How to Convert String to Unicode in Python
- Understanding Unicode in Python
- Converting Strings to Unicode in Python 2
- Working with Unicode in Python 3
- Handling Special Characters and Encodings
- Conclusion
- FAQ
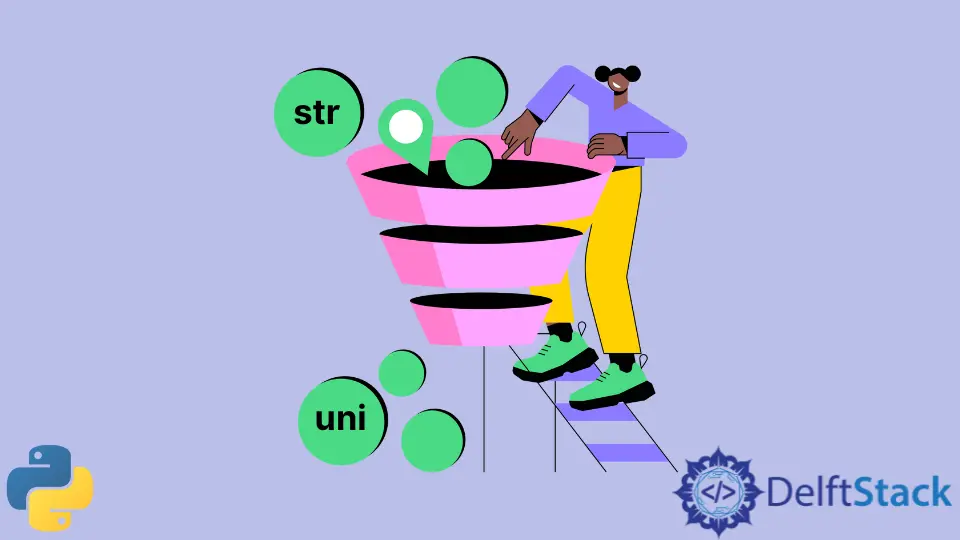
When working with strings in Python, understanding how to convert them to Unicode is crucial, especially if you deal with internationalization or special characters. In Python 2, converting strings to Unicode was a common task, achieved using the unicode()
function. However, with the advent of Python 3, things changed significantly. All strings in Python 3 are Unicode by default, simplifying the process for developers.
This article will explore the nuances of string conversion in both versions of Python, providing clear examples and explanations to help you grasp this essential concept. Whether you’re transitioning from Python 2 to Python 3 or just starting your programming journey, this guide will equip you with the knowledge you need.
Understanding Unicode in Python
Unicode is a universal character encoding standard that allows for the representation of text in various languages and symbols. In Python, strings are sequences of characters. In Python 2, a string is a byte sequence by default, which can lead to confusion when dealing with non-ASCII characters. The unicode()
function was introduced to handle this, allowing developers to convert byte strings to Unicode strings seamlessly.
In contrast, Python 3 simplifies this process by making all string literals Unicode by default. This means that when you create a string in Python 3, you are essentially working with a Unicode string. This change eliminates many common pitfalls associated with string handling, making it easier to work with text data from different languages and scripts.
Converting Strings to Unicode in Python 2
In Python 2, if you have a byte string and you want to convert it to a Unicode string, you can use the unicode()
function. This function takes two arguments: the string to convert and the encoding. The most common encoding is utf-8
, which supports a wide range of characters.
Here’s how you can do it:
byte_string = "Hello, world!"
unicode_string = unicode(byte_string, "utf-8")
print(unicode_string)
Output:
Hello, world!
In this example, we start with a byte string byte_string
. We then use the unicode()
function to convert it to a Unicode string, specifying utf-8
as the encoding. The result is stored in unicode_string
, which is printed to the console.
This conversion is essential when working with text that may contain special characters or symbols not represented in the ASCII character set. By converting to Unicode, you ensure that your strings can represent a broader array of characters, making your applications more versatile and international-friendly.
Working with Unicode in Python 3
In Python 3, the handling of strings has been significantly improved. All strings are Unicode by default, which means you don’t need to perform any explicit conversion for standard string literals. However, if you are dealing with byte strings (which are represented by the bytes
type), you may need to decode them into Unicode.
Here’s how you can convert a byte string to a Unicode string in Python 3:
byte_string = b"Hello, world!"
unicode_string = byte_string.decode("utf-8")
print(unicode_string)
Output:
Hello, world!
In this code snippet, we start with a byte string byte_string
, which is prefixed with b
to indicate that it is a byte literal. We then use the decode()
method to convert it into a Unicode string. The result is stored in unicode_string
, which is printed to the console.
This approach is particularly useful when reading data from files or network sources where the data might be in byte format. By decoding it, you ensure that your application can handle and display the text correctly, regardless of the characters involved.
Handling Special Characters and Encodings
When converting strings to Unicode, it’s essential to consider the encoding of the original string. Different encodings can represent the same characters in various ways, leading to potential issues if not handled properly. The most commonly used encoding is utf-8
, which can represent any character in the Unicode standard.
Here’s an example that demonstrates how to handle special characters:
byte_string = "Café"
unicode_string = unicode(byte_string, "utf-8") # For Python 2
# In Python 3, you would use:
# unicode_string = byte_string.decode("utf-8")
print(unicode_string)
Output:
Café
In this example, the byte string byte_string
contains a special character, the accented ‘é’. By using the appropriate encoding, we can convert the byte string to a Unicode string, preserving the special character correctly.
For Python 3 users, the conversion would involve decoding the byte string using the decode()
method, ensuring that the special characters are accurately represented. This kind of handling is crucial for applications that require support for multiple languages and character sets, making your code robust and reliable.
Conclusion
Understanding how to convert strings to Unicode in Python is vital for any developer working with text data. In Python 2, the unicode()
function provides a straightforward way to handle this conversion, while Python 3 simplifies the process by making all strings Unicode by default. By mastering these techniques, you can ensure your applications handle text data efficiently and accurately, regardless of the language or characters involved. Whether you’re maintaining legacy code or developing new applications, this knowledge will serve you well in your programming endeavors.
FAQ
-
What is the difference between Python 2 and Python 3 regarding strings?
In Python 2, strings are byte sequences by default, while in Python 3, all strings are Unicode by default. -
How do I convert a byte string to a Unicode string in Python 2?
You can use theunicode()
function, specifying the string and its encoding, typicallyutf-8
. -
What method is used to decode byte strings in Python 3?
You can use thedecode()
method on byte strings to convert them to Unicode. -
Why is Unicode important in programming?
Unicode allows for the representation of text in multiple languages and scripts, making applications more versatile and user-friendly. -
Can I handle special characters in Python?
Yes, by using appropriate encodings likeutf-8
, you can handle and represent special characters correctly in your strings.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn