How to Display an Image in Python
- Using Matplotlib to Display Images
- Using OpenCV to Display Images
- Using PIL (Pillow) to Display Images
- Conclusion
- FAQ
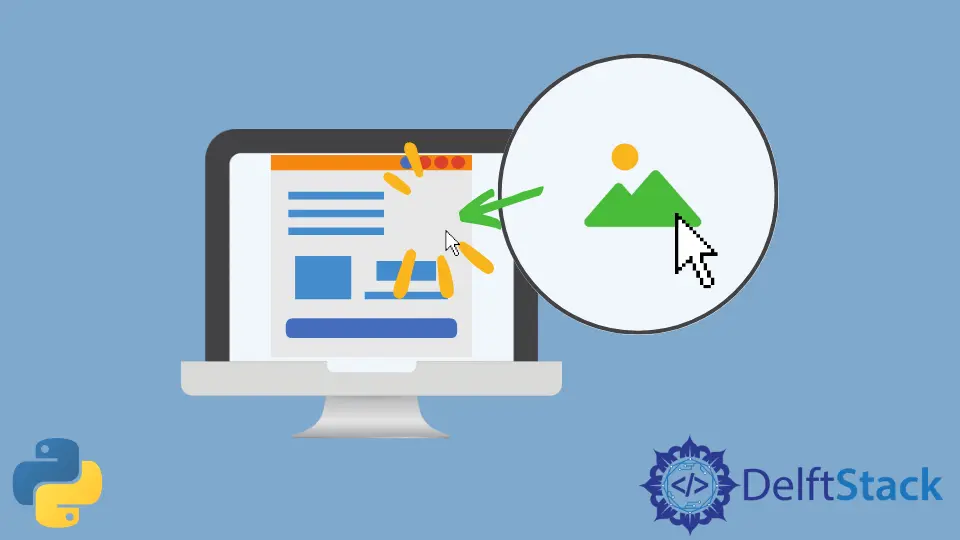
Displaying images in Python is a fundamental skill for anyone interested in computer vision, data visualization, or simply learning more about the capabilities of Python. Whether you’re working on a project that requires image processing or just want to visualize some data, knowing how to display images can significantly enhance your workflow.
In this tutorial, we will explore several methods to display images using popular libraries like Matplotlib and OpenCV. Each method will come with a clear example, ensuring you can easily follow along and implement these techniques in your projects. Let’s dive in!
Using Matplotlib to Display Images
Matplotlib is one of the most widely used libraries for data visualization in Python. It provides a simple way to display images using the imshow()
function. First, you need to make sure you have Matplotlib installed, which you can do via pip if you haven’t already.
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
img = mpimg.imread('path_to_your_image.jpg')
plt.imshow(img)
plt.axis('off')
plt.show()
In this code snippet, we first import the necessary modules from Matplotlib. The imread()
function reads the image file, and imshow()
displays it. The axis('off')
command hides the axes, providing a cleaner view of the image. Finally, show()
displays the image in a new window. This method is particularly useful for quick visualizations, especially in Jupyter notebooks or during exploratory data analysis.
Using OpenCV to Display Images
OpenCV is another powerful library that specializes in computer vision tasks. It has its own method for displaying images, which can handle a variety of image formats and is optimized for performance. Here’s how you can use OpenCV to display an image.
import cv2
img = cv2.imread('path_to_your_image.jpg')
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
In this example, we import the OpenCV library and use the imread()
function to load the image. The imshow()
function is then called to display the image in a window titled ‘Image’. The waitKey(0)
function pauses the program until any key is pressed, ensuring that the window remains open for you to view the image. Finally, destroyAllWindows()
closes the window once you’re done. OpenCV is particularly advantageous for projects involving real-time image processing or video analysis.
Using PIL (Pillow) to Display Images
The Python Imaging Library (PIL), now maintained under the name Pillow, is another excellent option for image manipulation and display. It offers a straightforward way to work with images, and displaying them is no exception. Here’s how you can use Pillow to display an image.
from PIL import Image
img = Image.open('path_to_your_image.jpg')
img.show()
In this code, we import the Image
module from PIL. The open()
function loads the image file, and the show()
method displays it in the default image viewer on your system. This method is very user-friendly, making it an excellent choice for beginners or for quick image checks without the need for complex setups.
Conclusion
In this tutorial, we explored three popular methods to display images in Python: using Matplotlib, OpenCV, and Pillow. Each method has its unique advantages, making them suitable for different scenarios. Whether you’re visualizing data, processing images, or simply viewing an image file, these libraries can enhance your Python experience. So go ahead and experiment with these techniques in your projects!
FAQ
-
What libraries can I use to display images in Python?
You can use libraries like Matplotlib, OpenCV, and Pillow (PIL) to display images in Python. -
Is it necessary to install these libraries?
Yes, you need to install these libraries using pip if they are not included in your Python environment. -
Can I display images in Jupyter notebooks?
Yes, you can easily display images in Jupyter notebooks using Matplotlib. -
What is the difference between OpenCV and Matplotlib for image display?
OpenCV is optimized for real-time image processing, while Matplotlib is more suited for data visualization and quick image checks.
- How can I close the image window opened by OpenCV?
You can close the OpenCV image window by pressing any key after callingwaitKey(0)
.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn