How to Extract Numbers From a String in Python
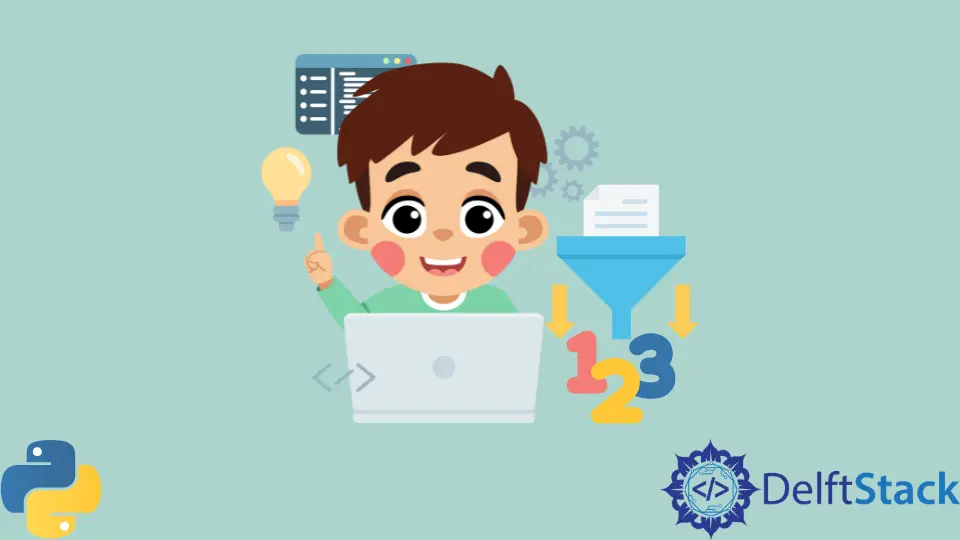
This tutorial explains how to get numbers from a string in Python. It also lists some example codes to further clarify the concept using different approaches.
Use List Comprehension to Extract Numbers From a String
Numbers from a string can be obtained by simple list comprehension. split()
method is used to convert string to a list of characters and isdigit()
method is used to check if a digit is found through the iteration.
A basis code example is given as:
temp_string = "Hi my age is 32 years and 250 days12"
print(temp_string)
numbers = [int(temp) for temp in temp_string.split() if temp.isdigit()]
print(numbers)
Output:
Hi my age is 32 years and 250 days12
[32, 250]
However, this code does not identify numbers that come with alphabets.
Use the re
Module to Extract Numbers From a String
The re
module of Python also provides functions that can search through the string and extract results. The re
module provides with the findall()
method that returns a list of all the matches. An example code is given below:
import re
temp_string = "Hi my age is 32 years and 250.5 days12"
print(temp_string)
print([float(s) for s in re.findall(r"-?\d+\.?\d*", temp_string)])
Output:
Hi my age is 32 years and 250.5 days12
[32.0, 250.5, 12.0]
The RegEx
solution works for negative and positive numbers and overcomes the problem encountered in the List Comprehension approach.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn