How to Address Already in Use Error in Python
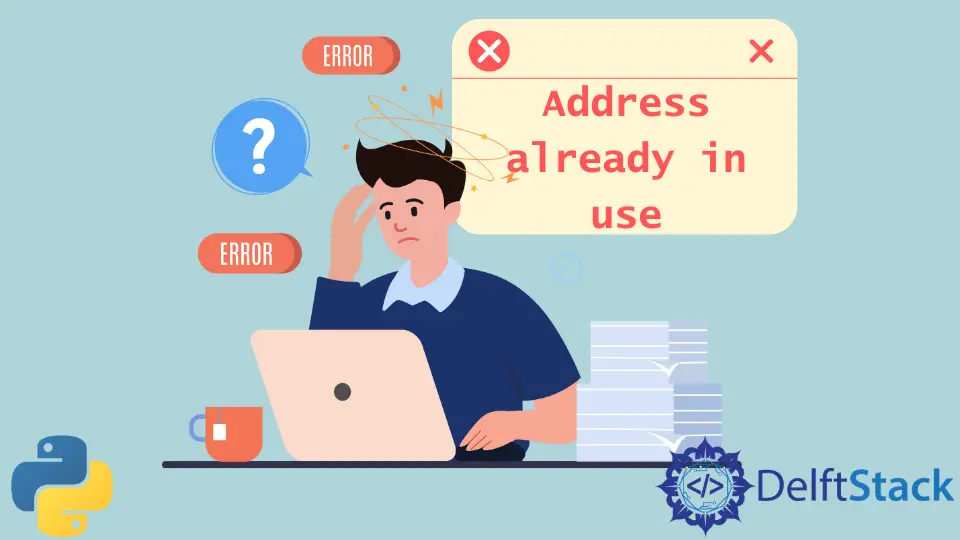
We will introduce when the error Address already in use
occurs in Python and how to solve it with examples.
Address already in use
Error in Python
This article will teach the Python stack error that occurs when running a program that uses a port. We will learn why this error occurs and how to resolve it and make your program run smoothly.
This error occurs when we are trying to access a port already in use and cannot be freed for the program we are trying to use.
Now, let’s discuss how we can resolve this error. Many methods resolve this error, but we will learn a few methods with examples.
In Python, if we create a program that runs over a server and has to perform some tasks over a server, this error can occur. Let’s discuss how to resolve this error.
As shown below, we will write the port number after the following command, which becomes available.
# python
python -m SimpleHTTPServer (443)
When we run this command and run our program again, we will use port 443
to perform some tasks. It will run smoothly without any problem because now the port is available for usage.
If this method still doesn’t help us run our program, we can use another method that can surely free up the taken port with some steps.
First, we will run the following command to locate and list the processes using the port, as shown below.
ps -fA | grep python
If this command was successful, we could see the process codes in the argument. If the argument displays multiple processes running on the port, we can spot the process that is blocking the port by searching for the SimpleHTTPServer
process, as shown below.
# python
443 89330 12879 0 1:53AM ttys00 0:00.15 python -m SimpleHTTPServer
We will check for the port that the process is using, and we will use it to kill the process with the code and free up the port by using the following command as shown below.
kill 89330
It will kill the process and free up the port. If the process is still not responding, we can use a tougher command below.
sudo kill -9 89330
Once the process is killed and your port is free, we can bind up the freed port using the following command.
# python
python -m SimpleHTTPServer (443)
So following these steps, we can free up the port and get rid of the socket error Address already in use
in Python.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python