How to Create Identity Matrix With Python
- Method 1: Using NumPy
- Method 2: Using a List Comprehension
-
Method 3: Using the
scipy
Library - Conclusion
- FAQ
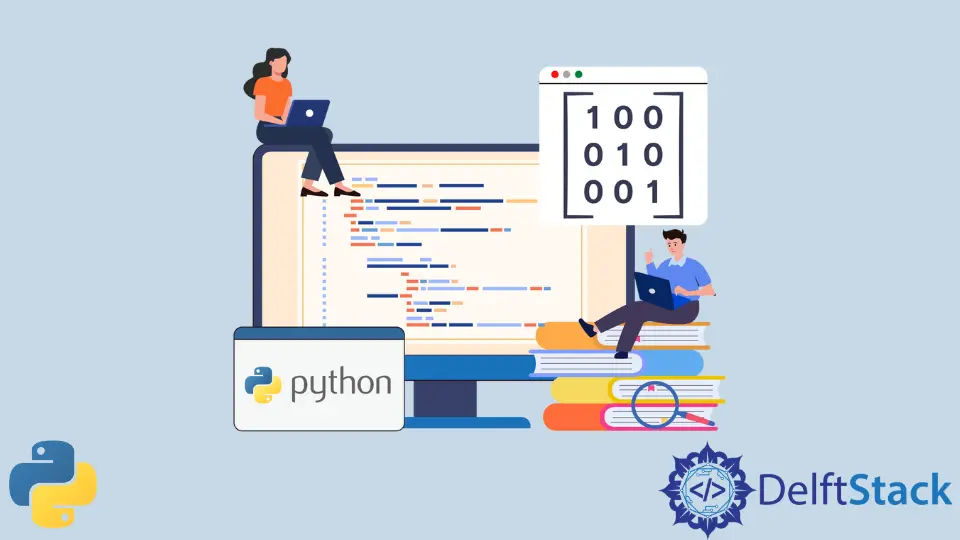
Creating an identity matrix is a fundamental operation in linear algebra and programming. Whether you’re dealing with machine learning, computer graphics, or scientific computations, knowing how to generate an identity matrix in Python can be incredibly useful.
In this article, we’ll explore various methods to create an identity matrix using Python, including popular libraries like NumPy and pure Python implementations. By the end, you’ll have a solid understanding of how to generate identity matrices efficiently, along with clear code examples to guide you through the process. So, let’s dive in and discover how to create identity matrices in Python!
Method 1: Using NumPy
One of the simplest and most efficient ways to create an identity matrix in Python is by using the NumPy library. NumPy is a powerful library for numerical computations that provides a wide range of mathematical functions. The numpy.eye()
function allows you to create an identity matrix with ease.
Here’s how you can do it:
pythonCopyimport numpy as np
size = 4
identity_matrix = np.eye(size)
print(identity_matrix)
Output:
textCopy[[1. 0. 0. 0.]
[0. 1. 0. 0.]
[0. 0. 1. 0.]
[0. 0. 0. 1.]]
This code begins by importing the NumPy library. We then define the size of the identity matrix we want to create. In this case, we set it to 4, which means we will generate a 4x4 identity matrix. The np.eye(size)
function generates the identity matrix, where the diagonal elements are set to 1, and all other elements are set to 0. Finally, we print the resulting identity matrix to the console. Using NumPy not only simplifies the process but also optimizes performance for larger matrices.
Method 2: Using a List Comprehension
If you prefer not to use external libraries, you can create an identity matrix using pure Python with a list comprehension. This method is straightforward and allows you to understand the underlying mechanics of matrix creation.
Here’s how you can implement it:
pythonCopysize = 4
identity_matrix = [[1 if i == j else 0 for j in range(size)] for i in range(size)]
for row in identity_matrix:
print(row)
Output:
textCopy[1, 0, 0, 0]
[0, 1, 0, 0]
[0, 0, 1, 0]
[0, 0, 0, 1]
In this code, we define the size of the identity matrix as 4. We then use a nested list comprehension to create the matrix. The outer loop iterates through each row, while the inner loop populates each row with 1s and 0s. If the row index (i
) matches the column index (j
), we place a 1; otherwise, we place a 0. Finally, we print each row of the identity matrix. This method is lightweight and effective for small matrices, making it a good choice for educational purposes.
Method 3: Using the scipy
Library
Another approach to creating an identity matrix is by using the scipy
library, which is built on top of NumPy and offers additional functionality for scientific computing. The scipy.sparse.eye()
function can create a sparse identity matrix, which is particularly useful when dealing with large matrices that contain a lot of zeros.
Here’s how you can use it:
pythonCopyfrom scipy.sparse import eye
size = 4
identity_matrix = eye(size).toarray()
print(identity_matrix)
Output:
textCopy[[1. 0. 0. 0.]
[0. 1. 0. 0.]
[0. 0. 1. 0.]
[0. 0. 0. 1.]]
In this example, we first import the eye
function from the scipy.sparse
module. We define the size of the identity matrix as 4, just like in previous methods. The eye(size)
function generates a sparse matrix, which is then converted to a dense array using .toarray()
. Finally, we print the resulting identity matrix. This method is particularly advantageous when working with very large matrices, as it conserves memory by only storing non-zero values.
Conclusion
Creating an identity matrix in Python is a straightforward task, whether you choose to use a library like NumPy or implement it using pure Python. Each method has its own advantages, depending on your specific needs, such as performance or memory efficiency. By mastering these techniques, you’ll be well-equipped to handle various applications in linear algebra and data science. So go ahead and experiment with these methods to find the one that best suits your project!
FAQ
-
What is an identity matrix?
An identity matrix is a square matrix in which all the elements of the principal diagonal are ones, and all other elements are zeros. -
Why are identity matrices important?
Identity matrices serve as the multiplicative identity in matrix multiplication, meaning that when any matrix is multiplied by an identity matrix, it remains unchanged. -
Can I create a non-square identity matrix?
No, identity matrices are always square matrices, meaning they have the same number of rows and columns. -
Is NumPy necessary to create an identity matrix in Python?
No, you can create an identity matrix using pure Python without any external libraries, although using NumPy is more efficient for larger matrices. -
How do I create a larger identity matrix?
Simply change the size parameter in the methods provided to the desired dimension, such as 10 for a 10x10 identity matrix.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn