How to Get the Number of CPUs Using Python
-
Method 1: Using the
os
Module -
Method 2: Using the
multiprocessing
Module -
Method 3: Using the
psutil
Library - Conclusion
- FAQ
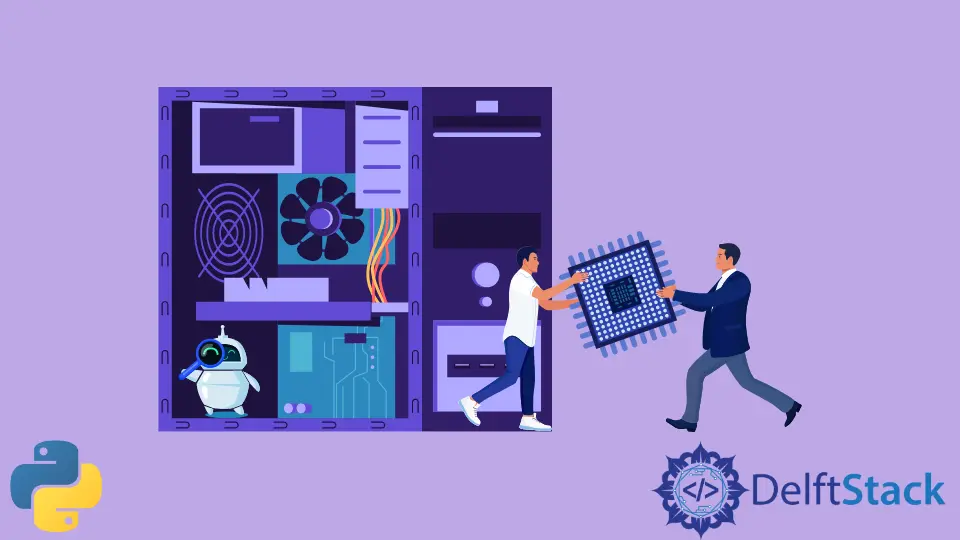
Determining the number of CPUs available on your system can be crucial for optimizing performance, especially when you’re working with Python for data processing or computational tasks. Whether you’re building a web application, running machine learning algorithms, or simply managing system resources, knowing how many CPUs you have can help you make informed decisions about parallel processing and resource allocation.
In this tutorial, we will explore various methods to find the number of CPUs using Python. With clear examples and detailed explanations, you’ll be able to implement these techniques efficiently. Let’s dive in!
Method 1: Using the os
Module
One of the simplest ways to find the number of CPUs in Python is by using the built-in os
module. This method is straightforward and works across different operating systems. The os.cpu_count()
function returns the number of CPUs available on the machine.
Here’s how you can implement this:
import os
cpu_count = os.cpu_count()
print(f"Number of CPUs: {cpu_count}")
Output:
Number of CPUs: 8
The os.cpu_count()
function provides a quick and easy way to get the total number of CPUs. It returns the number of logical CPUs, which includes both physical cores and hyper-threaded cores. This means if your CPU supports hyper-threading, the function will count each logical core separately. This method is efficient and requires minimal code, making it ideal for quick checks or when you need to adapt your application based on available resources.
Method 2: Using the multiprocessing
Module
Another effective way to determine the number of CPUs is by utilizing the multiprocessing
module. This module is particularly useful when you’re looking to implement parallel processing in your applications. The multiprocessing.cpu_count()
function behaves similarly to os.cpu_count()
but is specifically designed for multiprocessing tasks.
Here’s an example of how to use it:
import multiprocessing
cpu_count = multiprocessing.cpu_count()
print(f"Number of CPUs: {cpu_count}")
Output:
Number of CPUs: 8
Using multiprocessing.cpu_count()
is particularly beneficial if you’re planning to utilize the multiprocessing capabilities of Python in your application. This function not only returns the number of logical CPUs but also helps you ensure that your parallel processes are efficiently distributed across available resources. This can lead to significant performance improvements, especially in CPU-bound tasks.
Method 3: Using the psutil
Library
For more advanced use cases, the psutil
library offers a wealth of information about system utilization, including CPU count. This library is not included in the standard Python library, so you’ll need to install it first. Once you have it set up, you can retrieve detailed system information, including the number of CPUs.
Here’s how to use psutil
to find the number of CPUs:
import psutil
cpu_count = psutil.cpu_count(logical=True)
print(f"Number of CPUs: {cpu_count}")
Output:
Number of CPUs: 8
The psutil.cpu_count(logical=True)
method provides the total count of logical CPUs. If you want to get the physical core count, you can call psutil.cpu_count(logical=False)
. This flexibility allows you to gather more specific information about your system’s CPU architecture, which can be particularly useful for performance tuning or when developing applications that require precise resource management.
Conclusion
In this tutorial, we explored several methods to determine the number of CPUs available on your system using Python. From the built-in os
and multiprocessing
modules to the more advanced psutil
library, each method has its unique advantages. Depending on your specific needs—whether it’s a quick check or detailed system analysis—you can choose the approach that best suits your application. Understanding the number of CPUs can significantly enhance your ability to optimize performance and manage resources effectively in your Python projects.
FAQ
-
How do I install the
psutil
library?
You can install thepsutil
library using pip by running the commandpip install psutil
in your terminal. -
Is the number returned by
os.cpu_count()
the same asmultiprocessing.cpu_count()
?
Yes, both methods return the number of logical CPUs available on your system. -
Can I determine the number of physical CPUs using Python?
Yes, usingpsutil.cpu_count(logical=False)
will give you the count of physical CPU cores.
-
Why is it important to know the number of CPUs?
Knowing the number of CPUs helps optimize performance, especially for CPU-bound tasks and parallel processing. -
Are these methods cross-platform?
Yes, the methods discussed work across different operating systems, including Windows, macOS, and Linux.