do...while Loop in Python
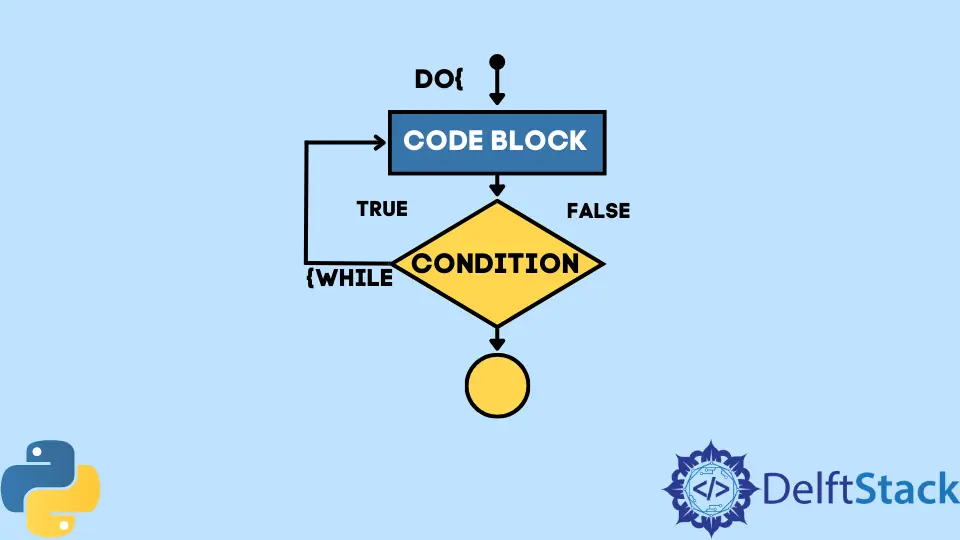
The loop is a very common and useful feature in almost all programming languages. We have entry-controlled loops and exit-controlled loops. The do-while
loop is an example of the latter. This means that unlike the while
loop, which is an entry-controlled loop, the do-while
loop tests the condition at the end of the iteration, and the loop is executed at least one time, regardless of the condition.
The do-while
loop is not present in Python by default, but we can generate some code using the while loop to make something that can act as a do-while
loop.
In the following code, we try to emulate a do-while
loop which will print values from one to ten.
x = 0
while True:
print(x)
x = x + 1
if x > 10:
break
Output:
0
1
2
3
4
5
6
7
8
9
10
In the above method, we put the condition as True
so that the while
loop will execute at least one time, and later in the loop, we test the condition to stop the loop. The break
statement here is used to break out from the loop as soon as the desired condition is met.
We can avoid using the break
statement and create something as shown below to emulate the do-while
loop.
x = 0
condition = True
while condition == True:
print(x)
x = x + 1
if x > 10:
condition = False
Output:
0
1
2
3
4
5
6
7
8
9
10
Both the above methods are the simulations of the do-while
loop. It allows us to create something from the while
loop that can achieve the desired effect of the do-while
loop.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn