How to Import Modules From Parent Directory in Python
- Import a Module From the Parent Directory in Python Using the Relative Import
-
Import a Module From the Parent Directory in Python by Adding It to
PYTHONPATH
-
Import a Module From the Parent Directory in Python Using the
sys.path.insert()
Method
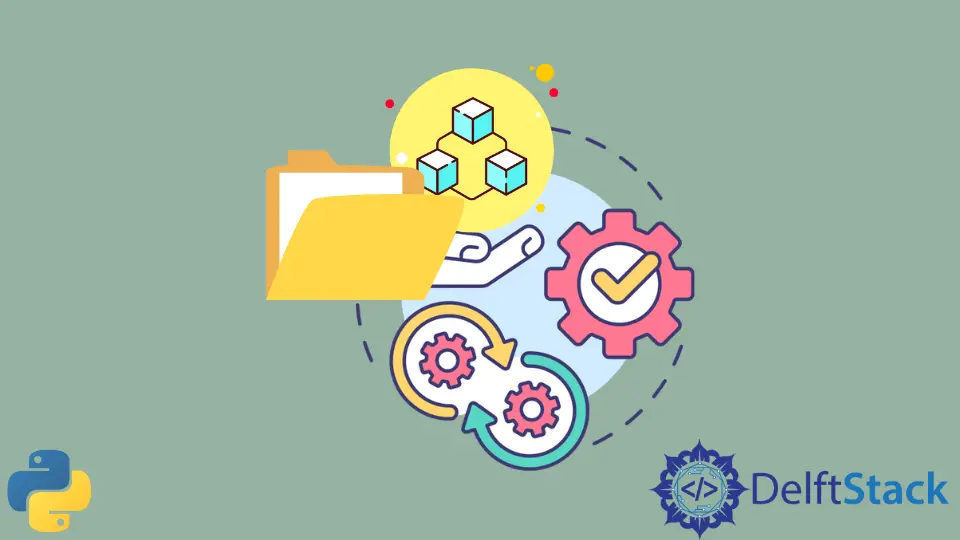
This tutorial will explain various methods to import a module from the parent directory in Python. We import different modules to use other functionalities in our code. It saves us from copying or implementing the functionalities again and makes the code clean and easier to understand.
Suppose we want to import a module from the parent directory of the current project directory. We can do so using different methods, which are explained below.
Import a Module From the Parent Directory in Python Using the Relative Import
The relative import is used to import a module in the code using the current directory path as a reference. To import a module using the import
statement, we will first have to declare the directory a package by adding the __init__.py
file in the parent directory. Once the parent directory is declared a package, we can import the module using the relative package approach.
Suppose we have the following directory tree.
parent_parent_directory/
parent_directory/
mymodule.py
__init__.py
current_directory/
currentmodule.py
mymodule.py
__init__.py
The below example code demonstrates how to import the module from the parent package.
from ..parent_directory import mymodule
To import the module from the directory two levels above from the current directory, we will have to put three dots before the package directory name to go two levels back, as shown in the below example code.
from ...parent_parent_directory import mymodule
Import a Module From the Parent Directory in Python by Adding It to PYTHONPATH
The PYTHONPATH
is an environment variable specifying the directories list that Python should look to import modules and packages.
So if we add the parent directory from where we need to import the module, Python will automatically look into the parent directory and find the required module.
Import a Module From the Parent Directory in Python Using the sys.path.insert()
Method
We can also use the sys.path.insert()
method to add the parent directory to the sys.path
list, which is the list of strings that specifies the paths to look for the packages and modules. The sys.path
contains the PYTHONPATH environment variable directory list, and other paths can also be added using the sys.path.insert()
method.
The below example code demonstrates how to use the sys.path.insert()
method to add the parent directory to the sys.path
list in Python.
import mymodule
import os
import sys
p = os.path.abspath(".")
sys.path.insert(1, p)