Naming Convention for Functions, Classes, Constants, and Variables in Python
- Naming Convention for Functions in Python
- Naming Convention for Classes in Python
- Naming Convention for Constants in Python
- Naming Convention for Variables in Python
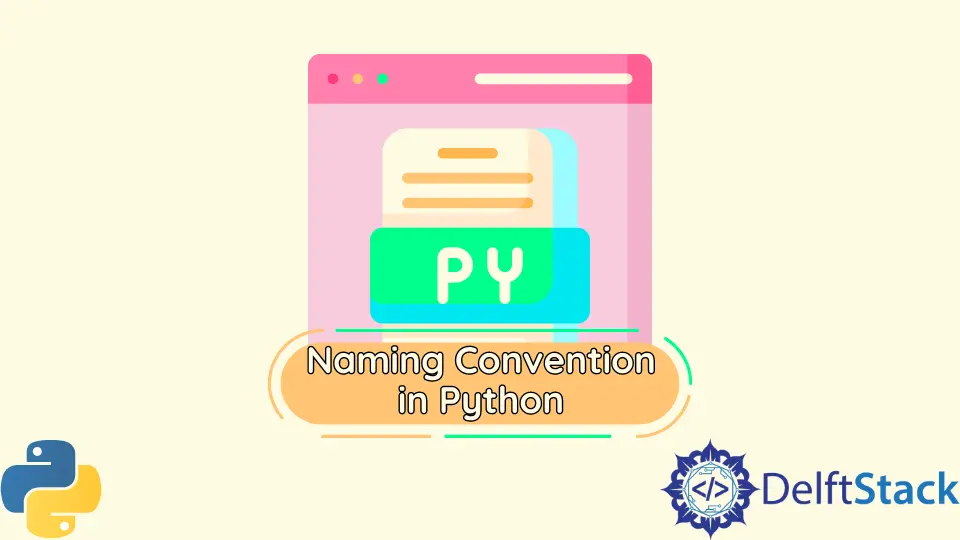
PEP 8 or Python Enhancement Proposal 8 is a guide for guidelines and best practices to write Python code. These guidelines aim to improve the readability, understanding, and consistency of Python codebases. Since PEP 8 has a standard and is majorly used in the industry and by professionals, it is better to stick to it as a beginner because almost all the Python codebases use it, and using the same for new additions promotes backward compatibility with the style of coding.
PEP 8 also talks about the naming convention used to name variables, functions, constants, and classes in Python. This article will talk about those conventions along with some relevant examples.
Naming Convention for Functions in Python
PEP 8 recommends to use lowercase words seperated by underscores to name functions. For example, hello_world
, computer_science
, send_mail_to_user
, get_updates_from_user
, delete_all_users
, etc.
def hello_world():
pass
def computer_science():
pass
def send_mail_to_user():
pass
def get_updates_from_user():
pass
def delete_all_users():
pass
Naming Convention for Classes in Python
PEP 8 recommends using Upper Camel Case or Pascal Case to name classes. For example, Person
, HelloWorld
, Human
, PythonIsFun
, MyCustomClass
, etc.
class Person:
pass
class HelloWorld:
pass
class Human:
pass
class PythonIsFun:
pass
class MyCustomClass:
pass
Naming Convention for Constants in Python
PEP 8 recommends using uppercase words separated by underscores to name constants. For example, HELLO_WORLD
, COMPUTER_SCIENCE
, NUMBER_OF_USERS
, EMAIL_LIMIT
, EMAIL_USERNAME
, etc.
HELLO_WORLD = "A string"
COMPUTER_SCIENCE = "A subject"
NUMBER_OF_USERS = 450
EMAIL_LIMIT = 100
EMAIL_USERNAME = "vaibhav"
Naming Convention for Variables in Python
PEP 8 recommends to use lowercase words seperated by underscores to name variables. For example, hello_world
, computer_science
, number_of_users
, email_limit
, email_username
, etc.
hello_world = "A string"
computer_science = "A subject"
number_of_users = 450
email_limit = 100
email_username = "vaibhav"