The getpass Module in Python
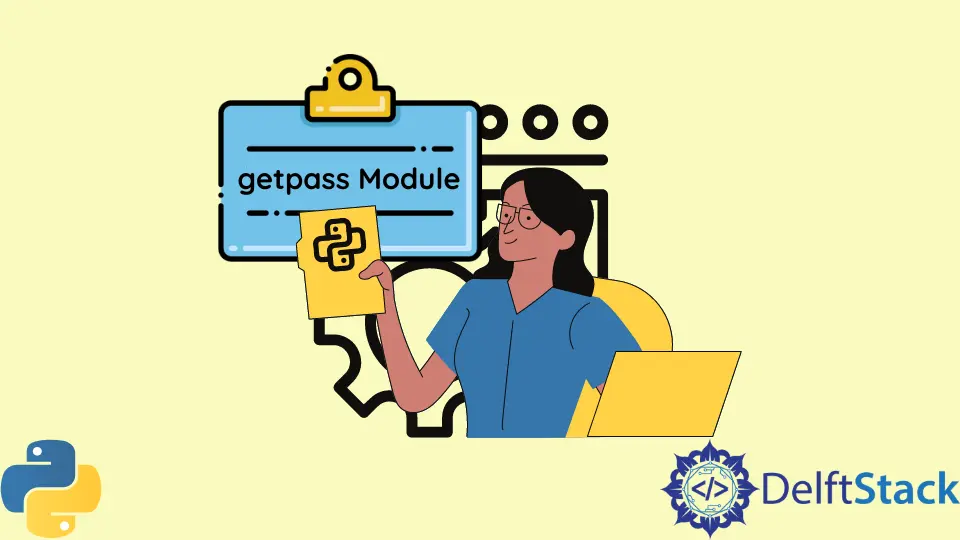
This tutorial will discuss a secure way to input passwords from a user in Python.
Use the getpass.getpass()
Function in Python
The getpass
module in Python provides a secure method for retrieving the password from the user through a command prompt. The getpass()
function inside the getpass module prompts the user to enter the password but hides the figures entered by the user.
It takes the prompt text as its input parameter and returns the data entered by the user.
Example:
import getpass
password = getpass.getpass(prompt="Please input Password : ")
print("Password:", password)
Output:
Please input Password : ··········
Password: 12345
As seen in the output, the figures entered by the user are hidden while typing.
Use the getpass.getuser()
Function in Python
We can also get the username of the currently logged-in user with the getpass
module in Python.
The getuser()
function returns the current user’s username, and it checks the LOGNAME
, USER
, LNAME
, and USERNAME
environment variables. It also returns the first non-empty value that it finds.
Example:
import getpass
user = getpass.getuser()
print("Current User =", user)
Output:
Current User = root
We retrieved the username of the currently logged-in user, which happens to be root
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn