How to Check if Directory Exists using Python
-
Check if Directory Exists Using
path.isdir()
Method ofos
Module in Python -
Check if Directory Exists Using
path.exists()
Method ofos
Module in Python
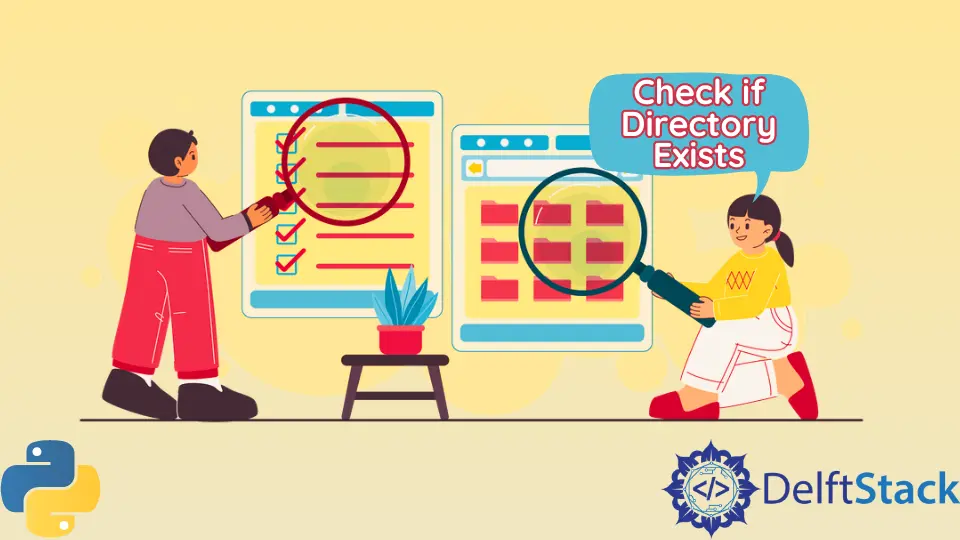
This tutorial will look into various methods in Python to check if a specific directory exists or not. Suppose we have a program that saves a file in a specific directory and if the directory does not exist, it creates it first. For this, we need a method to check if a specific directory exists or not.
Check if Directory Exists Using path.isdir()
Method of os
Module in Python
The path.isdir()
method of the os
module takes a path string as input and returns True
if the path refers to an existing directory and returns False
if the directory does not exist on that path.
Suppose we want to check if the directory myfolder
exists at the path /testfolder/myfolder
, the path.isdir()
method will return True
if the directory myfolder
exists at the path, otherwise it will return False
.
The example code below demonstrates the use of the path.isdir()
method:
import os
os.path.isdir(r"/testfolder/myfolder")
Check if Directory Exists Using path.exists()
Method of os
Module in Python
The path.exists()
method of the os
module in Python takes a path as input and returns True
if the path refers to an existing path and returns False
otherwise. It is different from the path.isdir()
method as it also works for files.
Unlike the path.isdir()
method, the path.exists()
method checks not only the directory but also the file exists. And to check the existence of a directory, we will have to give the path of that directory like /testfolder/myfolder
.
The code example below demonstrates the use of the path.exists()
method for both file and directory:
import os
os.path.exists("Desktop/folder/myfolder")
os.path.exists("Desktop/folder/myfile.txt")
path.exists()
method can not distinguish between a path of a directory or a file, in case there is a file named myfolder
with no extension, in the path Desktop/folder/myfolder
the path.exists()
method will return True
.Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python