How to Get Parent Directory in Python
-
Get the Parent Directory in Python Using the
path.parent()
Method of thepathlib
Module -
Get the Parent Directory in Python Using the
pardir()
Method of theos
Module -
Get the Parent Directory in Python Using the
dirname()
Method of theos
Module
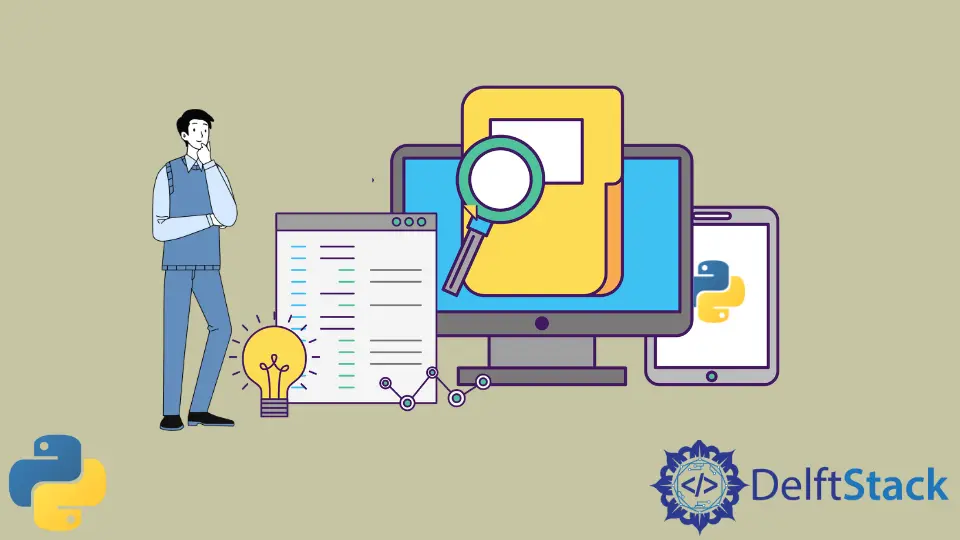
This tutorial will explain various methods to get the parent directory of a path in Python. The parent directory is a directory that is above or higher than the given directory or file. For example, the parent directory of a path C:\folder\subfolder\myfile.txt
is C:\folder\subfolder
. Every directory except the root directory has a parent directory.
Get the Parent Directory in Python Using the path.parent()
Method of the pathlib
Module
The path.parent()
method, as the name suggests, returns the parent directory of the given path passed as an argument in the form of a string. Therefore, to get the parent directory of a path, we need to pass the path string to the path.parent()
method of the pathlib
module.
The example code below demonstrates how to use path.parent()
to get the parent directory of a path in Python:
from pathlib import Path
path1 = Path(r"C:\folder\subfolder\myfile.txt")
path2 = Path(r"C:\Myfile.txt")
print(path1.parent)
print(path2.parent)
Output:
C:\folder\subfolder
C:\
Get the Parent Directory in Python Using the pardir()
Method of the os
Module
os.pardir
is a constant string referring to the parent directory. It is '..'
for Windows and POSIX OS, and '::'
for macOS.
When we combine the given path and os.pardir
in the os.path.join()
method, we could get the parent directory of the given directory.
The example code below demonstrates how to use the os.pardir
and path.join()
method of the os
module to get the parent directory of a path:
import os.path
path1 = r"C:\folder\subfolder\myfile.txt"
path2 = r"C:\Myfile.txt"
print(os.path.abspath(os.path.join(path1, os.pardir)))
print(os.path.abspath(os.path.join(path2, os.pardir)))
Output:
C:\folder\subfolder
C:\
As mentioned above, we could also get the same result if we replace os.pardir
with '..'
if the OS is Windows or POSIX.
import os.path
path1 = r"C:\folder\subfolder\myfile.txt"
path2 = r"C:\Myfile.txt"
print(os.path.abspath(os.path.join(path1, "..")))
print(os.path.abspath(os.path.join(path2, "..")))
Output:
C:\folder\subfolder
C:\
Get the Parent Directory in Python Using the dirname()
Method of the os
Module
The dirname()
method of the os
module takes path string as input and returns the parent directory as output.
The example code below demonstrates how to use the dirname()
to get the parent directory of a path:
import os.path
path1 = Path(r"C:\folder\subfolder\myfile.txt")
path2 = Path(r"C:\Myfile.txt")
print(os.path.dirname(path1))
print(os.path.dirname(path2))
Output:
C:\folder\subfolder
C:
Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python