How to Calculate Derivative in Python
- What is SymPy?
- Calculating Derivatives of Simple Functions
- Calculating Derivatives of Trigonometric Functions
- Calculating Higher-Order Derivatives
- Using Lambdify for Numerical Evaluation
- Conclusion
- FAQ
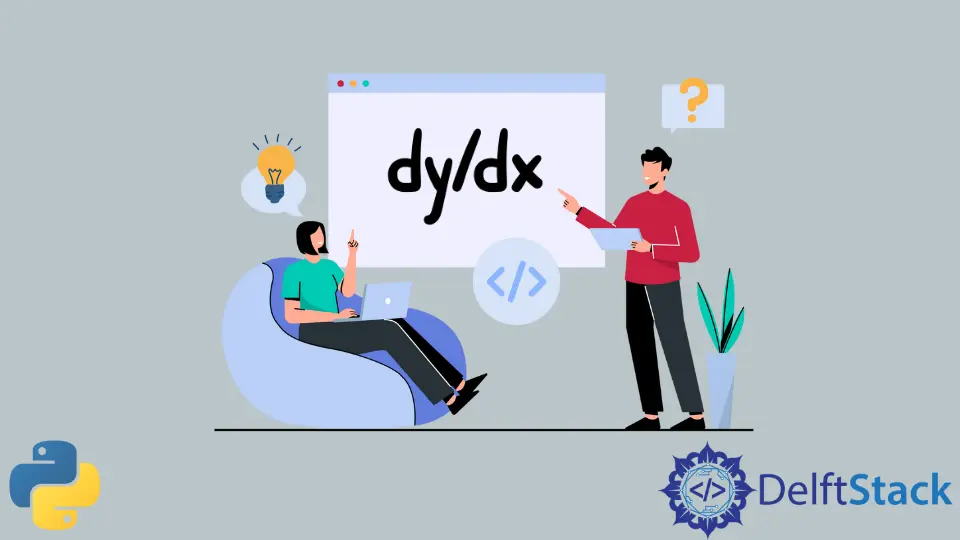
Calculating derivatives is an essential aspect of calculus, often used in various fields such as physics, engineering, and economics. If you’re a Python enthusiast or a data scientist, you’re in luck! The SymPy library makes it incredibly easy to perform symbolic mathematics, including derivative calculations.
In this article, we’ll explore how to calculate derivatives in Python using SymPy. Whether you’re looking to differentiate simple polynomials or complex functions, you’ll find that SymPy provides a straightforward approach. Let’s dive into the world of derivatives and see how we can implement them in Python.
What is SymPy?
Before we jump into the code, let’s understand what SymPy is. SymPy is a powerful Python library for symbolic mathematics. It allows you to perform operations such as differentiation, integration, and simplification of mathematical expressions symbolically rather than numerically. This means you can work with formulas in their exact forms, which is particularly useful for calculus.
To get started, you’ll need to install the SymPy library if you haven’t already. You can do this using pip:
pip install sympy
Once installed, you can import it into your Python script, and you’re ready to go!
Calculating Derivatives of Simple Functions
Let’s start with a basic example: calculating the derivative of a simple polynomial function. Suppose we want to differentiate the function f(x) = x^2 + 3x + 5. Here’s how you can do it using SymPy:
import sympy as sp
x = sp.symbols('x')
function = x**2 + 3*x + 5
derivative = sp.diff(function, x)
print(derivative)
Output:
2*x + 3
In this code, we first import the SymPy library and define a symbolic variable x
. We then create a function f(x)
as a polynomial expression. The sp.diff()
function is used to calculate the derivative with respect to x
. The result, 2*x + 3
, is printed out, which is the derivative of our original function.
This method is straightforward and allows you to handle simple functions easily. The beauty of using SymPy is that it automatically simplifies the result for you, making it easy to read and understand.
Calculating Derivatives of Trigonometric Functions
Next, let’s see how to calculate derivatives for trigonometric functions. Consider the function f(x) = sin(x) + cos(x). Here’s how you can differentiate it:
import sympy as sp
x = sp.symbols('x')
function = sp.sin(x) + sp.cos(x)
derivative = sp.diff(function, x)
print(derivative)
Output:
cos(x) - sin(x)
In this example, we again start by importing SymPy and defining our symbolic variable x
. We then define our function using sp.sin()
and sp.cos()
for the sine and cosine functions, respectively. The sp.diff()
function calculates the derivative, which results in cos(x) - sin(x)
.
This method showcases how SymPy can handle trigonometric functions seamlessly. It’s particularly useful for those working in fields like physics or engineering, where such functions frequently appear.
Calculating Higher-Order Derivatives
Sometimes, you may need to calculate higher-order derivatives. For instance, let’s differentiate the function f(x) = e^x multiple times. Here’s how you can do that:
import sympy as sp
x = sp.symbols('x')
function = sp.exp(x)
first_derivative = sp.diff(function, x)
second_derivative = sp.diff(first_derivative, x)
print(first_derivative)
print(second_derivative)
Output:
exp(x)
exp(x)
In this code, we define the function as sp.exp(x)
for the exponential function. We first calculate the first derivative using sp.diff()
, which gives us exp(x)
. We then calculate the second derivative by applying sp.diff()
again on the first derivative. Interestingly, the derivative of e^x is e^x itself, which you can see from the output.
This method is particularly useful in advanced mathematics and physics, where you often deal with higher-order derivatives in differential equations.
Using Lambdify for Numerical Evaluation
While SymPy is excellent for symbolic differentiation, you might also need to evaluate derivatives numerically. For this purpose, you can use the lambdify()
function. This function converts a SymPy expression into a function that can be evaluated numerically. Let’s see how it works:
import sympy as sp
x = sp.symbols('x')
function = x**2 + 3*x + 5
derivative = sp.diff(function, x)
numerical_derivative = sp.lambdify(x, derivative)
result = numerical_derivative(2)
print(result)
Output:
7
In this example, we first compute the derivative as before. We then use sp.lambdify()
to create a numerical function from our symbolic derivative. When we call this function with the value 2
, it returns 7
, which is the slope of the tangent line to the function at that point.
This method is particularly useful when you want to evaluate the derivative at specific points, making it a versatile tool for applications in data analysis and machine learning.
Conclusion
Calculating derivatives in Python using the SymPy library is both straightforward and powerful. Whether you’re working with simple polynomials, trigonometric functions, or even higher-order derivatives, SymPy provides a comprehensive set of tools to help you achieve your goals. Additionally, the ability to convert symbolic expressions into numerical functions with lambdify()
adds an extra layer of flexibility. By mastering these techniques, you’ll be well-equipped to handle various mathematical challenges in your programming endeavors.
FAQ
-
What is SymPy?
SymPy is a Python library for symbolic mathematics that allows for operations like differentiation, integration, and simplification. -
How do I install SymPy?
You can install SymPy using pip with the command pip install sympy. -
Can I compute higher-order derivatives using SymPy?
Yes, you can compute higher-order derivatives by repeatedly using the sp.diff() function. -
What is the purpose of the lambdify function?
The lambdify function converts a SymPy expression into a numerical function that can be evaluated at specific points. -
Is SymPy suitable for complex mathematical functions?
Yes, SymPy can handle a wide variety of mathematical functions, including polynomials, trigonometric functions, and exponentials.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn