Python Egg
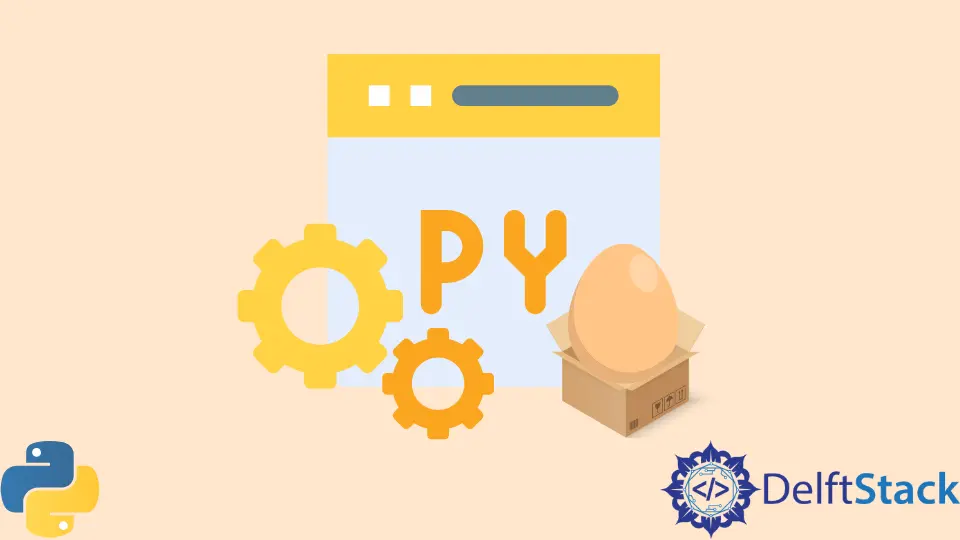
Python Egg is a packaging format used in the past but is now replaced by a newer format called Python Wheel. The Egg distribution format was introduced in 2004 by setuptools
, whereas the Wheel format was launched by PEP 427 in 2012.
.egg
files are like .zip
files and are completely cross-platform. They are built using the setuptools
package.
This tutorial will teach you to create a .egg
file and install it using easy_install
in Python.
Create a .egg
File for Your Package in Python
Let’s see how you can create the egg file for the package in Python. This tutorial will use the test
package, which contains these files:
hello.py
__init__.py
First, create a new directory and put your Python package folder inside it. Then make a new file, setup.py
, and add the content below.
from setuptools import setup, find_packages
setup(name="test", version="0.1", packages=find_packages())
You have to replace the test
with your Python package name. The find_packages
function finds the package in the current directory.
Next, run the following command to create a .egg
file.
python setup.py bdist_egg
Output:
It will create three new folders in the current directory: build
, dist
, and test.egg-info
.
C:\USERS\RHNTM\EGG
├───build
│ ├───bdist.win-amd64
│ └───lib
│ └───test
├───dist
├───test
└───test.egg-info
You can find your .egg
file test-0.1-py3.10.egg
inside the dist
folder. 3.10 is the version of Python installed on this computer.
Now, since you have a .egg
file, you can use this command to install the package you have created.
python2 -m easy_install .\test-0.1-py3.10.egg
Output:
Processing test-0.1-py3.10.egg
Copying test-0.1-py3.10.egg to c:\python27\lib\site-packages
Adding test 0.1 to easy-install.pth file
Installed c:\python27\lib\site-packages\test-0.1-py3.10.egg
If you change the .egg
file extension to .zip
, you can find two folders: test
and EGG-INFO
inside the .zip
file.
Now you should have understood the .egg
file and how to create it for your Python package. You have also learned to use easy_install
to install the package in Python.
The .egg
is an obsolete format and no longer in use; wheels
and pip install
have replaced it. For more information, read this article.