How to Have Beep Sound in Python
- Using the winsound Library
- Using the os System Command
- Using the winsound Library for Continuous Beeping
- Using the playsound Library for More Complex Sounds
- Conclusion
- FAQ
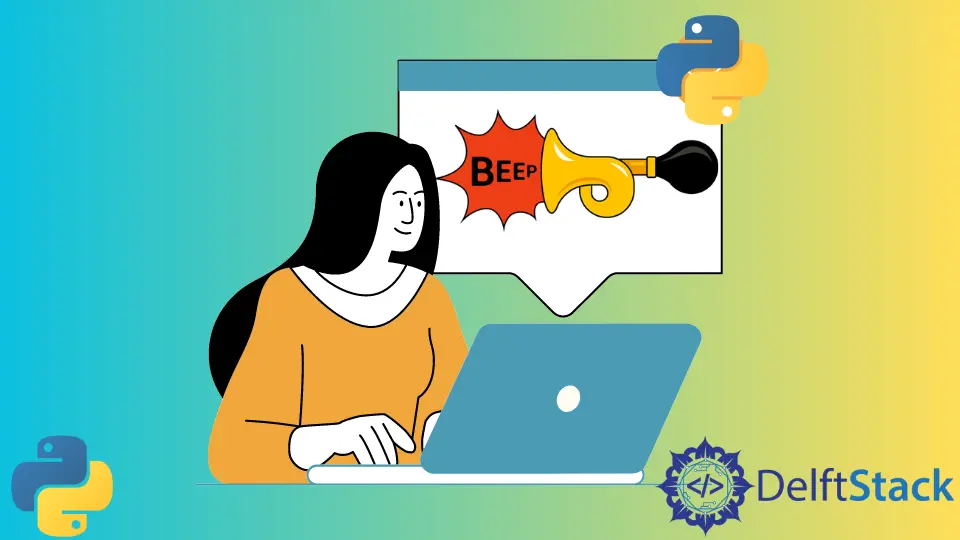
Creating a beep sound in Python is a fun and straightforward task that can be accomplished using various methods. Whether you’re looking to add a simple audio cue to your program or create a more complex sound effect, Python provides several options to suit your needs.
In this tutorial, we will explore different techniques to generate a beep sound, allowing you to enhance your applications with auditory feedback. By the end of this guide, you’ll have the knowledge and skills to implement sound effects in your Python projects effortlessly. Let’s dive in and discover how to bring your Python applications to life with sound!
Using the winsound Library
If you’re working on a Windows machine, the winsound
library is a built-in module that makes it easy to generate simple sounds, including beeps. This library is straightforward to use and requires minimal setup.
Here’s how you can use the winsound
library to create a beep sound:
pythonCopyimport winsound
frequency = 1000 # Set Frequency To 1000 Hertz
duration = 1000 # Set Duration To 1000 ms == 1 second
winsound.Beep(frequency, duration)
Output:
textCopyBeep sound will be heard for 1 second.
The code above imports the winsound
library and then uses the Beep()
function to generate a sound. The frequency
variable determines the pitch of the beep, measured in hertz (Hz), while the duration
variable specifies how long the beep will last, measured in milliseconds. In this example, the beep will sound at 1000 Hz for 1 second. This method is perfect for simple audio cues in your applications.
Using the os System Command
If you’re looking for an alternative method that works across different operating systems, you can use the os
module to execute system commands that generate a beep sound. This method is particularly useful for users who may not have the winsound
library available.
Here’s how you can implement this method:
pythonCopyimport os
# For Windows
os.system('echo \a')
# For Unix/Linux
os.system('printf "\a"')
Output:
textCopyBeep sound will be heard.
In this code snippet, we use the os.system()
function to execute a command that triggers a beep sound. For Windows, the command echo \a
is used, while for Unix/Linux systems, the command printf "\a"
is executed. This method is versatile and works across platforms, making it an excellent choice for cross-compatible applications. However, keep in mind that the output may vary depending on the system’s audio settings.
Using the winsound Library for Continuous Beeping
If you want to create a continuous beep sound, the winsound
library can also accommodate this. By utilizing a loop, you can generate a series of beeps at specified intervals.
Here’s how you can achieve continuous beeping:
pythonCopyimport winsound
import time
frequency = 1000
duration = 500 # 500 ms for half a second
for _ in range(5): # Beep 5 times
winsound.Beep(frequency, duration)
time.sleep(0.5) # Pause for half a second between beeps
Output:
textCopyFive beeps will be heard with a half-second pause in between.
In this example, we set the frequency and duration as before but added a loop that calls the Beep()
function five times. After each beep, the program pauses for half a second using time.sleep()
. This method is great for alert notifications or signaling events in your applications, providing a more dynamic auditory experience.
Using the playsound Library for More Complex Sounds
While the previous methods are excellent for simple beeps, you might want to play more complex sound files, such as .wav or .mp3 formats. The playsound
library is a great option for this purpose. It allows you to play sound files easily and is compatible with various operating systems.
Here’s how to use the playsound
library:
pythonCopyfrom playsound import playsound
# Ensure you have a sound file in the same directory
playsound('your_sound_file.mp3')
Output:
textCopyYour sound file will play.
In this code, we import the playsound
library and call the playsound()
function with the path to your sound file. This method is ideal for playing back more intricate audio clips, such as notifications or background music. Make sure to have the sound file in the same directory as your script or provide the full path to the file.
Conclusion
In this tutorial, we’ve explored various methods to generate beep sounds in Python, from using the built-in winsound
library to executing system commands and playing sound files with the playsound
library. Each method has its advantages, depending on your specific needs and the environment in which you’re working. By integrating sound into your Python applications, you can enhance user experience and provide auditory feedback effectively. Now that you have the tools and knowledge, feel free to experiment with these methods and make your projects more engaging!
FAQ
-
Can I use the winsound library on macOS?
Thewinsound
library is specific to Windows and cannot be used on macOS. For macOS, consider using theos
system command or theplaysound
library. -
What audio formats does the playsound library support?
Theplaysound
library supports various formats, including .mp3 and .wav files, making it versatile for different audio needs. -
Do I need to install any additional libraries for the playsound method?
Yes, you need to install theplaysound
library using pip. You can do this by runningpip install playsound
in your terminal or command prompt. -
How can I adjust the volume of the beep sound?
The volume of the beep sound is controlled by your system’s audio settings, and Python does not provide a direct method to adjust it programmatically. -
Can I create a custom beep sound using the winsound library?
No, thewinsound
library only allows you to generate beeps with specified frequency and duration. For custom sounds, use theplaysound
library with your audio files.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn