How to Get the Current Date in Python
- Method 1: Using the datetime Module
- Method 2: Using the date Class
- Method 3: Using the time Module
- Method 4: Using the arrow Library
- Conclusion
- FAQ
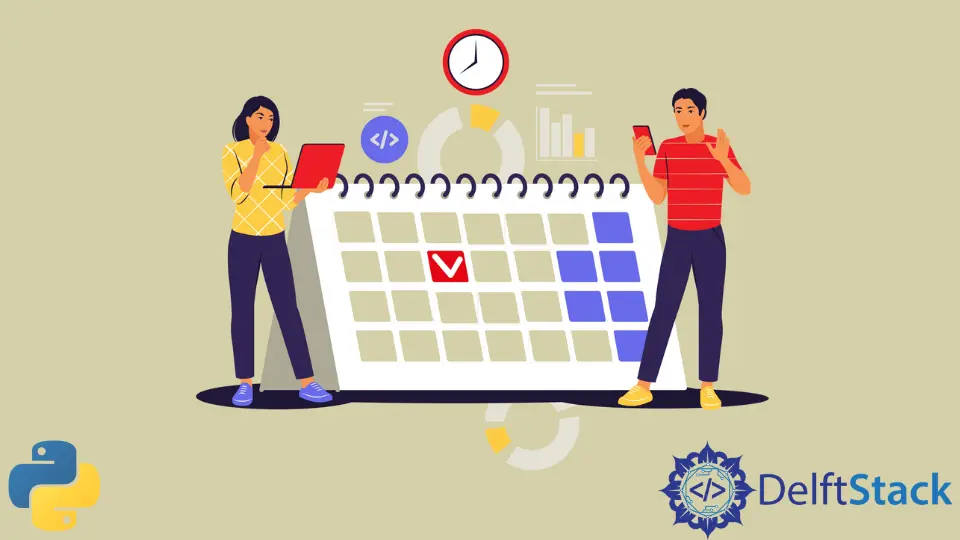
Getting the current date in Python is a common task that many developers encounter. Whether you’re working on a simple script or a complex application, knowing how to retrieve the current date can come in handy for various purposes, such as logging events, timestamping data, or simply displaying the date to users.
In this article, we will explore several methods to get the current date in Python, along with clear examples and explanations. By the end of this guide, you will have a solid understanding of how to use Python’s built-in libraries to fetch the current date effortlessly.
Method 1: Using the datetime Module
One of the most straightforward ways to get the current date in Python is by using the datetime
module. This module provides a variety of functions and classes to manipulate dates and times. Here’s how you can use it:
from datetime import datetime
current_date = datetime.now().date()
print(current_date)
Output:
2023-10-03
The datetime
module is part of Python’s standard library, meaning you don’t need to install anything extra to use it. In the code example above, we first import the datetime
class from the datetime
module. We then call datetime.now()
to get the current date and time. By chaining the .date()
method, we extract just the date part, discarding the time. The result is printed in the format YYYY-MM-DD, which is a common date format used in many applications. This method is simple and effective, making it a popular choice among developers.
Method 2: Using the date Class
Another approach to get the current date is by directly using the date
class from the datetime
module. This method is slightly more straightforward if you only need the date without the time component.
from datetime import date
current_date = date.today()
print(current_date)
Output:
2023-10-03
In this example, we import the date
class from the datetime
module. The date.today()
method retrieves the current local date directly, without needing to deal with time. This method is particularly useful when you only care about the date and not the time. The output format is the same as before, YYYY-MM-DD. This method is concise and efficient, making it a favorite for many developers who want a quick and easy way to get the current date.
Method 3: Using the time Module
While the datetime
module is the most common way to handle dates and times in Python, you can also use the time
module to get the current date. This method is less common but still effective.
import time
current_time = time.localtime()
current_date = time.strftime("%Y-%m-%d", current_time)
print(current_date)
Output:
2023-10-03
In this code snippet, we first import the time
module. The time.localtime()
function retrieves the current local time as a time struct. We then use time.strftime()
to format this time struct into a string that represents the date in the YYYY-MM-DD format. This method provides a bit more flexibility in terms of formatting, as you can customize the output string to suit your needs. However, it’s generally more complex than using the datetime
module, so it’s usually recommended for situations where you need specific formatting options.
Method 4: Using the arrow Library
If you’re looking for a more powerful and user-friendly way to handle dates and times, consider using the arrow
library. This third-party library offers a more intuitive approach to date and time manipulation.
import arrow
current_date = arrow.now().format('YYYY-MM-DD')
print(current_date)
Output:
2023-10-03
To use the arrow
library, you will first need to install it using pip:
pip install arrow
Once installed, you can use it as shown in the code snippet. The arrow.now()
function retrieves the current date and time, and the format()
method allows you to specify the output format. In this case, we are formatting it to display only the date in the YYYY-MM-DD format. The arrow
library is particularly useful for more complex date manipulations, such as timezone conversions or relative date calculations, making it a great choice for developers who need more than just basic date functionality.
Conclusion
In this article, we explored various methods to get the current date in Python, including using the built-in datetime
and time
modules, as well as the third-party arrow
library. Each method has its own advantages, depending on your specific needs. Whether you prefer the simplicity of datetime
, the directness of date
, the flexibility of time
, or the power of arrow
, Python provides multiple options to retrieve the current date effortlessly. By mastering these techniques, you can enhance your Python programming skills and effectively manage date and time in your projects.
FAQ
-
How do I get the current date and time in Python?
You can use thedatetime.now()
method from thedatetime
module to get both the current date and time. -
What format does the date output in Python?
By default, the date is formatted as YYYY-MM-DD when using thedate
class or thedatetime
module. -
Can I use Python to get the current date in a different format?
Yes, you can use thestrftime()
method to customize the date format according to your needs. -
Is the arrow library necessary for getting the current date?
No, the arrow library is not necessary, but it offers more advanced features and a more user-friendly interface for date manipulation. -
How do I install the arrow library?
You can install the arrow library using pip with the commandpip install arrow
.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn