Python Divisible
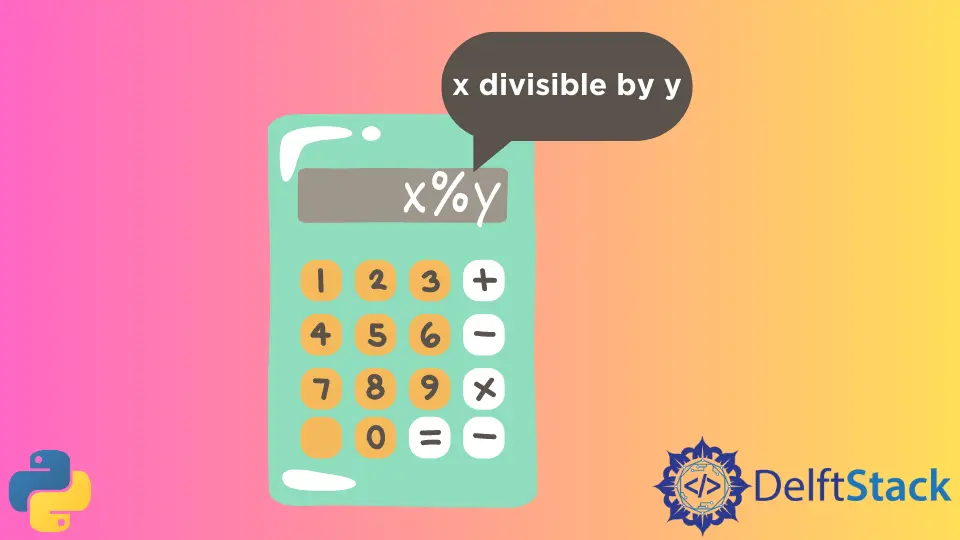
This tutorial will discuss how to check whether a number is completely divisible by another number in Python.
Check Whether a Number Is Divisible by Another Number With the %
Operator in Python
Let x
and y
be two numbers. The number x
is completely divisible by y
if there is no remainder after x/y
. To check this, we have a built-in operator %
called the modulus operator in Python. The modulus operator carries out the division and returns the remainder of that division. For example, if x = 3
and y = 2
, then x%y
will divide 3
by 2
and give us 1
as a remainder. The divisible()
function in the following code snippet shows us how we can check whether one number is completely divisible by another number with the %
operator.
def divisible(x, y):
if x % y == 0:
print("Divisible")
else:
print("Not Divisible")
divisible(20, 10)
Output:
Divisible
The divisible()
function takes two parameters x
and y
. If the value of x%y
is equal to 0
, that means x
is completely divisible by y
, and Divisible
is displayed on the console. If the value of x%y
is not equal to 0
, that means x
isn’t completely divisible by y
, and Not Divisible
is displayed on the console.
In the current scenario, our x
is 20
and y
is 10
. Because 20%10
gives us 0
, the output shows Divisible
in the console.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn