How to Create a BitArray in Python
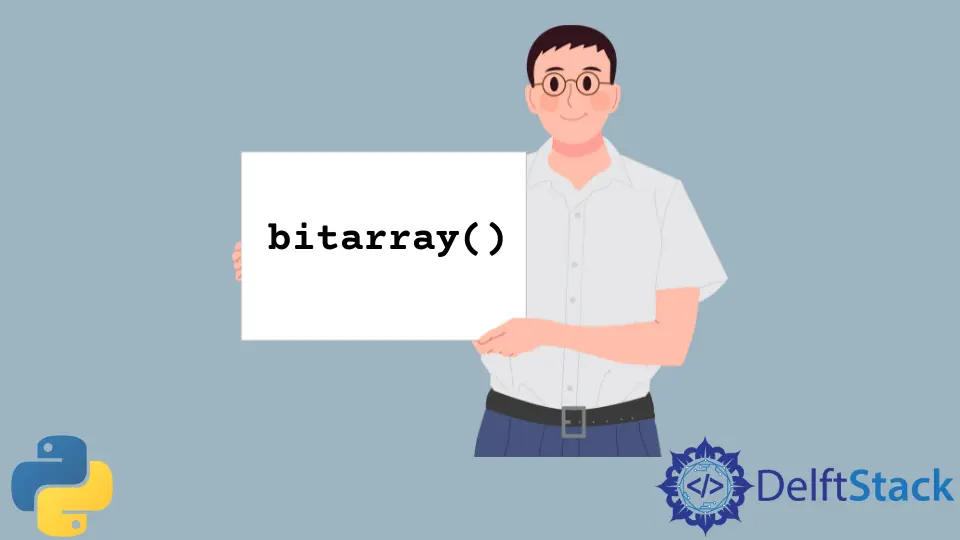
This guide will show you how to create a bitarray
in Python. There are some small complications along the way for some users; we’ll show you how to get around those as well. Let’s dive in.
Create a BitArray
in Python
In Python, you can use the function bitarray()
to initialize an array with bits. For instance, take a look at the following code.
a = bitarray(10)
print(a)
The above code will generate an array of 10 random bits. There are some cases in which it will not work. So, you need to download and install bitarray
in Python. You can use the following command in your command prompt.
pip install bitarray
Once installed, you can use the above code again or use the following code line.
# import the bitarray from the module bitarrray
from bitarray import bitarray
z = bitarray(15)
print(z)
You’ll need to import the bitarray
from the bitarray
module. Then create an array of random bits.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn