Coverage in Python
-
Install the
pytest
Plugin for Coverage in Python -
Use the
pytest
Plugin for Statement Coverage in Python
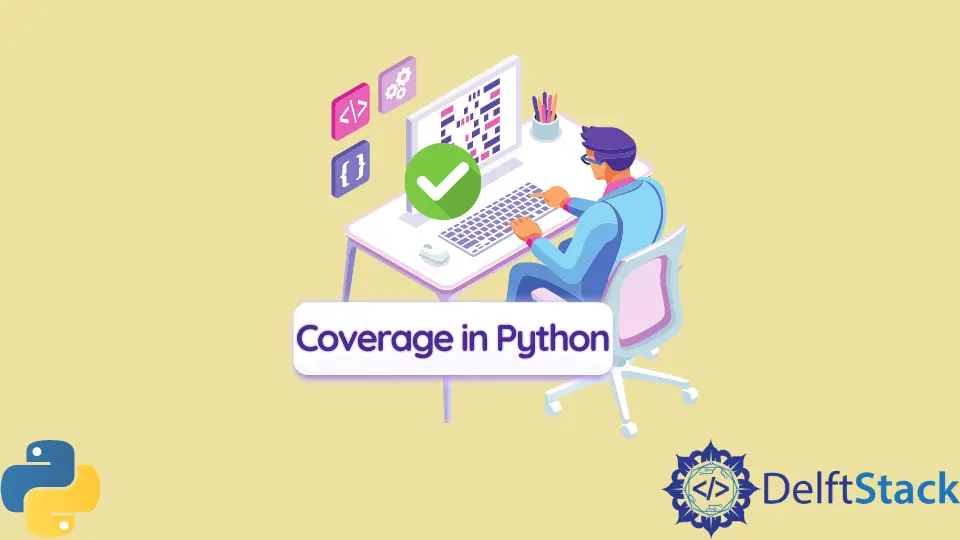
This article will discuss the uses of coverage in Python for testing the python program execution.
Code coverage means monitoring the code to check which part has been executed and has not. For this purpose, we use the pytest
plugin in Python.
Install the pytest
Plugin for Coverage in Python
To use this plugin, we should install it first using the following command.
bashCopy#Python 3.x
pip install pytest-cov
Use the pytest
Plugin for Statement Coverage in Python
Statement coverage, also known as line coverage, is white box testing. All the executable statements are executed at least once to ensure running without any error.
Statement coverage is the default coverage used by the coverage module in Python. It calculates the coverage based on the following formula.
textCopyStatement Coverage = {Number of statements executed / Total number of statements in the code} * 100
We will create a module, CovModule
, to run the coverage in our example. This will consist of the actual code to cover.
We will make another Python file, in which we will import this module and call the function.
In the following code, if the value of a
is greater than or equal to b
, then the if
block will execute, and the else
block will skip. So, coverage will be 75 percent.
Therefore, either if
or else
will execute in any situation. But the overall coverage of the code will be 100 percent.
pythonCopy# Python 3.x
# CovModule.py
def check(num1, num2):
if num1 <= num2:
print("if executed")
else:
print("else executed")
pythonCopy# Python 3.x
# test.py
from CovModule import check
check(2, 3)
Finally, we will run the following command to run the code coverage and generate its report. We will see the module name, the total number of statements, missed statements and executed statements in the report.
bashCopypytest --cov CovModule test.py
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn