How to Catch All Exceptions in Python
-
Use the
Exception
Class to Catch All Exceptions in Python -
Use the
BaseException
Class to Catch All Exceptions in Python
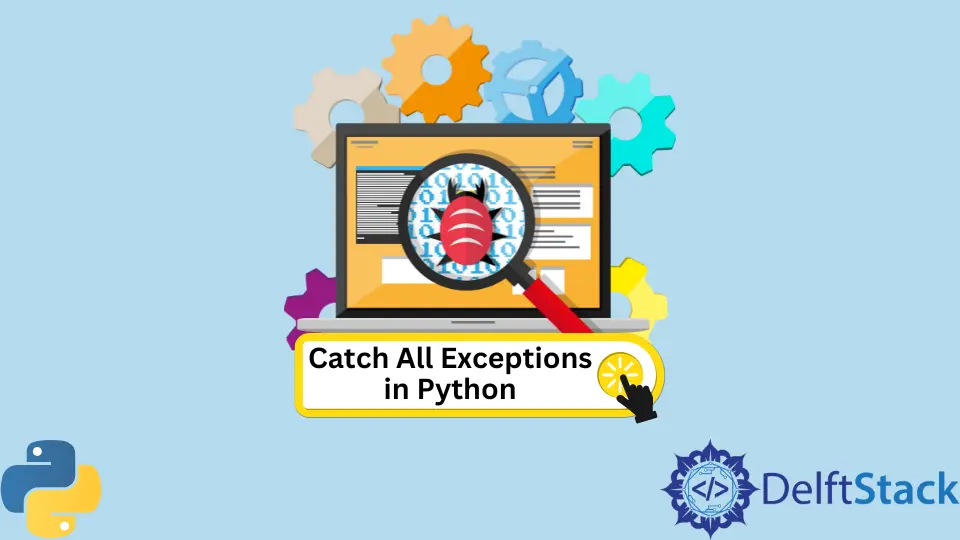
We use the try
and except
block to deal with exceptions. The try
block contains some code that may raise an exception. If an exception is raised, then we can specify the alternate code in the except
block that can be executed. We know that we have different types of exceptions in Python to have multiple except
statements for different exceptions.
For example,
try:
raise ValueError()
except ValueError:
print("Value Error")
except KeyError:
print("Key Error")
Output:
Value Error
However, at times, we may want a general except
block that can catch all exceptions. It is very simple to implement this. If we do not mention any specific exception in the except
block, then it catches any exception which might occur.
The following code implements this.
try:
# Your
# Code
pass
except:
print("Exception Encountered")
However, it is not advisable to use this method because it also catches exceptions like KeyBoardInterrupt
, and SystemExit
, which one usually wants to ignore.
Use the Exception
Class to Catch All Exceptions in Python
We can avoid the errors mentioned above by simply catching the Exception
class. All built-in, non-system-exiting exceptions, as well as user-defined exceptions, are usually derived from this class.
For example,
try:
# Your
# Code
pass
except Exception as e:
print("Exception Encountered")
Use the BaseException
Class to Catch All Exceptions in Python
It should be noted that even the above method may omit some exceptions. We can also use the BaseException
class, which is at the top of the hierarchy. It may be required in some cases, and we can see its use in the following code.
try:
# Your
# Code
pass
except BaseException as e:
print("Exception Encountered")
In this tutorial, we discussed a few methods, which may not be perfect but can catch most of the raised exceptions. In general, it is not advisable to catch all exceptions, so be cautious in whichever method you choose to use.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn