How to Check if a Variable Exists in Python
-
Check if a Variable Exists in Python Using the
locals()
Method -
Check if a Variable Exists in Python Using the
globals()
Method
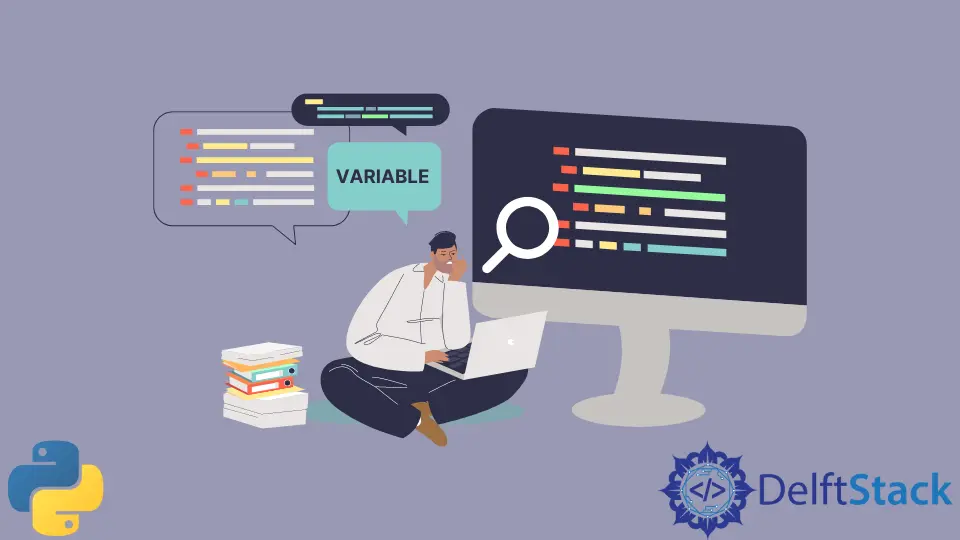
Exceptions can be used to check whether a variable exists in Python, but it is not a recommended solution as, in some cases, we do not know whether the variable is defined or not. Some other ways are helpful in checking the variable existence in Python.
This article will discuss the different methods to check if a variable exists in Python.
Check if a Variable Exists in Python Using the locals()
Method
This method will check the existence of the local variable using the locals()
function. locals()
returns a dictionary whose keys are strings of variables’ names that are present in the local namespace.
Let’s first make a user-defined function named local_func()
, it defines a variable and initializes the value in it. We can use the in
operator to check if the string of the variable name exists in the dictionary. If so, it means the variable exists in the local namespace; otherwise, not.
The complete example code is as follows:
def local_func():
var = "Test"
if "var" in locals():
print("var variable exists")
else:
print("var variable does not exist in the local namespace")
local_func()
Output:
var variable exists
Check if a Variable Exists in Python Using the globals()
Method
This function will check whether if a variable exists in the global namespace using the globals()
method. globals()
returns a dictionary whose keys are strings of variables’ names that are present in the global namespace.
We can use the in
operator to check if the string of the variable name exists in the dictionary. If so, it means the variable exists in the global namespace; otherwise, not.
The complete example code is as follow:
var2 = "Python"
if "var2" in globals():
print("var2: variable exist")
else:
print("var2: variable does not exist")
Output:
var2: variable exist
Related Article - Python Exception
- How to Open File Exception Handling in Python
- How to Mock Raise Exception in Python
- How to Rethrow Exception in Python
- How to Raise Exception in Python
- How to Handle NameError Exception in Python
- Python Except Exception as E