How to Check File Size in Python
-
Check File Size in Python Using the
pathlib
Module -
Check File Size in Python Using the
os.path()
Method -
Check File Size in Python Using the
os.stat
Method - Check File Size Using the File Object Method in Python
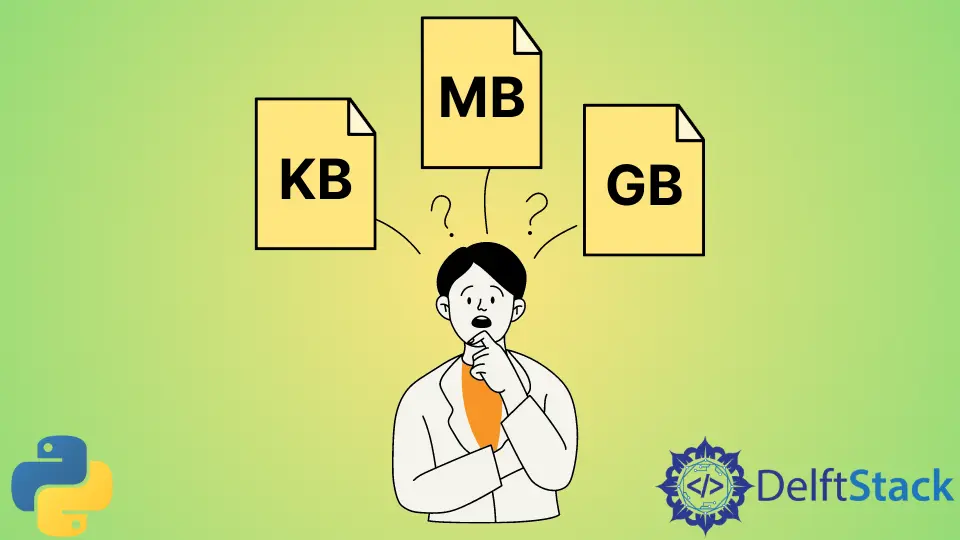
A file is a collection of different data stored in one unit. The file size evaluates how much space a file will take to store in a storage medium like a hard disk. It can be measured in different memory units like kilo bytes(kB), mega bytes(MB), and gigabytes(GB) etc.
This article will introduce different methods to check the file size in Python.
Check File Size in Python Using the pathlib
Module
The stat()
method of the Path
object returns the detailed properties of the file like st_mode
, st_dev
etc. st_size
attribute of the stat
method gives the file size in bytes.
The complete example code is as follows:
from pathlib import Path
Path(r"C:\test\file1.txt").stat()
file_size = Path(r"C:\test\file1.txt").stat().st_size
print("The file size is:", file_size, "bytes")
We must place r
before making the file path as the raw string; otherwise, we need to escape the backslash, like C:\\test\\file1.txt
.
Output:
The file size is: 40 bytes
Check File Size in Python Using the os.path()
Method
This Python’s module os.path
has a function getsize
) that returns the file size in bytes by taking the file path as the argument.
The complete example code is as follows:
import os
file_size = os.path.getsize(r"C:\test\file1.txt")
print("File Size:", file_size, "bytes")
Output:
File Size: 20 bytes
Check File Size in Python Using the os.stat
Method
This Python os
module also provides a stat
method to check the file size. It also takes the file path as the argument and returns a structure type object. This object has a st_size
attribute giving the file size in bytes.
The complete example code is as follows:
import os
file_size = os.stat(r"C:\test\file1.txt")
print("File Size is", file_size.st_size, "bytes")
Output:
Size of file is 40 bytes
Check File Size Using the File Object Method in Python
We pass the file path to the open()
function and get the file object that can be used for file handling operations.
The complete example code is as follows:
import os
with open(r"C:\test\file1.txt") as file_obj:
file_obj.seek(0, os.SEEK_END)
print("File Size is", file_obj.tell(), "bytes")
Output:
File Size is 40 bytes
After the desired file is open, the file cursor is at the start of the file. The seek
method of the file object will set the file cursor to the desired position.
file_obj.seek(0, os.SEEK_END)
The first argument of the seek()
method is the offset of the File
object, and the second argument is the reference position of the file. os.SEEK_END
specifies the reference position to the end of the file object.
Therefore, the above script line sets the cursor to the end of the file object.
The tell()
method of the file object returns the current cursor position. Because the cursor is already set to the end, so the result here is equal to the file size.