How to Count Unique Values in Python List
-
Use
collections.counter
to Count Unique Values in Python List -
Use
set
to Count Unique Values in Python List -
Use
numpy.unique
to Count the Unique Values in Python List
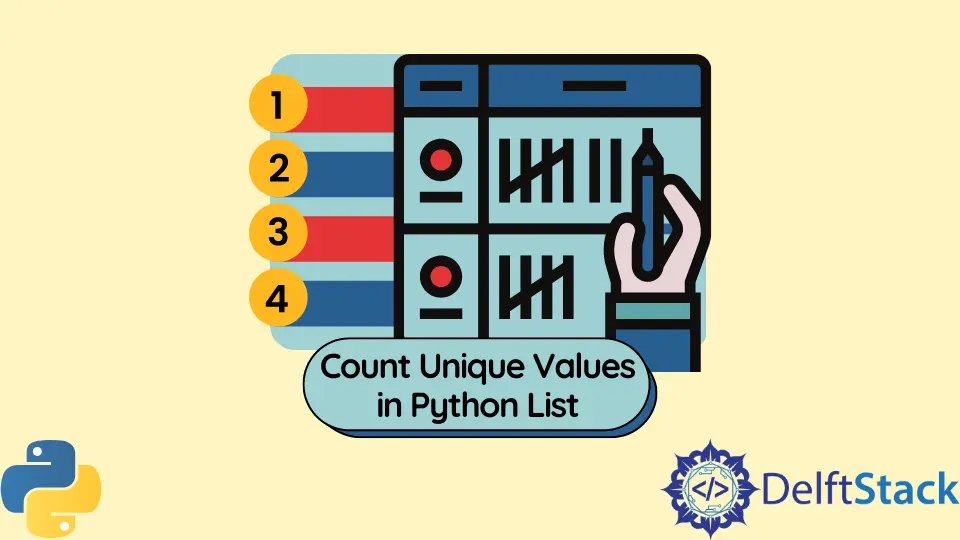
This article will introduce different methods to count unique values inside list. using the following methods:
collections.Counter
set(listName)
np.unique(listName)
Use collections.counter
to Count Unique Values in Python List
collections
is a Python standard library, and it contains the Counter
class to count the hashable objects.
Counter
class has two methods :
keys()
returns the unique values in the list.values()
returns the count of every unique value in the list.
We can use the len()
function to get the number of unique values by passing the Counter
class as the argument.
Example Codes:
from collections import Counter
words = ["Z", "V", "A", "Z", "V"]
print(Counter(words).keys())
print(Counter(words).values())
print(Counter(words))
Output:
['V', 'A', 'Z']
[2, 1, 2]
3
Use set
to Count Unique Values in Python List
set
is an unordered collection data type that is iterable, mutable, and has no duplicate elements. We can get the length of the set
to count unique values in the list after we convert the list to a set
using the set()
function.
Example Codes:
words = ["Z", "V", "A", "Z", "V"]
print(len(set(words)))
Output:
3
Use numpy.unique
to Count the Unique Values in Python List
numpy.unique
returns the unique values of the input array-like data, and also returns the count of each unique value if the return_counts
parameter is set to be True
.
Example Codes:
import numpy as np
words = ["Z", "V", "A", "Z", "V"]
np.unique(words)
print(len(np.unique(words)))
Output:
3
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python