How to Clamp Numbers Within a Range Using Python
-
Method 1: Using the Built-in
max()
andmin()
Functions - Method 2: Using Conditional Statements
- Method 3: Using NumPy for Clamping
- Conclusion
- FAQ
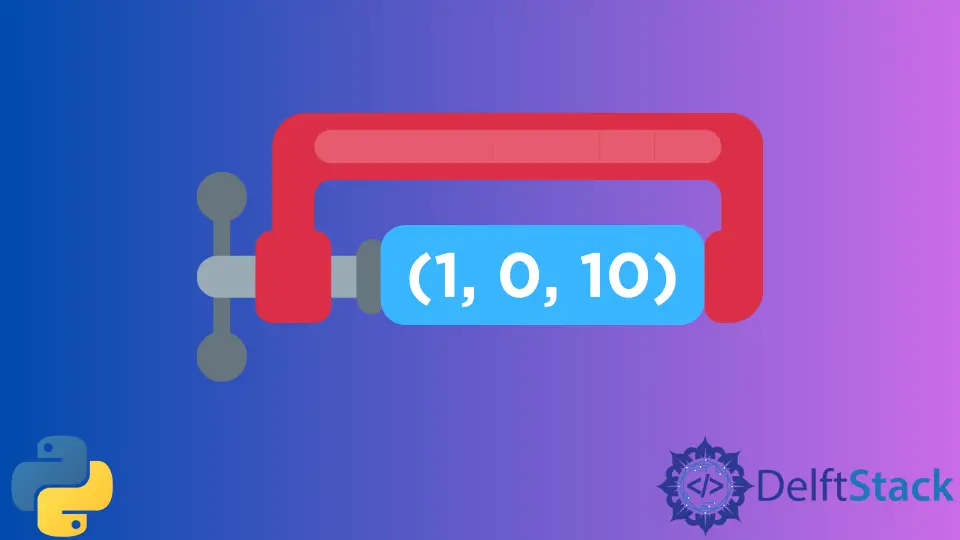
Clamping numbers within a specific range is a common task in programming, especially when dealing with user inputs or data that needs to conform to certain limits. In Python, this can be achieved in several straightforward ways. Whether you are working with values that need to be restricted to prevent errors or simply want to ensure that your data remains within expected boundaries, understanding how to clamp numbers effectively is essential.
In this article, we will explore different methods to clamp numbers within a range using Python, providing clear examples and explanations to help you grasp the concept quickly. So, let’s dive in and learn how to keep our numbers in check!
Method 1: Using the Built-in max()
and min()
Functions
One of the simplest and most effective ways to clamp a number within a range in Python is by using the built-in max()
and min()
functions. This method is both readable and efficient. The idea is to first use the max()
function to ensure that the number is not less than the minimum value of the range. Then, you apply the min()
function to ensure that the number does not exceed the maximum value of the range.
Here’s a quick example to illustrate this:
def clamp(value, min_value, max_value):
return max(min(value, max_value), min_value)
result = clamp(15, 10, 20)
Output:
15
In this example, we define a function called clamp
that takes three parameters: value
, min_value
, and max_value
. The function first clamps the input value to the maximum value using min(value, max_value)
. Then, it clamps the result to the minimum value using max()
. If the input value is within the specified range (10 to 20), it will return the value itself; otherwise, it will return the closest boundary. This method is straightforward and easy to understand, making it an excellent choice for beginners.
Method 2: Using Conditional Statements
Another approach to clamp numbers within a range is by using simple conditional statements. This method offers more control and flexibility, allowing you to customize the clamping behavior based on specific conditions. While it may be slightly more verbose than the previous method, it can be useful in situations where you want to add additional logic.
Here’s how you can implement this:
def clamp(value, min_value, max_value):
if value < min_value:
return min_value
elif value > max_value:
return max_value
else:
return value
result = clamp(25, 10, 20)
Output:
20
In this example, the clamp
function checks if the input value is less than the min_value
. If it is, the function returns the min_value
. If the value is greater than the max_value
, it returns the max_value
. If the value is within the range, it simply returns the original value. This method is particularly useful if you need to add more complex conditions or actions based on the clamped value.
Method 3: Using NumPy for Clamping
For those working with numerical data in Python, especially in scientific computing, the NumPy library provides an efficient way to clamp numbers. NumPy’s clip()
function allows you to specify the minimum and maximum limits directly, making it an excellent choice for handling arrays of numbers.
Here’s an example of how to use NumPy for clamping:
import numpy as np
values = np.array([5, 15, 25, 35])
clamped_values = np.clip(values, 10, 20)
Output:
[10 15 20 20]
In this example, we create a NumPy array containing several values. We then use the np.clip()
function, which takes three arguments: the array to clamp, the minimum value, and the maximum value. The function returns a new array where each element is clamped to the specified range. This method is extremely efficient for large datasets, as NumPy is optimized for performance. If you’re processing large amounts of numerical data, using NumPy can significantly speed up your operations while keeping your code clean and concise.
Conclusion
Clamping numbers within a range is a valuable technique in Python programming. Whether you choose to use the built-in functions, conditional statements, or the powerful NumPy library, each method has its advantages and can be applied based on your specific needs. Understanding how to effectively clamp numbers will help you write more robust and error-free code. As you become more familiar with these techniques, you’ll find that controlling data flow in your applications becomes easier and more intuitive. So go ahead and implement these methods in your projects to enhance your programming skills!
FAQ
-
What is clamping in programming?
Clamping refers to restricting a value to a specified range, ensuring it does not fall below a minimum or exceed a maximum limit. -
Can I clamp numbers in Python without using libraries?
Yes, you can easily clamp numbers using built-in functions likemax()
andmin()
, or by using conditional statements.
-
What is the benefit of using NumPy for clamping?
NumPy is optimized for performance and can efficiently handle large arrays of numbers, making it a great choice for numerical data processing. -
Are there any performance considerations when clamping large datasets?
Using libraries like NumPy can significantly improve performance when dealing with large datasets compared to using standard Python lists and loops. -
Can clamping be used in real-world applications?
Yes, clamping is commonly used in applications like graphics programming, data validation, and any scenario where data needs to conform to specific limits.