How to Get Dictionary Value in Python
-
Dictionary Get Value in Python Using the
dict.get(key)
Method -
Dictionary Get Value in Python Using the
dict[key]
Method
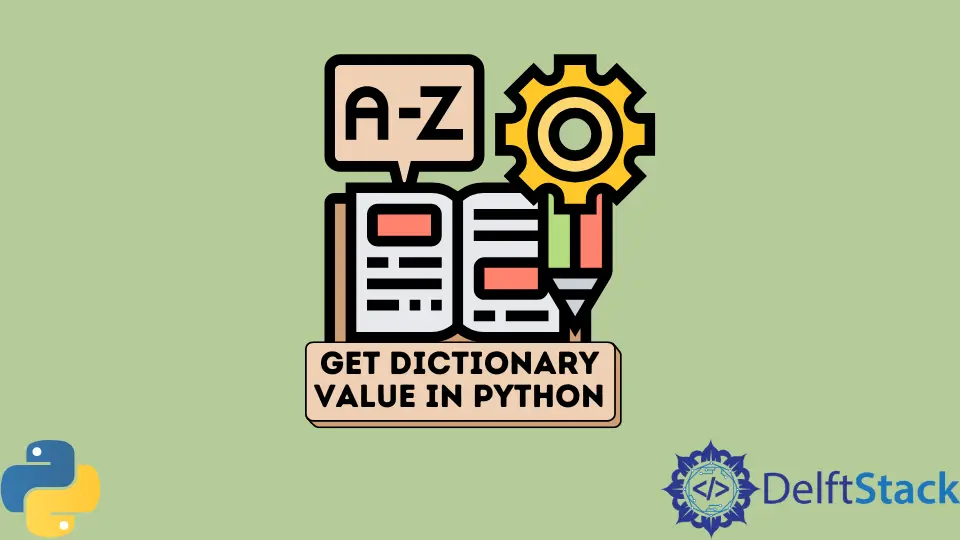
This tutorial will look into the multiple methods to get the value for the key from the dictionary in Python. Dictionary is one of the default data types in Python. It is an ordered collection of data used to store the data as key-value pairs. We can get the value of the key stored in the dictionary using the following methods in Python.
Dictionary Get Value in Python Using the dict.get(key)
Method
The get(key[, default])
method takes the key
as input and returns the value
of the input key
stored in the dictionary as output. The method returns the user’s default
value if the key
is not in the dictionary. The method will return None
as output if no default
value is provided.
The advantage of using this method is that we do not have to worry about the KeyError
exception, as it returns the default
value or None
as output in case of exception. The example below demonstrates how to use the dict.get()
method to get the value
of the key
in Python.
mydict = {0: "a", 1: "b", 2: "c", 3: "d", 5: "e"}
print(mydict.get(1))
print(mydict.get(4))
print(mydict.get(4, "KeyNotFound"))
Output:
b
None
KeyNotFound
Dictionary Get Value in Python Using the dict[key]
Method
The dict[key]
method takes the key
as input and returns the value
of the key
stored in the dictionary. Unlike the dict.get()
method the dict[key]
method raises the KeyError
exception if the key
is not present in the dictionary. Therefore the KeyError
exception has to be handled separately if the dict[key]
method is used to get the value
from the dictionary.
The following code example demonstrates how to use the dict[key]
method to get the value
stored in the dictionary in Python.
mydict = {0: "f", 1: "g", 2: "h", 3: "i", 5: "j"}
print(mydict[2])
Output:
h
We can handle the KeyError
exception when the key
is not found in the following way.
mydict = {0: "f", 1: "g", 2: "h", 3: "i", 5: "j"}
try:
print(mydict[2])
print(mydict[4])
except KeyError:
print("KeyNotFound!")
Output:
h
KeyNotFound!