How to Convert Float to String in Python
-
Method 1: Using the
str()
Function -
Method 2: Using the
format()
Method - Method 3: Using F-Strings (Python 3.6+)
- Conclusion
- FAQ
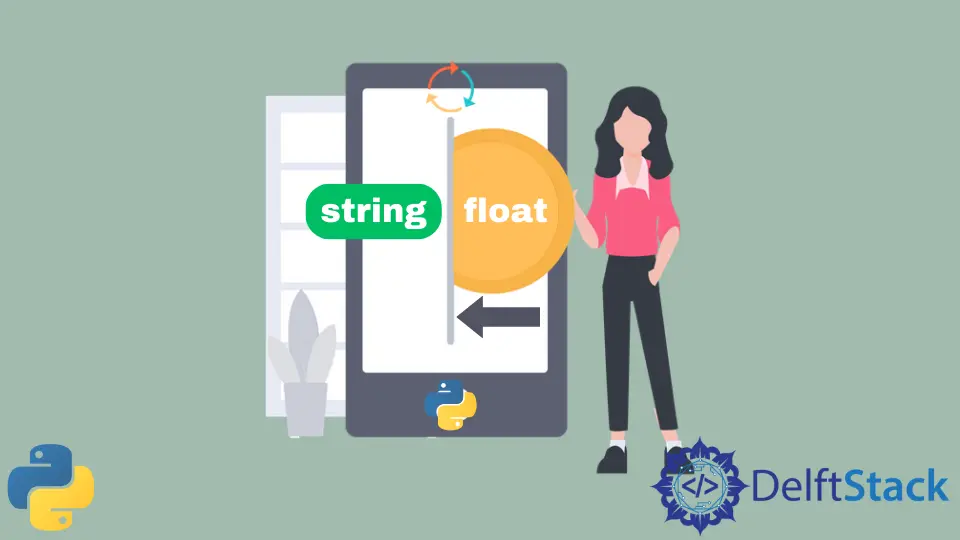
Converting a float to a string in Python is a common task that every programmer encounters. Whether you’re working with numerical data, formatting output, or preparing data for storage, knowing how to perform this conversion is essential. Python offers various methods to achieve this, each with its unique advantages.
In this tutorial, we’ll explore three primary methods: using the built-in str()
function, the format()
method, and f-strings. By the end of this article, you’ll have a solid understanding of how to convert floats to strings, enabling you to apply these techniques in your own projects effectively.
Method 1: Using the str()
Function
The simplest way to convert a float to a string in Python is by using the built-in str()
function. This method is straightforward and easy to implement, making it ideal for beginners. The str()
function takes a float as an argument and returns its string representation.
Here’s a basic example:
# Example of converting a float to string using str()
float_number = 12.34
string_number = str(float_number)
print(string_number)
Output:
12.34
The str()
function works seamlessly for any float value, converting it to a string without requiring additional formatting. This method is particularly useful when you need a quick conversion without concerns about decimal places or formatting. However, it does not provide options for controlling the number of decimal points or padding.
If you’re looking for a simple and efficient way to convert floats to strings, the str()
function is your best bet. It is widely used in various applications where basic string representation is sufficient. However, if you require more control over the formatting, you may want to explore other methods.
Method 2: Using the format()
Method
Another effective way to convert a float to a string in Python is by using the format()
method. This method allows for greater flexibility and control over how the float is represented as a string. With format()
, you can specify the number of decimal places, add padding, or even include thousands separators.
Here’s an example of how to use the format()
method:
# Example of converting a float to string using format()
float_number = 12.34567
string_number = "{:.2f}".format(float_number)
print(string_number)
Output:
12.35
In this example, the "{:.2f}"
format specifier indicates that we want to round the float to two decimal places. The format()
method is versatile, allowing for various formatting options. You can adjust the number of decimal places by changing the number in the format string.
Moreover, you can also add padding or specify alignment using additional formatting codes. For instance, if you want to include thousands separators or control the total width of the output string, the format()
method can accommodate those needs as well.
Using the format()
method is ideal when you want to ensure that your float is displayed in a specific format, making it a powerful tool for data presentation and reporting.
Method 3: Using F-Strings (Python 3.6+)
F-strings, introduced in Python 3.6, provide a modern and efficient way to format strings. They allow you to embed expressions within string literals, making them a convenient choice for converting floats to strings. F-strings are not only more readable but also often faster than the format()
method.
Here’s how you can use f-strings to convert a float to a string:
# Example of converting a float to string using f-strings
float_number = 12.34567
string_number = f"{float_number:.3f}"
print(string_number)
Output:
12.346
In this example, the f-string f"{float_number:.3f}"
indicates that we want to format the float_number
to three decimal places. The syntax is clean and intuitive, making it easy to read and understand.
F-strings also offer the ability to evaluate expressions and include variables directly within the string. This feature simplifies code and enhances readability, especially when dealing with multiple variables.
If you’re using Python 3.6 or newer, f-strings are highly recommended for their efficiency and ease of use. They are particularly useful in scenarios where you need to format strings dynamically, such as in logging or user-facing messages.
Conclusion
In this tutorial, we explored three effective methods to convert a float to a string in Python: using the str()
function, the format()
method, and f-strings. Each method has its strengths and is suited for different scenarios. Whether you need a simple conversion or require specific formatting control, Python provides versatile options to meet your needs. By mastering these techniques, you’ll enhance your programming skills and be better equipped for various tasks in your Python projects.
FAQ
-
What is the simplest way to convert a float to a string in Python?
The simplest way is to use the built-instr()
function. -
Can I control the number of decimal places when converting a float to a string?
Yes, you can use theformat()
method or f-strings to specify the number of decimal places. -
Are f-strings available in all Python versions?
No, f-strings were introduced in Python 3.6. If you’re using an older version, consider using theformat()
method. -
What is the output of the str() function when converting a float?
Thestr()
function returns the string representation of the float, preserving its decimal format. -
Can I include variables in my string conversion using f-strings?
Yes, f-strings allow you to embed variables directly within the string for dynamic formatting.
Related Article - Python Float
- How to Find Maximum Float Value in Python
- How to Fix Float Object Is Not Callable in Python
- How to Convert a String to a Float Value in Python
- How to Check if a String Is a Number in Python
- How to Convert String to Decimal in Python
- How to Convert List to Float in Python