The __file__ Variable in Python
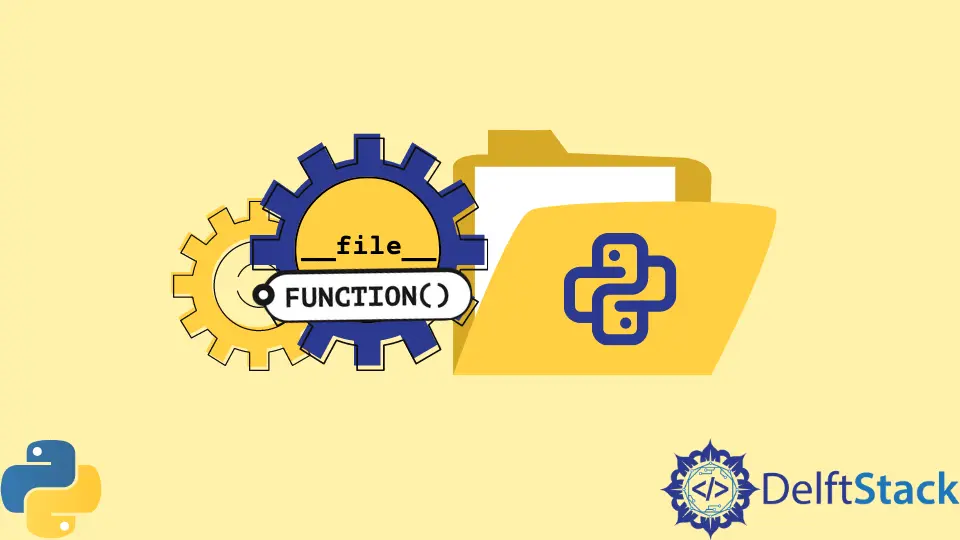
This tutorial will discuss the __file__
variable in Python.
the __file__
Variable in Python
The double underscore surrounding the name of some variables and methods is also known as a dunder in Python. Any variable or method whose name is surrounded by a dunder is a special variable or method by convention. The __file__
variable is also a special variable used to get the exact path of any modules imported into our code. The following code snippet below shows us how to get the path of an imported module with the __file__
variable.
import os
print(os.__file__)
Output:
/usr/lib/python3.7/os.py
We printed the file’s path containing the os
module with the __file__
special variable in the code above; this can also be used for user-generated modules. The following code snippets show us how to use the __file__
variable with user-generated modules.
hello.py
file:
def printHello():
print("Hello World")
main.py file:
import hello as h
h.printHello()
print(h.__file__)
Output:
Hello World
/content/hello.py
We used the __file__
special variable to get the path of the user-generated module hello
in the code above. First, we created the hello.py
file that contains the printHello()
method, which prints Hello World
in the console.
Then, we imported the hello
module inside our main.py
file and called the h.printHello()
method. In the end, we printed the path of the file containing the hello
module with the print(h.__file__)
method.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn