How to Get Function Signature
- Method 1: Using the inspect Module
- Method 2: Using the doc Attribute
- Method 3: Using Help Function
- Conclusion
- FAQ
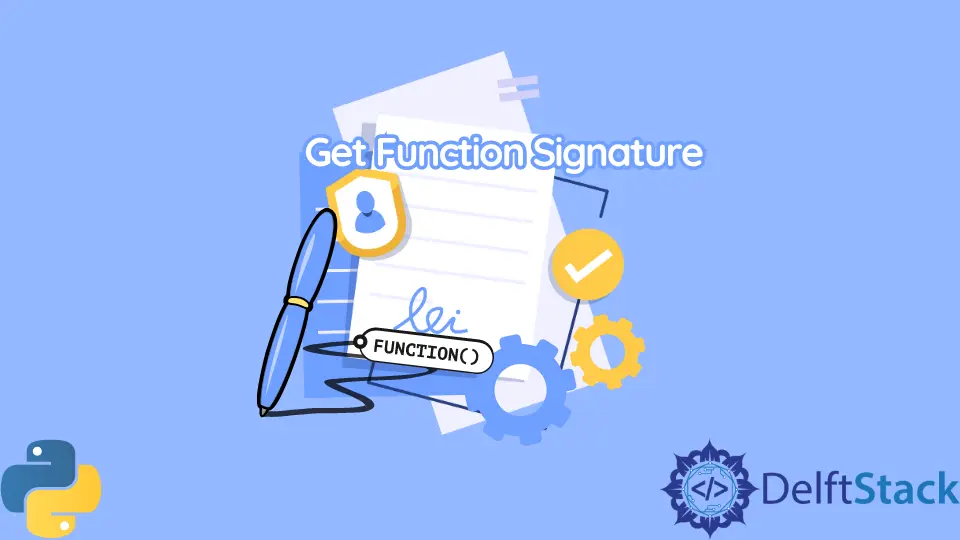
Understanding how to get function signatures in Python can significantly enhance your programming experience. Whether you are debugging code, developing new features, or simply trying to understand a library, knowing how to retrieve function signatures helps you grasp what arguments a function expects, its return type, and much more.
In this tutorial, we will explore various methods to achieve this, providing clear examples and explanations that will empower you to navigate Python’s function signatures with confidence. So, let’s dive in and discover how to extract this valuable information effortlessly!
Method 1: Using the inspect Module
One of the most straightforward ways to get function signatures in Python is by using the built-in inspect
module. This module provides several useful functions to help you retrieve information about live objects, including functions. By utilizing the signature
function from the inspect
module, you can easily access the signature of any callable object.
Here’s a simple example to demonstrate how to use the inspect
module:
import inspect
def example_function(arg1, arg2, kwarg1='default', *args, **kwargs):
pass
sig = inspect.signature(example_function)
print(sig)
Output:
(arg1, arg2, kwarg1='default', *args, **kwargs)
In this code snippet, we first import the inspect
module. We then define a function called example_function
that takes two positional arguments, one keyword argument with a default value, and allows for additional positional and keyword arguments. By calling inspect.signature(example_function)
, we retrieve the function’s signature and print it out.
The output shows the full signature, including both positional and keyword arguments. This method is particularly useful because it provides a clear and concise view of what the function accepts. You can use this approach for any function, making it a versatile tool for developers at all levels.
Method 2: Using the doc Attribute
Another way to get information about a function’s parameters is by accessing its __doc__
attribute. This attribute contains the docstring of the function, which often includes details about the parameters and return types. While this method relies on the function being well-documented, it can still be a quick way to gather information.
Let’s see how this works in practice:
def example_function(arg1, arg2, kwarg1='default'):
"""
This function takes two required arguments and one optional keyword argument.
Parameters:
arg1: The first argument.
arg2: The second argument.
kwarg1: An optional keyword argument with a default value.
Returns:
None
"""
pass
print(example_function.__doc__)
Output:
This function takes two required arguments and one optional keyword argument.
Parameters:
arg1: The first argument.
arg2: The second argument.
kwarg1: An optional keyword argument with a default value.
Returns:
None
In this example, we define the same example_function
, but this time, we include a detailed docstring that describes the parameters and return type. When we print example_function.__doc__
, we get the entire docstring, which provides a wealth of information about how to use the function.
While this method is dependent on the quality of documentation, it can be a quick reference for understanding what a function does and how to use it. It’s particularly useful when working with third-party libraries where you might not have access to the source code.
Method 3: Using Help Function
The built-in help()
function is another excellent way to retrieve function signatures and documentation in Python. This function displays the docstring along with the function signature, providing a clear view of how the function should be used. It’s a great tool for both beginners and experienced developers who need quick access to function details.
Here’s how you can use the help()
function:
def example_function(arg1, arg2, kwarg1='default'):
"""
This function takes two required arguments and one optional keyword argument.
"""
pass
help(example_function)
Output:
Help on function example_function in module __main__:
example_function(arg1, arg2, kwarg1='default')
This function takes two required arguments and one optional keyword argument.
When we call help(example_function)
, Python provides an informative output that includes the function signature and the docstring. This method is particularly useful because it not only gives you the signature but also provides context about what the function does.
The help()
function is a powerful feature in Python that can be used for any object, not just functions. It’s an essential tool for exploring libraries and understanding how to utilize various functions effectively.
Conclusion
Getting function signatures in Python is a crucial skill for any developer. Whether you choose to use the inspect
module, access the __doc__
attribute, or employ the help()
function, each method provides valuable insights into how functions operate. By mastering these techniques, you can write better code, debug more efficiently, and enhance your overall programming experience. So, the next time you encounter a function, remember these methods, and you’ll be well-equipped to understand its signature and usage.
FAQ
-
What is a function signature in Python?
A function signature in Python refers to the declaration of a function, which includes its name, parameters, and return type. -
Why is it important to know a function’s signature?
Knowing a function’s signature helps you understand what arguments it expects, which can prevent errors and improve code readability. -
Can I get the signature of built-in functions in Python?
Yes, you can use theinspect
module or thehelp()
function to retrieve signatures for built-in functions. -
What if a function does not have a docstring?
If a function lacks a docstring, you can still use theinspect
module to get its signature, but you won’t have additional context about its purpose. -
Are there any tools that can help with function signatures in Python?
Yes, various IDEs and code editors provide features that show function signatures and documentation as you type, which can be very helpful.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn