Python Dictionary Index
- Understanding Python Dictionaries
- Accessing Elements by Keys
-
Using the
get()
Method - Iterating Through a Dictionary
- Conclusion
- FAQ
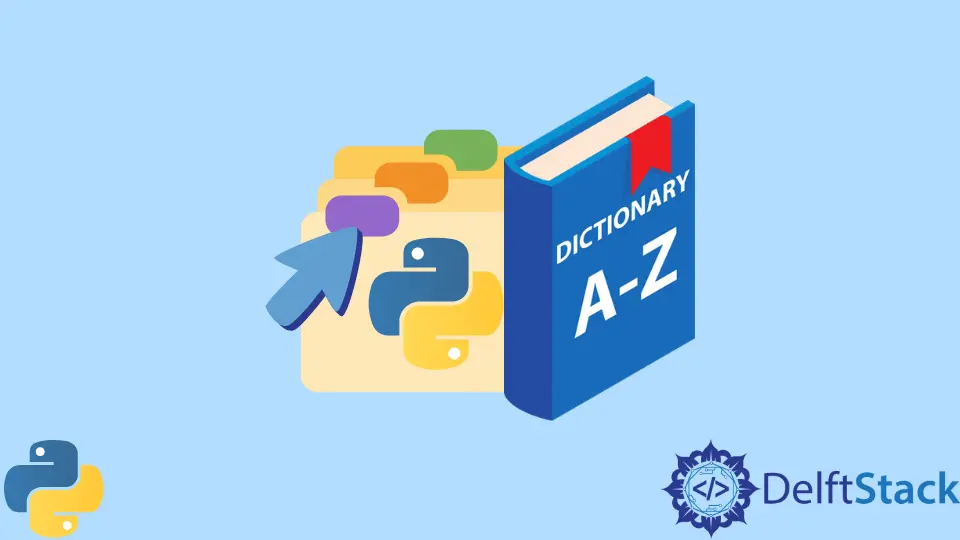
Dictionaries in Python are versatile data structures that allow you to store key-value pairs. They are widely used due to their ability to provide fast access to data. However, a common point of confusion for many beginners is how to index and access elements within a dictionary. Unlike lists, dictionaries do not maintain order in a way that allows for traditional indexing.
This tutorial will guide you through the process of accessing dictionary elements using their keys, which serve as unique identifiers. We’ll explore different methods to efficiently retrieve values, ensuring you have a solid understanding of how to work with Python dictionaries.
Understanding Python Dictionaries
Before diving into indexing, it’s essential to understand the structure of a Python dictionary. A dictionary is defined using curly braces {}
and consists of key-value pairs. Each key is unique, and it maps to a specific value. Here’s a simple example of a dictionary:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York"
}
In this example, “name”, “age”, and “city” are the keys, while “Alice”, 30, and “New York” are the corresponding values. To access a value, you use its key, like so:
print(my_dict["name"])
Output:
Alice
This will retrieve the value associated with the key “name”. Understanding this fundamental concept is crucial for effectively working with dictionaries in Python.
Accessing Elements by Keys
The most straightforward method to access elements in a Python dictionary is by using the keys directly. This method is efficient and clear, making it the go-to approach for most users. Here’s how it works:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York"
}
name = my_dict["name"]
age = my_dict["age"]
city = my_dict["city"]
print(name)
print(age)
print(city)
Output:
Alice
30
New York
In this code, we access each value by referencing its key. The process is straightforward: you simply place the key inside square brackets following the dictionary name. If the key exists, Python returns the corresponding value. However, if you attempt to access a key that doesn’t exist, Python will raise a KeyError
. To avoid this, you can use the get()
method, which allows you to specify a default value to return if the key is not found.
Using the get()
Method
Another effective way to access dictionary elements is by utilizing the get()
method. This method is particularly useful when you’re unsure if a key exists in the dictionary, as it prevents errors and allows for default values. Here’s a closer look at how to use it:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York"
}
name = my_dict.get("name", "Not Found")
age = my_dict.get("age", "Not Found")
country = my_dict.get("country", "Not Found")
print(name)
print(age)
print(country)
Output:
Alice
30
Not Found
In this example, we retrieve the values for “name” and “age” just like before. However, when we try to access “country”, which does not exist in the dictionary, the get()
method returns “Not Found” instead of raising an error. This feature is particularly beneficial in scenarios where data may be incomplete or when working with user-generated input.
Iterating Through a Dictionary
Sometimes, you may want to access all elements in a dictionary without specifying each key. In such cases, you can iterate through the dictionary using a loop. This method is particularly useful for processing or displaying all key-value pairs. Here’s how to do it:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York"
}
for key, value in my_dict.items():
print(f"{key}: {value}")
Output:
name: Alice
age: 30
city: New York
In this code snippet, we use a for
loop combined with the items()
method, which returns a view of the dictionary’s key-value pairs. By unpacking these pairs into key
and value
, we can easily print or manipulate them as needed. This method allows for dynamic processing of dictionary contents, making it a powerful tool for data handling in Python.
Conclusion
Navigating Python dictionaries may seem daunting at first, especially when it comes to indexing and accessing elements. However, by understanding how to use keys effectively and leveraging methods like get()
and iteration, you can efficiently manage and manipulate dictionary data. With practice, you’ll find that dictionaries are not only easy to use but also invaluable in your programming toolkit. Whether you’re building applications or analyzing data, mastering dictionaries will enhance your Python skills significantly.
FAQ
-
What is a Python dictionary?
A Python dictionary is a built-in data structure that stores key-value pairs, allowing for fast data retrieval using unique keys. -
How do I check if a key exists in a dictionary?
You can check if a key exists by using thein
keyword, like this:if "key" in my_dict:
. -
Can dictionary keys be of any data type?
Yes, dictionary keys can be of any immutable data type, such as strings, numbers, or tuples.
-
What happens if I try to access a non-existent key?
If you attempt to access a non-existent key using square brackets, Python raises aKeyError
. Using theget()
method can help avoid this issue. -
How can I remove an item from a dictionary?
You can remove an item using thedel
statement or thepop()
method, which also returns the value of the removed key.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn