How to Convert List of Strings to Integer in Python
-
Python Convert List of Strings to Integers Using the
map()
Function - Python Convert List of String to Integers Using List Comprehension Method
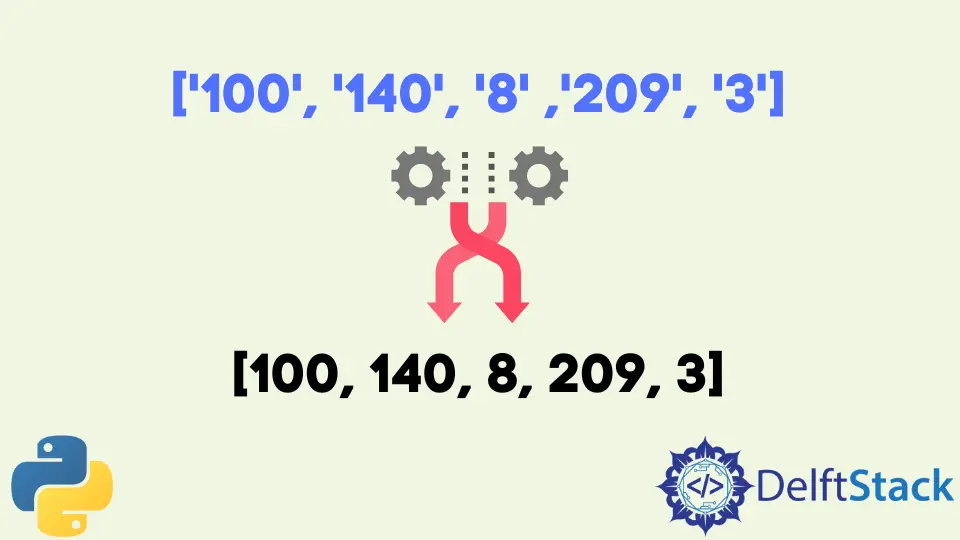
This tutorial will explain various methods to convert a list of strings to a list of integers in Python. In many cases, we need to extract numerical data from strings and save it as an integer; for example, it can be a price of an item saved as a string or some identity number saved as a string.
Python Convert List of Strings to Integers Using the map()
Function
The map(function, iterable)
function applies function
to each element of the iterable
and returns an iterator.
To convert a list of strings to the list of integers, we will give int
as the function
to the map()
function and a list of strings as the iterable
object. Because the map()
function in Python 3.x returns an iterator, we should use the list()
function to convert it to the list.
string_list = ["100", "140", "8", "209", "50" "92", "3"]
print(string_list)
int_list = list(map(int, string_list))
print(int_list)
Output:
['100', '140', '8', '209', '5092', '3']
[100, 140, 8, 209, 5092, 3]
Python Convert List of String to Integers Using List Comprehension Method
The other way we can use to convert the list of strings to the list of integers is to use list comprehension. The list comprehension creates a new list from the existing list. As we want to create a list of integers from a list of strings, the list comprehension method can be used for this purpose.
The code example below demonstrates how to use the list comprehension method to convert a list from string to integer.
string_list = ["100", "140", "8", "209", "50" "92", "3"]
print(string_list)
int_list = [int(i) for i in string_list]
print(int_list)
Output:
['100', '140', '8', '209', '5092', '3']
[100, 140, 8, 209, 5092, 3]
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python