How to Fix Bytes-Like Object Is Required Not STR Error in Python
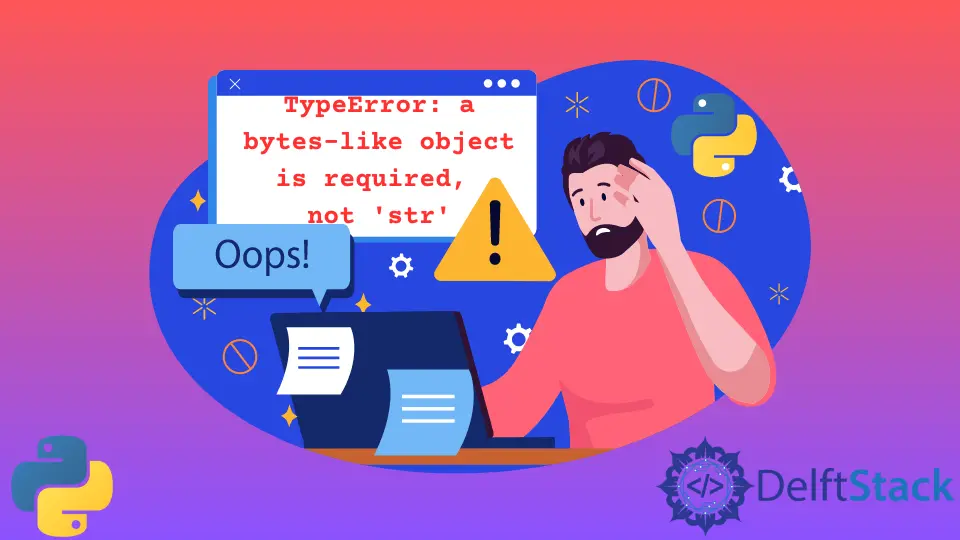
This tutorial will discuss the error a bytes-like object is required, not 'str'
in Python, and ways to fix it. This TypeError
shows when an invalid operation is done on the wrong data type.
We will discuss string and bytes objects in Python. Strings are a collection of characters, whereas the latter is a sequence of bytes, also called Unicode
objects. In Python3, all strings are Unicode
objects by default. In Python 2, we can convert strings to Unicode
and vice-versa using the encode
and decode
functions.
We get this error when working with a bytes object but treating it as a string. It is common due to the change of these objects in Python 2 and Python 3. We get this error while working with a binary file and treat it as a string.
For example:
with open("myfile.txt", "rb") as f:
a = f.read()
print(type(a))
a.split(";")
Output:
TypeError: a bytes-like object is required, not 'str'
In the example above, we read a file in rb
mode. This mode means reading a binary file. The contents of this are bytes and stored in variable a
, and we display the type.
When we apply the split()
function to this variable, we get a bytes-like object is required, not 'str'
error. It’s because the split()
function works with string objects.
To avoid this error, beware of the data read type and its operations. We can also fix this error by converting the bytes-like object to string using the str()
function.
For example:
with open("myfile.txt", "rb") as f:
a = str(f.read())
print(type(a))
s = a.split(";")
Output:
<class 'str'>
The str()
converts the object to a string to use the split()
function.
This error is also frequent when working with sockets and sending or receiving data. We can use the b
character before a string to send bytes or the encode()
function with the utf-8
parameter.
For example:
data = b"result"
s.sendall(data)
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python