The Callback Function in Python
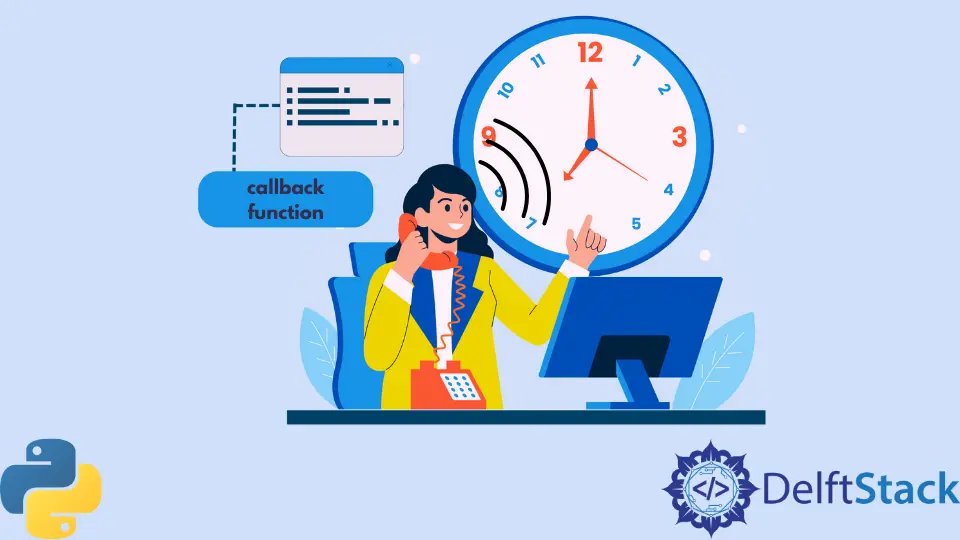
In this article, you’ll have a deeper understanding of the Python callback
function. Check out our sample program below, which demonstrates the function’s purpose.
Parallel Python
Parallel Python
is a module offered by Python that helps in providing a proper mechanism for the parallel execution of a program in Python. These Python codes are generally on SMP
or Systems with Multiple Processors and Clusters
, which are computers that are connected through a network.
This module is both open-source and cross-platform, which is written only in Python. It is a very light module and is also very easy to install with any Python software.
Callback Function Definition in Python
In the Parallel Python
Module, the submit
function is known as the callback
function. The callback
function acts as an argument for any other function. The other function in which the callback
function is an argument calls the callback
function in its function definition. Other modules can call the callback
function depending upon their requirements and their nature.
These callback
functions generally come into play when asynchronous functions are used in the program. An asynchronous function is a function that sometimes works out of sync or asynchronously through tasks like a loop.
The program below will demonstrate the use of a callback
function:
def Func_CallBack(c):
print("File Length : ", c)
def File_Len(filepath, callback):
i = open(filepath, "r")
file_length = len(i.read())
i.close()
callback(file_length)
if __name__ == "__main__":
File_Length("randomfile.txt", Func_CallBack)
In this example, we first define a function called Func_CallBack
, which returns the total length of the whole text file. Initially, the Func_CallBack
function takes the file path and the callback
modules as arguments. Finally, the function reads the whole file and returns the length of the file. In the end, the Func_CallBack
function calls to the callback
modules, which initially functioned as arguments.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn