How to Import Module From Subdirectory in Python
- Understanding Python’s Import System
-
Method 1: Using the
__init__.py
file -
Method 2: Modifying the
sys.path
- Method 3: Using Relative Imports
- Conclusion
- FAQ
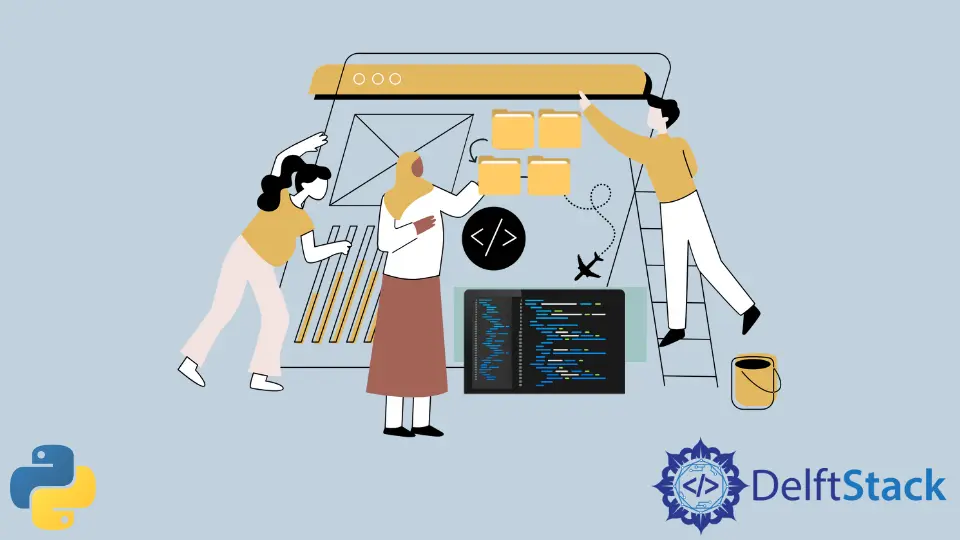
Importing modules from subdirectories in Python can seem tricky at first, but once you understand the structure of your project, it becomes much simpler.
This tutorial will guide you through the process of importing a file from a subdirectory, ensuring that you can effectively organize your codebase without running into import errors. Whether you’re working on a small project or a larger application, knowing how to manage your imports is crucial for maintaining clean and efficient code. Let’s dive into the various methods you can use to import modules from subdirectories in Python.
Understanding Python’s Import System
Before we explore the methods of importing modules from subdirectories, it’s essential to grasp how Python’s import system works. When you use the import
statement, Python looks for the specified module in several locations, including the current directory and the directories listed in the sys.path
variable. By organizing your code into subdirectories, you can create a more modular structure, but you’ll need to ensure that Python can find these modules.
Method 1: Using the __init__.py
file
One of the most common ways to import a module from a subdirectory is by using an __init__.py
file. This file can be empty or contain initialization code for the package. By having this file in your subdirectory, you effectively tell Python that the directory should be treated as a package.
- Create a directory structure as follows:
project/
main.py
subdirectory/
__init__.py
my_module.py
- In
my_module.py
, define a simple function:
def greet():
return "Hello from my_module!"
- In
main.py
, import the module like this:
from subdirectory.my_module import greet
print(greet())
Output:
Hello from my_module!
By creating an __init__.py
file in the subdirectory
, you allow Python to recognize it as a package. This enables you to use dot notation to import the my_module
module directly into your main script. The greet
function is then called, demonstrating successful import and execution.
Method 2: Modifying the sys.path
Another method to import modules from subdirectories is to modify the sys.path
variable. This approach is particularly useful when you don’t want to create an __init__.py
file or when you’re working in a temporary environment.
- Create a directory structure similar to the previous example:
project/
main.py
subdirectory/
my_module.py
- In
my_module.py
, define a function:
def greet():
return "Hello from my_module!"
- In
main.py
, modify thesys.path
and import the module:
import sys
import os
sys.path.append(os.path.join(os.path.dirname(__file__), 'subdirectory'))
import my_module
print(my_module.greet())
Output:
Hello from my_module!
In this method, we first import the sys
and os
modules. We then append the path of the subdirectory
to sys.path
, which allows Python to locate my_module.py
. After that, we can import my_module
directly and call the greet
function. This method is particularly useful for quick scripts or when dealing with temporary directories.
Method 3: Using Relative Imports
If you’re working within a package structure, relative imports can be an efficient way to import modules from subdirectories. This method is applicable when your script is part of a larger package.
- Set up your directory structure like this:
project/
main.py
subdirectory/
__init__.py
my_module.py
- In
my_module.py
, define a function:
def greet():
return "Hello from my_module!"
- In
main.py
, use a relative import:
from .subdirectory.my_module import greet
print(greet())
Output:
Hello from my_module!
Using relative imports allows you to navigate the package structure without needing to specify the full path. The dot (.
) indicates that you’re starting from the current package level. This method is particularly useful for larger projects where you want to maintain a clear hierarchy and avoid hardcoding paths.
Conclusion
Importing modules from subdirectories in Python is a fundamental skill that can greatly enhance your coding efficiency. Whether you choose to use __init__.py
files, modify sys.path
, or utilize relative imports, each method has its advantages depending on your project’s structure. By following these methods, you can keep your code organized and maintainable, making collaboration and future development much easier. With practice, importing modules will become second nature, allowing you to focus more on writing great code.
FAQ
-
How do I know if I need an
__init__.py
file?
An__init__.py
file is necessary if you want Python to recognize a directory as a package. It can be empty or contain initialization code. -
Can I import a module without modifying
sys.path
?
Yes, you can import a module without modifyingsys.path
by ensuring the module is located in the same directory as your main script or in a directory already included insys.path
. -
What are relative imports, and when should I use them?
Relative imports allow you to import modules based on their location relative to the current module. They are best used within packages to maintain a clean and organized code structure. -
Is there a performance difference between these methods?
Generally, the performance difference is negligible for most applications. However, using__init__.py
files and relative imports can make your code cleaner and easier to manage. -
Can I import modules from multiple subdirectories?
Yes, you can import modules from multiple subdirectories by following the same methods outlined above for each subdirectory.