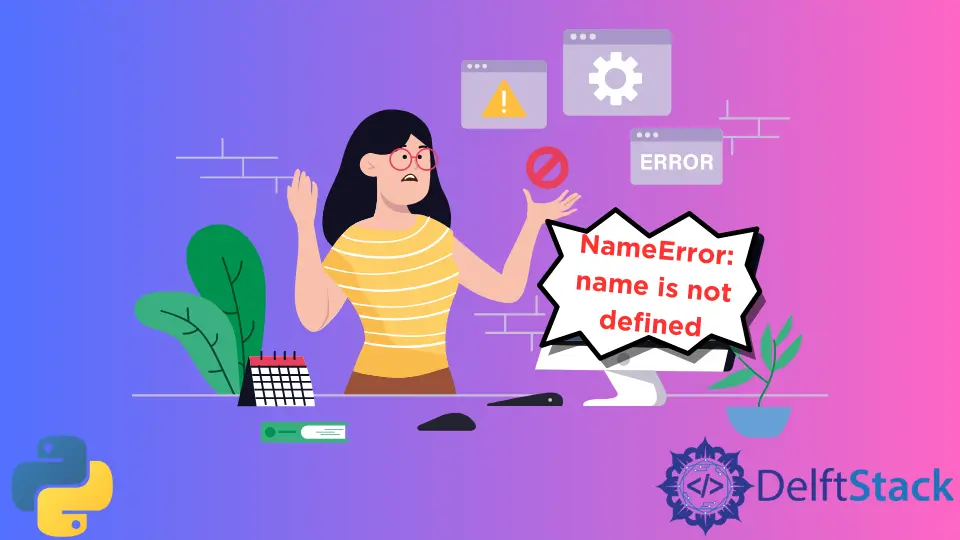
In Python 2.7 and 2.x versions, the raw_input()
function and the input()
were the two built-in functions to take the user’s input. But in the later versions of Python 3,0, the raw_input()
was renamed as input()
, which is now still in use.
Fix the NameError: input name is not defined
in Python
In the older version of Python, the input
function was used to evaluate the Python expression, but if you want to read strings, then the raw_input
was used for that purpose. But now, the raw_input
function is renamed as input, so it won’t work in 3.x versions of Python.
Let’s understand it through an example.
We are using Python version 2.7 for the sake of this topic. If you use this on the 3.x version of Python, this code will be executed without errors.
# Python 2.7 version
name = input("Hi! What is your good name? ")
print("Nice to meet you " + name)
Output:
NameError: name 'Zeeshan' is not defined
The above code has caused a Name Error because input wasn’t used to read the string in the older version of Python but to evaluate Python expression. And to fix this Name Error, we can use the raw_input
function because it was built to read strings.
Let’s fix the Name Error with the raw_input
function.
name = raw_input("Hi! What is your good name? ")
print("Nice to meet you " + name)
Output:
Hi! What is your good name? Nice to meet you Zeeshan
As you can see, the raw_input
function has fixed the Name Error and executed the program smoothly.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python