How to Get the Monitor Resolution in Python
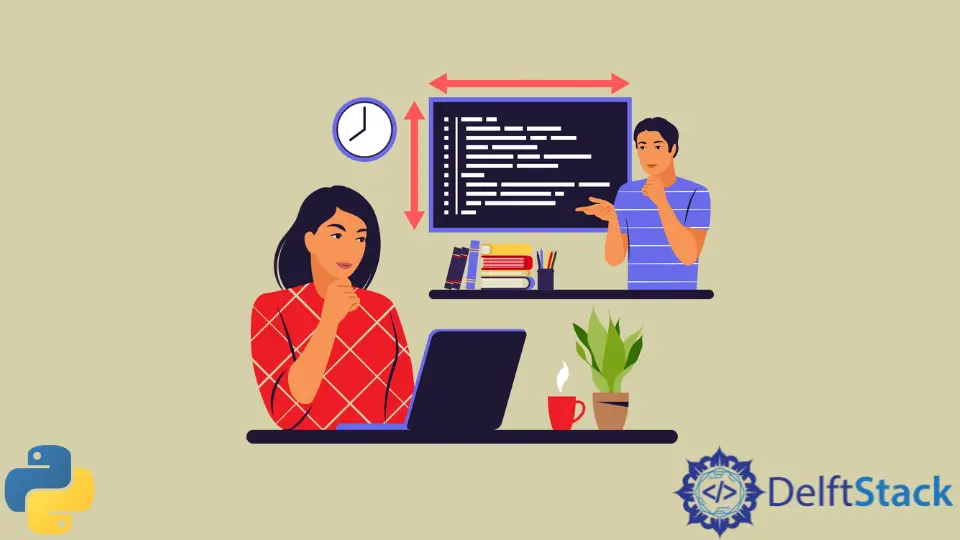
Many operations involve developing GUI in Python to interact with applications and operating systems. So to perform these kinds of procedures, Python provides multiple packages and libraries. One of the tasks that include the GUI is getting the resolution of the monitor.
This tutorial will show you a method on how to get the resolution of the monitor in Python.
the Use of the Tkinter
Library in Python
The Tkinter
package is Python’s GUI library. This library helps Python by providing a GUI tool kit that helps in developing GUI applications. By using this library, Python can interact with other applications easily. Check this example code below.
from tkinter import *
root = Tk()
monitor_height = root.winfo_screenheight()
monitor_width = root.winfo_screenwidth()
print("width x height = %d x %d (pixels)" % (monitor_width, monitor_height))
mainloop()
Output:
width x height = 1440 x 900 (pixels)
In the program above, we import the tkinter
library first. Then, in the next step, we create a root window
, which is basically the main application window in the applications. You must define this window before performing any operation on any application.
After that, we get the dimensions of the monitor by using the root.winfo_screenheight()
and root.winfo_screenwidth()
methods, respectively. Note that the values returned are in pixels
.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn