How to Fix Error - EOL While Scanning String Literal in Python
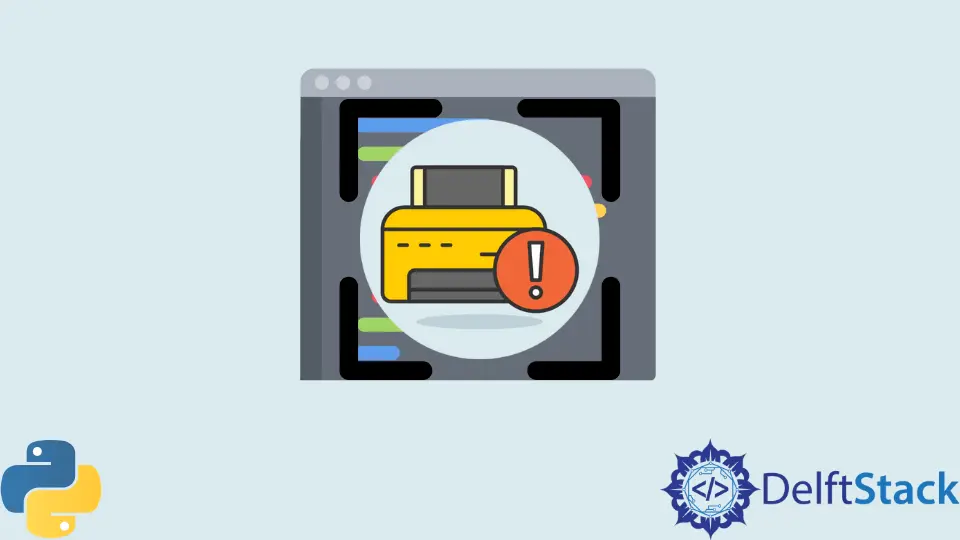
This tutorial will discuss the syntax error EOL while scanning string literal in Python.
Raw Strings in Python
Raw strings are used for specifying regular expressions in Python. Before the starting quotation mark, a raw string is declared by an r
or R
. A raw string does not need any escape characters and treats the backslash as a literal string by convention. The following code snippet demonstrates the working of a raw string.
print(r"\t\\")
Output:
\t\\
Now, lets see how this would look like in a normal string.
print("\t\\")
Output:
\
The difference is crystal clear. When we use a raw string, the python interpreter considers \t\\
as \t\\
, but when we use a regular string, the Python interpreter considers the \t
as a tab and the following \
as an escape character for the last \
.
EOL While Scanning String Literal in Python
The only limitation with these raw strings is that we can only end them with an even number of backslashes. If a raw string ends with an odd number of backslashes, the Python interpreter shows the syntax error EOL while scanning string literal
. This is because even in raw strings, the quotation mark can be escaped by a backslash. With an odd number of backslashes in the end, the interpreter thinks that the last backslash is used to escape the closing quotation mark and keeps scanning for the end of the string. This phenomenon has been demonstrated in the coding example below.
print(r'\t\\\')
Output:
File "<ipython-input-1-d2ab522bcdab>", line 1
print(r'\t\\\')
^
SyntaxError: EOL while scanning string literal
We demonstrated the EOL while scanning string literal
error while writing raw strings in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn