How to Initialize an Empty List in Python
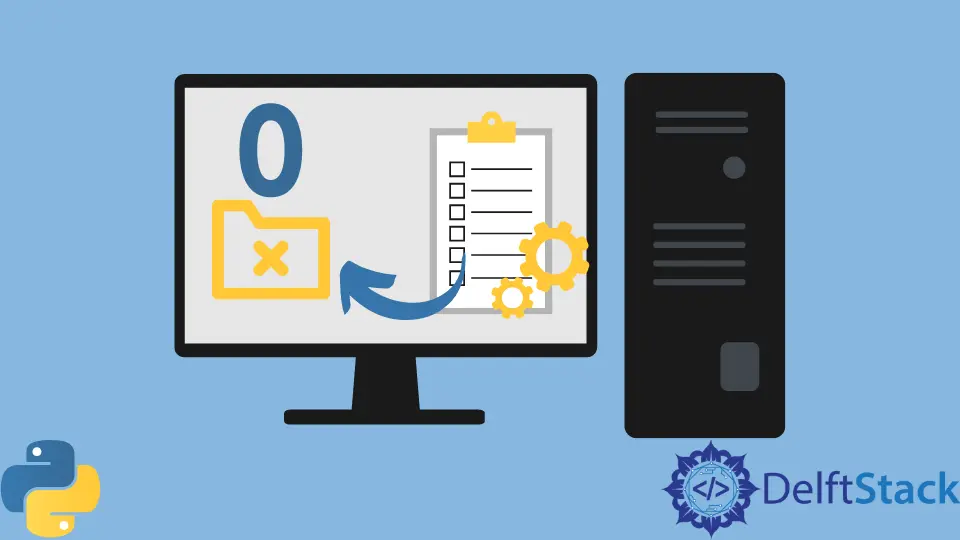
This tutorial demonstrates ways on how to initialize an empty list in Python. This will cover defining a list of size n
or defining a variable-sized list with the values all defaulting to empty.
When you declare a list in Python, it always starts off at size 0
since there is no explicit way of declaring a Python list’s size since Python lists are variable in nature. This tutorial will provide a way to initialize a fixed-size list with all the values defaulted to None
or empty.
Initializing n
-Sized None
Values to a List in Python
The best way to solve this problem is to initialize an n
-Sized list with the values all set to None
, which indicates that a value is empty in Python.
To do this, initialize a singleton list with the value None
and multiply it by n
, which is the list’s preferred size.
n = 15 # Size of the List
lst = [None] * n
print(lst)
Output:
[None, None, None, None, None, None, None, None, None, None, None, None, None, None, None]
The output is a list of size 15 as defined by n
with all the values set to None
, equivalent to an empty value.
This solution is the easiest and most practical way to initialize a list with empty values in Python. However, this is a workaround to the predefined nature of a Python list being variable in size.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python