How to Use a DLL File From Python
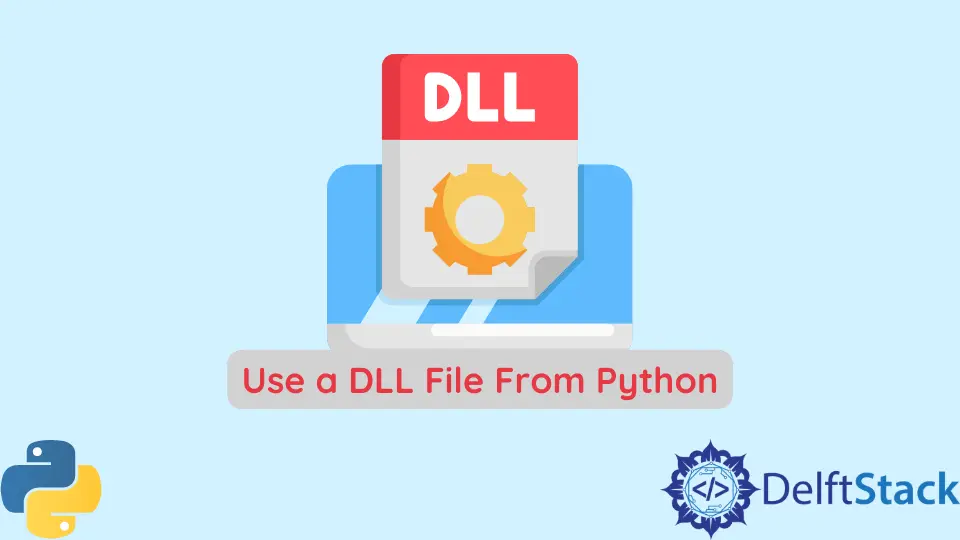
A DLL stands for Dynamic Link Library. A DLL file contains scripts that can be called by multiple programs to perform certain operations.
Most DLL files have .dll
extensions. They cannot be executed directly but can be used from other applications to call their functions.
This tutorial will teach you to use a DLL file from a Python program.
Use ctypes
Library to Use a DLL File From Python
ctypes
is a foreign function library that provides C-compatible data types in Python. It is also used for calling functions in DLLs.
Let’s see an example to call functions of .dll
using Python script. We will use a user32.dll
file for this tutorial in the C:\Windows\System32
directory.
First, you must import the ctypes
library and load a DLL file.
import ctypes
info = ctypes.WinDLL("C:\\Windows\\System32\\user32.dll")
After that, you can call methods in the DLL file. The following command prints the number of buttons on an installed mouse.
print(info.GetSystemMetrics(43))
Output:
7
The GetSystemMetrics
function in user32.dll
helps to retrieve the system metrics or configuration settings. It takes only one integer parameter.
You can find other valid parameters and their return values in this article. Also, there are several functions in user32.dll
that you can read from the Microsoft docs page.
For example, you can call the GetKeyboardType
function to view information about the keyboard.
print(info.GetKeyboardType(0))
Output:
4
The return value is 4
, meaning the current keyboard type is Enhanced 101- or 102-key.
Now you should know how to use a DLL file and call its functions in Python. We hope you like this tutorial.