How to Start a for Loop at 1 in Python
-
Use a Simple User-Defined Function to Start the
for
Loop at an Index 1 in Python -
Use Nested
for
Loop to Start thefor
Loop at an Index 1 in Python -
Use
n+1
in Place ofn
in therange()
Function to Start thefor
Loop at an Index 1 in Python
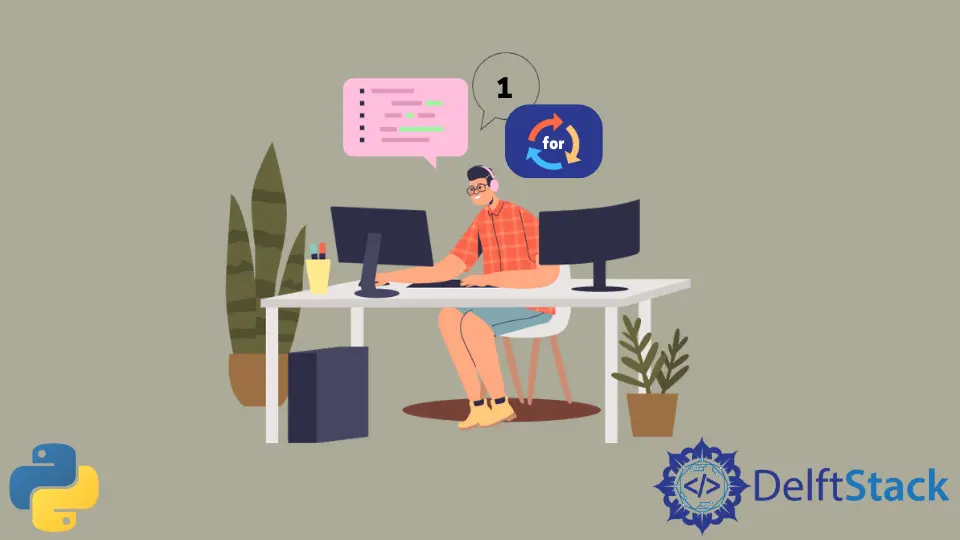
Similar to any other programming language, the starting index of the for
loop is 0
by default. However, the range of the iteration statement can be manipulated, and the starting index of the loop can be changed to 1
.
This tutorial will introduce how to start the for
loop at an index 1 in Python.
Use a Simple User-Defined Function to Start the for
Loop at an Index 1 in Python
We can easily create a function on our own to implement this method. The created function can then be utilized in the for
loop instead of the range()
function.
The following code uses a simple user-defined function to start the for
loop at an index 1 in Python.
def nums(first_number, last_number, step=1):
return range(first_number, last_number + 1, step)
for i in nums(1, 5):
print(i)
The above code provides the following output:
1
2
3
4
5
Use Nested for
Loop to Start the for
Loop at an Index 1 in Python
Another way to start the for
loop at an index 1 in Python is to use the for
loop twice. This is utilized along with the range()
function.
The following code uses the nested for
loop to start the for
loop at an index 1 in Python.
for x in (n + 1 for n in range(5)):
print(x)
The above code provides the following output:
1
2
3
4
5
Use n+1
in Place of n
in the range()
Function to Start the for
Loop at an Index 1 in Python
This method can be implemented by using the start
value as 1
and the stop value as n+1
instead of default values 0
and n
, respectively.
The following code uses n+1
in place of n
in the range()
function to start the for
loop at an index 1 in Python.
n = 5
for x in range(1, n + 1):
print(x)
The above code provides the following output:
1
2
3
4
5
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn