How to Use pydoc in Python
- What is pydoc?
- Using pydoc from the Command Line
- Generating Documentation for Your Own Modules
- Accessing pydoc in an Interactive Python Shell
- Creating Custom Documentation with pydoc
- Conclusion
- FAQ
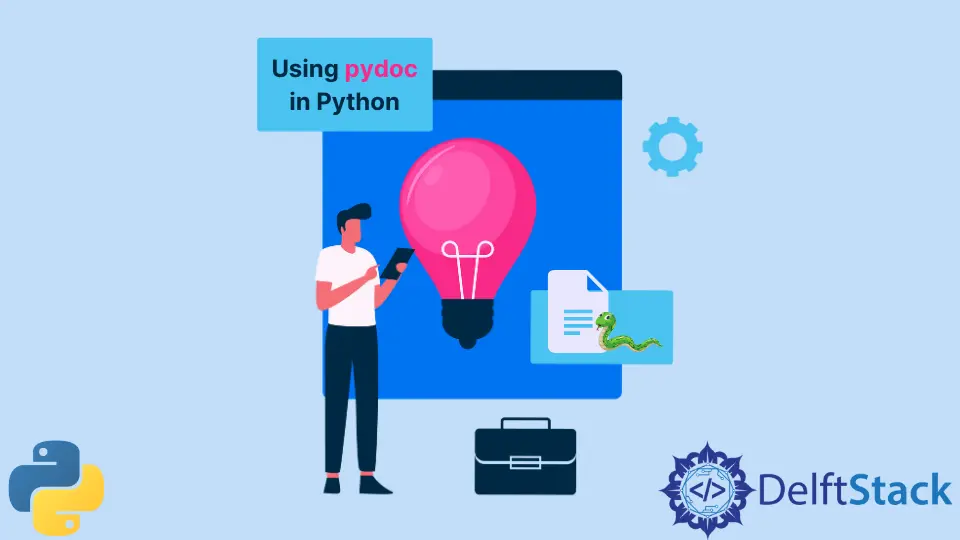
When it comes to Python programming, understanding how to effectively document your code is crucial. Enter the pydoc
package, a built-in tool that provides a straightforward way to generate documentation for your Python modules, functions, and classes. Whether you’re a beginner looking to enhance your coding skills or an experienced developer wanting to streamline your documentation process, this tutorial will guide you through the essentials of using pydoc
. We will explore various methods to utilize this powerful tool, complete with code examples and detailed explanations. By the end of this article, you’ll be well-equipped to create clear and concise documentation that enhances the readability and usability of your Python projects.
What is pydoc?
Before diving into the practical applications of pydoc
, it’s essential to understand what it is. pydoc
is a module in Python that automatically generates documentation for your Python code. It can extract docstrings from your code and present them in a user-friendly format. The beauty of pydoc
lies in its simplicity and effectiveness. You can use it directly in the command line or within your Python scripts, making it a versatile option for developers.
Using pydoc from the Command Line
One of the easiest ways to utilize pydoc
is through the command line. This method allows you to quickly generate documentation for any installed module. To do this, simply open your terminal and type the following command:
pydoc <module_name>
For example, if you want to generate documentation for the built-in math
module, you would enter:
pydoc math
Output:
Help on module math:
NAME
math - This module provides access to the mathematical functions
defined by the C standard.
...
When you run this command, pydoc
retrieves the documentation for the specified module and displays it in your terminal. This is particularly useful for quickly referencing functions, classes, and methods without needing to browse through extensive online documentation. You can also use pydoc
to generate HTML documentation by appending the -w
flag, as shown below:
pydoc -w <module_name>
This will create an HTML file containing the documentation, which you can view in any web browser. This method is ideal for those who prefer visual documentation or need to share it with others.
Generating Documentation for Your Own Modules
In addition to built-in modules, pydoc
can also be used to document your own Python files. To demonstrate this, let’s create a simple Python module named calculator.py
:
def add(a, b):
"""Return the sum of a and b."""
return a + b
def subtract(a, b):
"""Return the difference of a and b."""
return a - b
Once you have your module ready, you can generate documentation using pydoc
in the following way:
pydoc calculator
Output:
Help on module calculator:
NAME
calculator
FUNCTIONS
add(a, b)
Return the sum of a and b.
subtract(a, b)
Return the difference of a and b.
By running this command, pydoc
extracts the docstrings from your module and presents them in a clear format. This is an excellent way to ensure your code is well-documented, making it easier for others (and yourself) to understand its functionality in the future.
Accessing pydoc in an Interactive Python Shell
Another effective way to use pydoc
is within an interactive Python shell. This method is perfect for those who prefer a more hands-on approach. To start, open your Python interpreter by typing python
or python3
in your terminal. Once inside, you can import pydoc
and use its functions directly.
Here’s how you can do it:
import pydoc
help(math)
Output:
Help on module math:
NAME
math - This module provides access to the mathematical functions
defined by the C standard.
...
By calling the help()
function and passing in the module name, pydoc
will display the documentation for that module right in your interactive session. This is particularly useful for quick lookups while coding. You can also access documentation for functions and classes in the same way:
help(calculator.add)
Output:
Help on function add in module calculator:
NAME
add
DESCRIPTION
Return the sum of a and b.
Using pydoc
in an interactive shell provides immediate access to documentation, making it a great tool for learning and experimentation.
Creating Custom Documentation with pydoc
If you want to create custom documentation that includes more than just the default output from pydoc
, you can utilize the pydoc.render_doc()
function. This allows you to format the output in a more personalized way. Here’s a quick example:
import pydoc
doc = pydoc.render_doc(calculator)
print(doc)
Output:
Help on module calculator:
NAME
calculator
FUNCTIONS
add(a, b)
Return the sum of a and b.
subtract(a, b)
Return the difference of a and b.
By calling pydoc.render_doc()
with your module as an argument, you can generate a string that contains the documentation. You can then manipulate this string further, save it to a file, or print it as shown. This flexibility allows you to create documentation that fits your specific needs, whether for personal use or for sharing with others.
Conclusion
The pydoc
package is an invaluable tool for any Python developer looking to streamline their documentation process. By utilizing the command line, interactive shell, or custom documentation methods, you can easily generate clear and concise documentation for both built-in and custom modules. This not only enhances the usability of your code but also promotes better collaboration and understanding among team members. As you continue your Python journey, incorporating pydoc
into your workflow will undoubtedly elevate your programming skills and improve the quality of your projects.
FAQ
-
what is pydoc used for?
pydoc is used to generate documentation for Python modules, functions, and classes, helping developers understand and use code more effectively. -
can I use pydoc for my own Python scripts?
yes, you can use pydoc to document your own Python scripts by running it from the command line or within an interactive shell.
-
how do I create HTML documentation with pydoc?
to create HTML documentation, use the commandpydoc -w <module_name>
, which will generate an HTML file for the specified module. -
is pydoc included with Python?
yes, pydoc is a built-in module in Python, so you don’t need to install anything extra to use it. -
can I customize the output of pydoc?
yes, you can customize the output by using thepydoc.render_doc()
function to format and manipulate the documentation string as needed.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn