How to Use the cat Command in Python
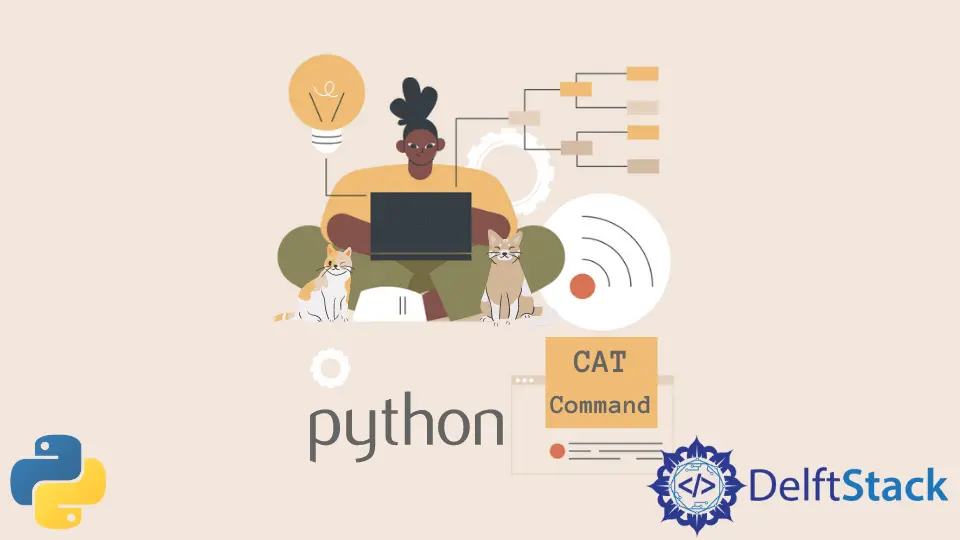
The cat
command is a shell command found in UNIX-based operating systems such as macOS and Linux. cat
is a short form for concatenate
.
This command is used to display the content of files, concatenate contents of multiple files into a single file, create single and multiple files.
In this article, we get to learn how to use the cat
command in the Python programming language.
Using the cat
Command in Python
Since the cat
command is a shell command, there is no direct way to access this command in Python scripts.
Interestingly, the Python programming language has utilities to execute shell commands right from the script. One such utility is the os
module.
The os
module has a method system()
that can execute shell commands. We can utilize the system()
method to execute Python’s cat
command.
Let us understand this with the help of an example. Refer to the following Python code.
import os
os.system("echo 'Hello! Python is the best programming language.' >> ~/file.txt")
os.system("cat ~/file.txt")
Output:
Hello! Python is the best programming language.
The Python script first creates a file named file.txt
in the current user’s home directory.
~/
is a shorthand for the home directory of the currently logged-in user. Next, it reads and displays the content of the created file using the cat
command.