B in Front of String in Python
- What Are Bytes Strings?
- Creating Bytes Strings
- Working with Bytes Strings
- Practical Applications of Bytes Strings
- Conclusion
- FAQ
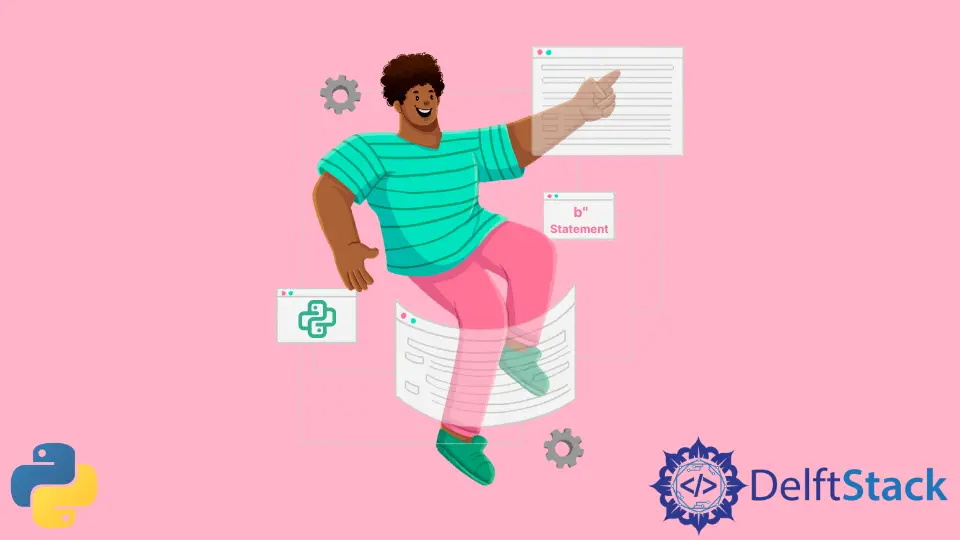
In the world of Python programming, understanding data types is crucial, especially when dealing with strings. One common notation you’ll encounter is the “b” in front of a string, which signifies a bytes string. But what does that mean, and why is it important? Bytes strings are essential for handling binary data, such as files or network communications. They differ from regular strings, which are Unicode by default.
In this article, we’ll explore what bytes strings are, how to create them, and their practical applications in Python programming. By the end, you’ll have a solid grasp of this concept and be ready to implement it in your projects.
What Are Bytes Strings?
Bytes strings in Python are sequences of bytes, which can represent binary data or encoded text. The “b” prefix indicates that the following string is a bytes object. This is particularly useful when you need to work with binary data, such as images or audio files, or when interfacing with network protocols that require data to be sent in bytes.
To create a bytes string, you can simply prepend the letter “b” to a string literal. For example:
byte_string = b"Hello, World!"
Output:
b'Hello, World!'
In this case, byte_string
holds a bytes object that represents the string “Hello, World!” in a byte format. This is different from a regular string, which would look like this:
regular_string = "Hello, World!"
Output:
'Hello, World!'
The primary difference is that byte_string
can only contain ASCII characters, while regular_string
can contain a wider range of characters due to its Unicode nature. Understanding this distinction is vital for tasks involving file I/O, data transmission, or any situation where you need to manipulate raw bytes.
Creating Bytes Strings
Creating bytes strings in Python is straightforward. You can use either a string literal with the “b” prefix or the bytes()
constructor. Let’s explore both methods.
Using the “b” Prefix
As mentioned earlier, the simplest way to create a bytes string is by using the “b” prefix. This method is concise and easy to read. Here’s an example:
byte_string1 = b"Python is great!"
Output:
b'Python is great!'
This creates a bytes object that holds the ASCII representation of the string. You can check the type of this variable using the type()
function:
print(type(byte_string1))
Output:
<class 'bytes'>
Using the bytes()
Constructor
Alternatively, you can use the bytes()
constructor to create a bytes string. This method allows for more flexibility, especially when dealing with non-ASCII characters. Here’s how to do it:
byte_string2 = bytes("Python is fun!", 'utf-8')
Output:
b'Python is fun!'
In this example, the bytes()
constructor takes two arguments: the string you want to convert and the encoding format. In this case, we used ‘utf-8’, which is a popular encoding that supports a wide range of characters. You can also create an empty bytes string like this:
empty_bytes = bytes()
Output:
b''
Using the bytes()
constructor is particularly useful when you need to handle strings with special characters or when you want to convert a list of integers to a bytes object.
Working with Bytes Strings
Once you’ve created bytes strings, you can perform various operations on them. These operations include slicing, concatenation, and encoding/decoding. Let’s dive into these concepts with some examples.
Slicing and Concatenation
Bytes strings support slicing and concatenation just like regular strings. Here’s how you can slice a bytes string:
byte_string = b"Learn Python"
sliced_bytes = byte_string[6:12]
Output:
b'Python'
In this case, we extracted the substring “Python” from the original bytes string. You can also concatenate bytes strings using the +
operator:
greeting = b"Hello, "
name = b"World!"
full_greeting = greeting + name
Output:
b'Hello, World!'
This combines two bytes strings into one. Remember that when working with bytes strings, the result will still be a bytes object.
Encoding and Decoding
Encoding and decoding are crucial when dealing with bytes strings, especially if you need to convert between bytes and regular strings. To decode a bytes string back to a regular string, use the decode()
method:
decoded_string = byte_string.decode('utf-8')
Output:
'Learn Python'
Conversely, if you have a regular string and want to convert it to bytes, you can use the encode()
method:
encoded_bytes = decoded_string.encode('utf-8')
Output:
b'Learn Python'
Understanding how to encode and decode data is essential for ensuring that your applications can handle various data formats, especially when communicating with APIs or processing files.
Practical Applications of Bytes Strings
Bytes strings have numerous practical applications in Python programming. Here are a few scenarios where they come in handy:
File Handling
When reading or writing binary files, such as images or audio files, you need to work with bytes strings. For instance, when opening a file in binary mode, you can read its content as bytes:
with open('example.png', 'rb') as file:
file_content = file.read()
Output:
b'\x89PNG...'
This allows you to manipulate the binary data directly.
Networking
When working with network protocols, data is often transmitted as bytes. For example, when sending data over a socket, you must convert your strings to bytes:
import socket
s = socket.socket()
s.connect(('example.com', 80))
s.send(b'GET / HTTP/1.1\r\nHost: example.com\r\n\r\n')
Output:
b'HTTP/1.1 200 OK...'
In this case, the HTTP request is sent as a bytes string, ensuring proper communication with the server.
Data Serialization
Bytes strings are also useful in data serialization formats like JSON or Protocol Buffers. When sending data over the network or saving it to a file, you often convert your data structures to bytes for efficient storage and transmission.
import json
data = {'name': 'Alice', 'age': 30}
json_data = json.dumps(data).encode('utf-8')
Output:
b'{"name": "Alice", "age": 30}'
This example shows how to serialize a Python dictionary to a JSON-formatted bytes string.
Conclusion
Understanding the “b” notation in Python is essential for any programmer dealing with binary data or network communications. Bytes strings offer a powerful way to handle raw data, ensuring that your applications can efficiently read, write, and transmit information. By mastering the creation, manipulation, and practical applications of bytes strings, you’ll be well-equipped to tackle various programming challenges. Whether you’re working with files, networking, or data serialization, bytes strings are an invaluable tool in your Python toolkit.
FAQ
-
What is the difference between bytes and strings in Python?
bytes are sequences of bytes, while strings are sequences of Unicode characters. Bytes can only contain ASCII characters, whereas strings can contain a wider range of characters. -
How do I convert a string to bytes in Python?
You can convert a string to bytes using theencode()
method or by prepending the “b” prefix to the string literal. -
Can I slice bytes strings in Python?
Yes, bytes strings support slicing just like regular strings. You can extract a portion of a bytes string using indexing. -
What encoding should I use for bytes strings?
The most commonly used encoding is ‘utf-8’, as it supports a wide range of characters. However, you can use other encodings depending on your needs. -
How do I read a binary file in Python?
You can read a binary file by opening it in binary mode using the ‘rb’ flag, allowing you to read its content as bytes.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn