If a goto Statement Exists in Python
-
Use Exceptions to Emulate a
goto
Statement in Python -
Use Loops With
break
andcontinue
Statements to Emulate agoto
Statement in Python
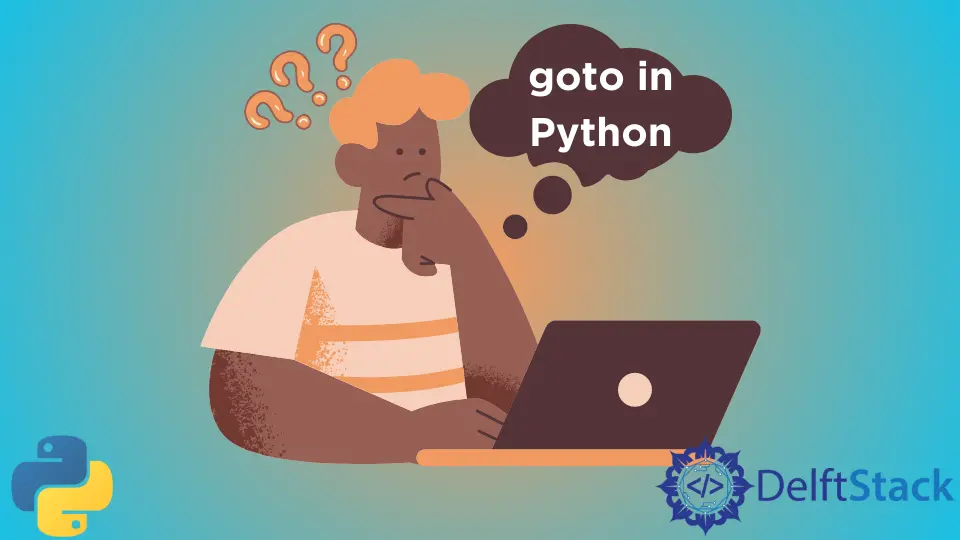
This article provides you with the answer if the goto
statement exists or not in Python.
Basically, goto
statements are not supported in Python. Generally, these statements are widely despised because they result in very unorganized code; thus, ending up in spaghetti code. Such code becomes hard to understand and trace back while trying to understand flows and debugging.
Python enables structured programming by using several ways to branch code, such as using if-else
expressions, exceptions, and loops.
If you want to emulate a goto
statement in Python, this article provides some examples. However, these methods are not recommended as it is a bad programming practice to use goto
.
Use Exceptions to Emulate a goto
Statement in Python
You could use exceptions to provide a structured way of implementing goto
, even though it is not a recommended programming practice. After all, exceptions can jump out of deeply nested control structures. Check this example below.
class gotolabel(Exception):
print("from the goto label") # declare a label
try:
x = 4
if x > 0:
raise gotolabel() # goto the label named "gotolabel"
except gotolabel: # where to goto the label named "gotolabel"
pass
Output:
from the goto label
Use Loops With break
and continue
Statements to Emulate a goto
Statement in Python
You could use loops with break
, and continue
statements to emulate a goto
statement in Python. This example program demonstrates this method.
prompt = "Roll the dice "
while True:
try:
y = int(input(prompt))
except ValueError:
print("Please enter a valid number")
continue
if y > 6:
prompt = "The dice has numbers 1-6 ! Input a number <6"
elif y < 1:
prompt = "The dice has numbers 1-6 ! Input a number >1"
else:
print("Correct!")
break
Output:
Roll the dice hj
Please enter a valid number
Roll the dice 6
Correct!
Here, the continue
statement helps the process jump to the next iteration of the loop and cause an infinite loop. On the other hand, the break
statement helps terminate the loop.