How to Check Operating System Using Python
-
Using the
platform
Module -
Using the
sys
Module -
Combining
platform
andsys
for Detailed Information - Conclusion
- FAQ
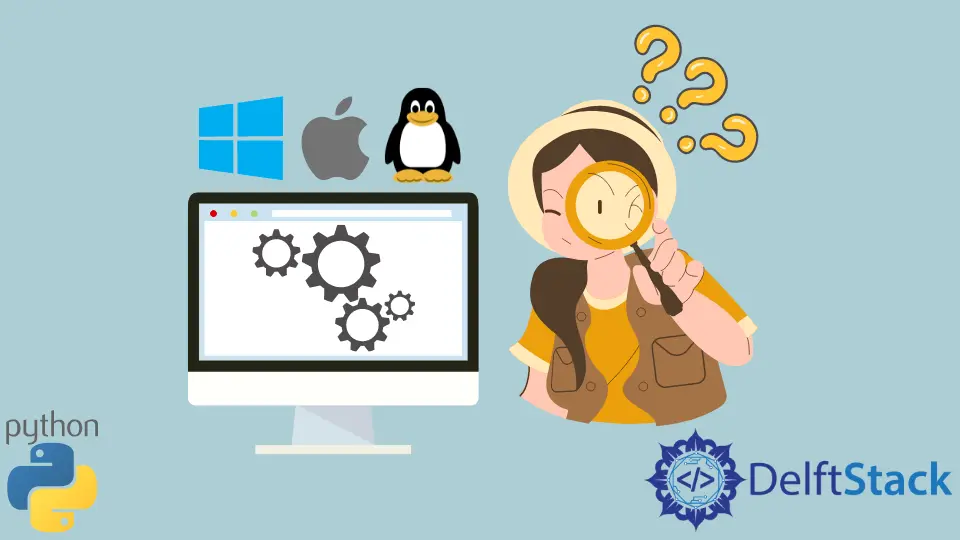
Detecting the operating system (OS) you are working on is a fundamental task in programming. Whether you’re developing applications, automating tasks, or simply trying to understand your environment better, knowing the OS can significantly influence how you write your code. In Python, the platform
and sys
modules provide powerful tools to identify the operating system in use.
This article will guide you through various methods to check the operating system using Python, complete with clear code examples and detailed explanations. By the end of this guide, you’ll be equipped to seamlessly integrate OS detection into your Python projects.
Using the platform
Module
The platform
module in Python is a robust way to retrieve information about the underlying platform, including the operating system. This module provides functions that can return detailed information, such as the OS name, version, and architecture. Here’s how you can use it:
import platform
os_name = platform.system()
os_version = platform.version()
os_release = platform.release()
print(f"Operating System: {os_name}")
print(f"Version: {os_version}")
print(f"Release: {os_release}")
Output:
Operating System: Windows
Version: 10.0.19042
Release: 10
The code above starts by importing the platform
module. It then uses platform.system()
to get the name of the operating system, which could return values like ‘Windows’, ‘Linux’, or ‘Darwin’ for macOS. The platform.version()
function provides the version number of the operating system, while platform.release()
gives the release number. This method is straightforward and effective for most use cases, making it a go-to solution for developers needing OS information.
Using the sys
Module
Another way to check the operating system in Python is by using the sys
module. This module offers a more straightforward approach to get the OS name and some additional platform-specific information. Here’s a simple example:
import sys
os_name = sys.platform
print(f"Operating System: {os_name}")
Output:
Operating System: win32
In this code snippet, we import the sys
module and use sys.platform
to get a string that indicates the platform on which Python is running. This string can return values like ‘win32’ for Windows, ’linux’ for Linux distributions, and ‘darwin’ for macOS. While this method may not provide as much detail as the platform
module, it is still useful for quickly checking the OS type, especially in scripts where simplicity is key.
Combining platform
and sys
for Detailed Information
For developers who need comprehensive information about the operating system, combining the platform
and sys
modules can yield excellent results. This approach allows you to gather both high-level and detailed information about the OS. Here’s how to implement this:
import platform
import sys
os_info = {
"System": platform.system(),
"Release": platform.release(),
"Version": platform.version(),
"Platform": sys.platform,
"Architecture": platform.architecture()
}
for key, value in os_info.items():
print(f"{key}: {value}")
Output:
System: Windows
Release: 10
Version: 10.0.19042
Platform: win32
Architecture: ('64bit', 'WindowsPE')
In this example, we create a dictionary called os_info
that captures various details about the operating system. Using a loop, we print out each piece of information in a clear format. This method provides a well-rounded view of the OS environment, including the architecture, which can be crucial for developers working on applications that need to cater to specific system requirements. By combining the strengths of both modules, you can easily adapt your code to different environments.
Conclusion
Knowing how to check the operating system using Python is a valuable skill for any developer. The platform
and sys
modules provide easy-to-use functions that can help you retrieve essential information about the environment in which your code is running. Whether you need basic OS identification or detailed system architecture, Python has you covered. By incorporating these methods into your projects, you can enhance compatibility and improve user experience. So, the next time you write a Python script, remember to check the operating system first!
FAQ
-
How can I check if my Python code is running on Windows?
You can use theplatform.system()
function to check if the OS is ‘Windows’. -
Is there a way to get the OS version in Python?
Yes, you can useplatform.version()
to retrieve the OS version. -
Can I use the
sys
module to check the operating system?
Absolutely! Thesys.platform
attribute provides a string indicating the platform. -
What is the difference between
platform.system()
andsys.platform
?
platform.system()
gives the OS name, whilesys.platform
returns a string indicating the specific platform type. -
Can I determine the architecture of my operating system using Python?
Yes, you can useplatform.architecture()
to get the architecture details of your OS.