Python Pyserial Readline
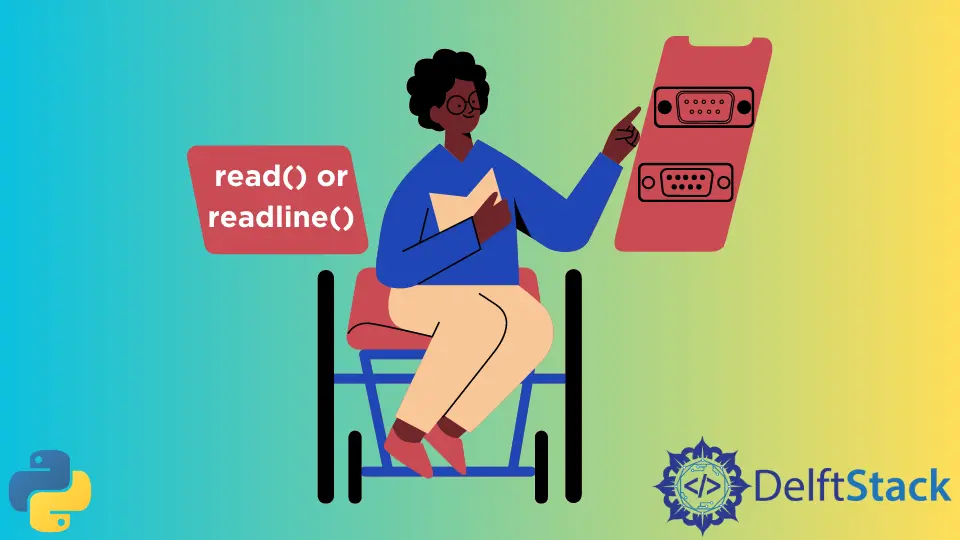
This tutorial will introduce how to use the read()
or readline()
function in Python serial
module.
The read()
and readline()
functions are an essential part of Python’s serial
module. The serial
module provides all the functions and necessities required for accessing the serial port.
Essentially, it can be said that the serial
module supplies the backends for Python that runs on Linux, Windows, OSX, etc. In simple terms, it means that the serial
automatically chooses the backend that it finds to be appropriate.
Let us begin with the read()
function and its application when we need to read more than a single character at a time. The read()
function of the serial
module is utilized to read the given text one byte at a time. It contains a parameter that denotes the count of the maximum amount of bytes
we want the function to read.
The following program uses the read()
function to read more than a single character at a time.
# general code of the serial module
import serial
ser = serial.Serial()
ser.port = "COM2"
ser.baudrate = 19200
ser.timeout = 0
x = ser.read() # This function will read one byte from the given variable.
Similarly, we can use the readline()
function. It has a working which is pretty similar to the read()
function, but instead, it reads a whole line at a time.
However, the timeout needs to be defined to implement the readline()
function properly. Moreover, the readline()
function stops reading one line only after it encounters the end of line or eol
, which is the \n
newline character, so it is essential to apply that to every line while using this function.
The following code uses the readline()
function to read more than a single character at a time.
# general code of the serial module
import serial
ser = serial.Serial()
ser.port = "COM2"
ser.baudrate = 19200
ser.timeout = 0
line = ser.readline() # This function reads one line at a time.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn