How to Encode UTF8 in Python
- Understanding UTF-8 Encoding
- Encoding Strings Using the encode() Method
- Handling Errors During Encoding
- Decoding UTF-8 Back to String
- Conclusion
- FAQ
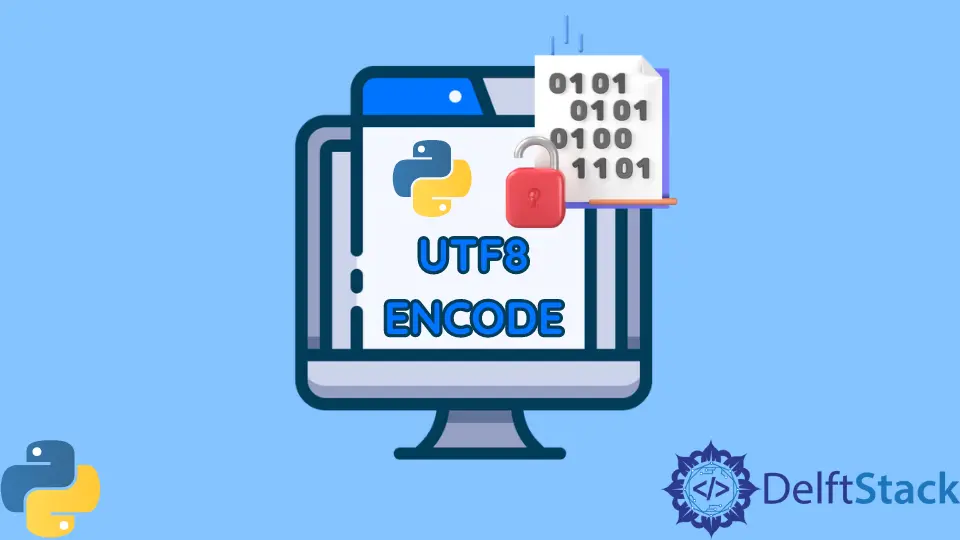
When working with strings in Python, especially those that include special characters, encoding becomes a crucial topic. UTF-8 is one of the most widely used encoding formats because it can represent any character in the Unicode standard.
This tutorial will walk you through the process of encoding strings in UTF-8 using Python’s built-in encode()
method. Whether you’re a beginner or an experienced developer, understanding how to handle UTF-8 encoding will enhance your ability to work with diverse text data. By the end of this article, you’ll have a solid grasp of how to effectively encode strings in UTF-8, ensuring that your applications can handle internationalization and special characters with ease.
Understanding UTF-8 Encoding
Before diving into the practical aspects of encoding strings in UTF-8, it’s essential to understand what UTF-8 is. UTF-8 is a variable-width character encoding that can represent every character in the Unicode character set. It uses one to four bytes for each character, making it efficient for encoding standard ASCII characters while still being able to represent more complex characters from other languages. This flexibility is what makes UTF-8 so popular in web development and data processing.
When you encode a string in UTF-8, you convert it from a Python string (which is a sequence of Unicode characters) to a bytes object. This conversion is crucial when you need to store or transmit text data, as bytes are more universally compatible across different systems and platforms.
Encoding Strings Using the encode() Method
The primary way to encode a string in UTF-8 in Python is by using the encode()
method. This method is available on string objects and takes the desired encoding format as an argument. By default, if no encoding is specified, it uses UTF-8.
Here’s how you can use the encode()
method:
text = "Hello, World! こんにちは"
encoded_text = text.encode('utf-8')
Output:
b'Hello, World! \xe3\x81\x93\xe3\x82\x93\xe3\x81\xaa\xe3\x81\xa1'
In this example, we start with a string containing both English and Japanese characters. When we call encode('utf-8')
, Python converts the string into a bytes object, which is a sequence of bytes representing the original string in UTF-8 format. The output shows the byte representation, where each character is encoded accordingly. This process is seamless and allows you to handle various character sets without any hassle.
Handling Errors During Encoding
While encoding strings, you may encounter characters that cannot be encoded in the specified format. Python’s encode()
method allows you to handle such situations gracefully by providing an optional errors
parameter. This parameter can take values like ‘ignore’, ‘replace’, or ‘strict’, which dictate how Python should respond to encoding errors.
Here’s an example:
text = "Hello, World! こんにちは"
encoded_text = text.encode('ascii', errors='ignore')
Output:
b'Hello, World! '
In this case, we attempt to encode the string using ASCII, which cannot handle the Japanese characters. By setting errors='ignore'
, Python ignores the characters that can’t be encoded, resulting in a bytes object that only includes the ASCII-compatible characters. This feature is particularly useful when you want to ensure your application remains functional, even when faced with unexpected characters.
Decoding UTF-8 Back to String
After encoding a string in UTF-8, you might need to decode it back to a regular string. This is done using the decode()
method, which is called on the bytes object. Decoding is just as straightforward as encoding, and it’s essential when you need to manipulate or display the original string.
Here’s how to decode a UTF-8 encoded string:
encoded_text = b'Hello, World! \xe3\x81\x93\xe3\x82\x93\xe3\x81\xaa\xe3\x81\xa1'
decoded_text = encoded_text.decode('utf-8')
Output:
Hello, World! こんにちは
In this example, we start with a bytes object that represents our original string in UTF-8. By calling decode('utf-8')
, we convert the bytes back into a Python string. The output confirms that we have successfully returned to our original text, including the special characters. This round-trip process of encoding and decoding is fundamental when working with text data in various formats.
Conclusion
Encoding strings in UTF-8 using Python is a straightforward yet essential skill for any developer. By leveraging the encode()
and decode()
methods, you can easily handle a wide range of characters from different languages and scripts. Whether you’re developing web applications, processing text files, or working with databases, understanding UTF-8 encoding will enhance your ability to manage text data effectively. With this knowledge, you can ensure that your applications remain robust and user-friendly, accommodating diverse user inputs without issues.
FAQ
-
What is UTF-8 encoding?
UTF-8 is a variable-width character encoding that can represent every character in the Unicode character set, using one to four bytes per character. -
How do I encode a string in UTF-8 in Python?
You can encode a string in UTF-8 by using theencode()
method, like this:encoded_text = your_string.encode('utf-8')
. -
What happens if I try to encode a character that isn’t supported?
If you attempt to encode unsupported characters, you can specify theerrors
parameter in theencode()
method to handle the situation, such as ignoring or replacing the characters.
-
Can I decode UTF-8 encoded bytes back to a string?
Yes, you can decode UTF-8 encoded bytes back to a string using thedecode()
method, like this:decoded_text = encoded_bytes.decode('utf-8')
. -
Why is UTF-8 important in programming?
UTF-8 is important because it allows for the representation of a wide range of characters from different languages, making it essential for internationalization and working with diverse text data.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn