How to Import a Variable From Another File in Python
-
the
import
Statement in Python -
the
from import
Statement in Python -
the
sys
Module in Python -
the
sys.path.append()
Method in Python - Use These Programs to Import Variables From Another File in Python
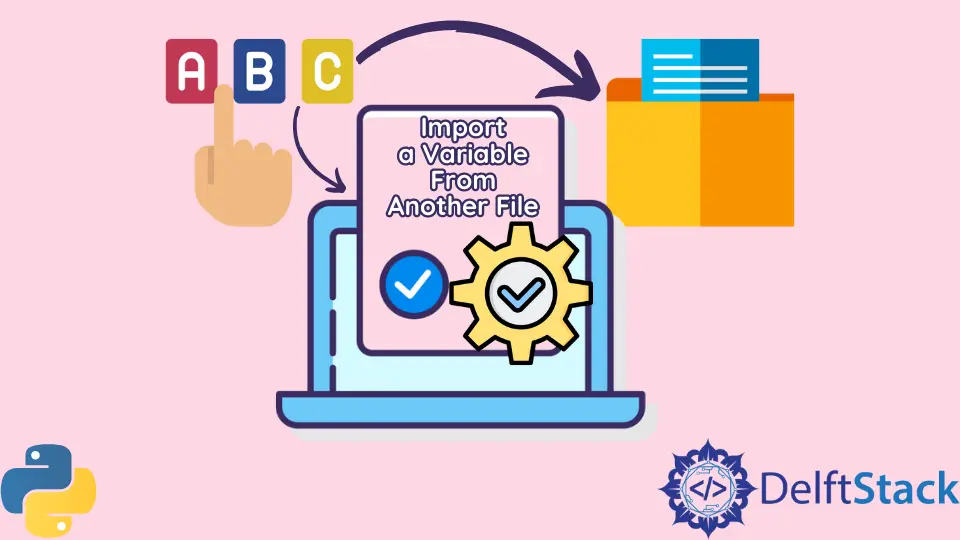
There might be cases when Python codes are very complex, and you need to print certain variables and values separately in another file. By doing this, one can read the values stored in specific variables more efficiently.
This tutorial will demonstrate how to import variables from another file in Python.
the import
Statement in Python
The import
statement in Python is very important because it invokes or defines any module in a Python code. By calling this statement, we can use all the functions and methods present in an imported Python module.
In the import
statement, one can also import a file as a module and access all the Python file contents from another Python file.
the from import
Statement in Python
Python’s from import
statement lets a user import specific contents or attributes of a file or a module in Python from another Python file.
the sys
Module in Python
The sys
module in Python is used to provide various functions and methods that are used to deal with the runtime environment of Python and various parts of the Python interpreter.
the sys.path.append()
Method in Python
The sys.path.append()
method of the sys
module helps the user to include a specific file in the program by passing the path to that file. Mentioning the path of that file makes it easy for the Python interpreter to reach that file easily.
Use These Programs to Import Variables From Another File in Python
Let us assume the following Python file integer.py
.
a = 5
b = 10
Now, suppose we have to import the value of the variable a
, i.e., 5
in a different Python file. We run the following Python code.
By using the import
statement:
import integer as i
import sys
sys.path.append("/downloads/integer")
print(i.a)
Output:
5
- By using the
from import
statement:
from integer import a
import sys
sys.path.append("/downloads/integer")
print(a)
Output:
5
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn