How to Create Directory in Python
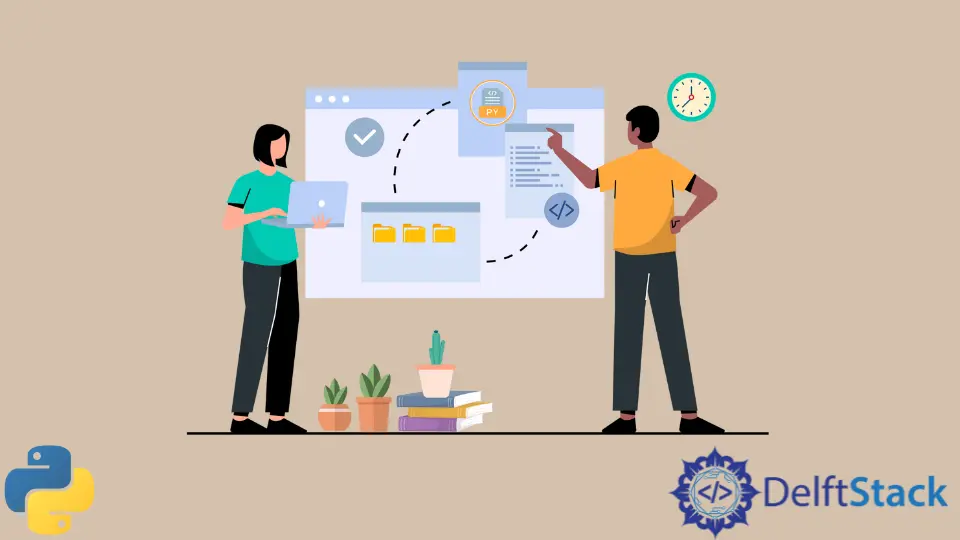
Creating directories in Python is a fundamental skill for any programmer, whether you are organizing files for a project or simply managing your data.
In this tutorial, we will dive into how to check if a directory exists and create it if it doesn’t. This is particularly useful when you want to ensure that your program has a designated space for saving files, logs, or any other data. By the end of this guide, you’ll be equipped with the knowledge to handle directories efficiently in your Python projects.
Using the os Module
One of the most common ways to create a directory in Python is by using the os
module. This built-in module provides a portable way of using operating system-dependent functionality, including file and directory management. The os.makedirs()
function is particularly useful because it allows you to create a directory along with any necessary parent directories.
Here’s how you can do it:
import os
directory = "new_directory"
if not os.path.exists(directory):
os.makedirs(directory)
print("Directory created")
else:
print("Directory already exists")
Output:
Directory created
In this code, we first import the os
module, which gives us access to various operating system functionalities. We then define a variable directory
that holds the name of the directory we want to create. The os.path.exists()
method checks if the directory already exists. If it doesn’t, os.makedirs()
creates the directory, and we print a confirmation message. If the directory is already present, we inform the user accordingly. This method is straightforward and effective for managing directories.
Using the pathlib Module
Another modern approach to creating directories in Python is by using the pathlib
module, which was introduced in Python 3.4. This module offers an object-oriented interface for filesystem paths, making it easier to work with files and directories. The Path
class in pathlib
has a method called mkdir()
that can create a directory.
Here’s an example of how to use pathlib
:
from pathlib import Path
directory = Path("new_directory")
if not directory.exists():
directory.mkdir(parents=True)
print("Directory created")
else:
print("Directory already exists")
Output:
Directory created
In this snippet, we import the Path
class from the pathlib
module. We then create a Path
object representing the desired directory. The exists()
method checks if the directory already exists. If it does not, we call mkdir()
, passing parents=True
to ensure that any necessary parent directories are created as well. This method is particularly useful for creating nested directories in one go. The use of pathlib
makes the code cleaner and more intuitive, especially for those who prefer object-oriented programming.
Handling Exceptions
While creating directories, it’s essential to handle potential exceptions that may arise, such as permission errors or invalid directory names. Python’s built-in exception handling can help you manage these scenarios gracefully. By using a try-except block, you can catch exceptions and take appropriate actions.
Here’s how you can implement error handling:
import os
directory = "new_directory"
try:
os.makedirs(directory)
print("Directory created")
except FileExistsError:
print("Directory already exists")
except PermissionError:
print("Permission denied: unable to create directory")
except Exception as e:
print(f"An error occurred: {e}")
Output:
Directory created
In this code, we use a try-except block to attempt the directory creation with os.makedirs()
. If the directory already exists, a FileExistsError
is raised, which we catch and handle by informing the user. Similarly, if there’s a PermissionError
, we notify the user about the permission issue. The final catch-all except Exception as e
helps capture any other unexpected errors and provides feedback. This approach ensures that your program can handle various situations without crashing, making your code more robust and user-friendly.
Conclusion
Creating directories in Python is a straightforward process that can be accomplished using either the os
or pathlib
modules. By checking for existing directories and handling exceptions, you can ensure that your applications run smoothly and efficiently. Whether you are managing files for a small project or a larger application, mastering directory creation will significantly improve your programming skills. With the knowledge gained from this tutorial, you are now ready to implement directory management in your Python projects confidently.
FAQ
-
What is the difference between os.makedirs() and os.mkdir()?
os.makedirs() creates a directory and any necessary parent directories, while os.mkdir() creates a single directory and will raise an error if the parent directory does not exist. -
Can I create a directory with a path using pathlib?
Yes, you can create a directory with a path using pathlib by creating a Path object and calling the mkdir() method. -
What happens if I try to create a directory that already exists?
If you try to create a directory that already exists, Python will raise a FileExistsError unless you handle it using a try-except block. -
Is it necessary to check if a directory exists before creating it?
While it is not strictly necessary, checking if a directory exists before creating it helps avoid errors and allows you to manage your program flow more effectively. -
Can I create nested directories in Python?
Yes, you can create nested directories in Python using os.makedirs() or pathlib.Path.mkdir() with the parents=True argument.
Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python