How to Print Blank Line in Python
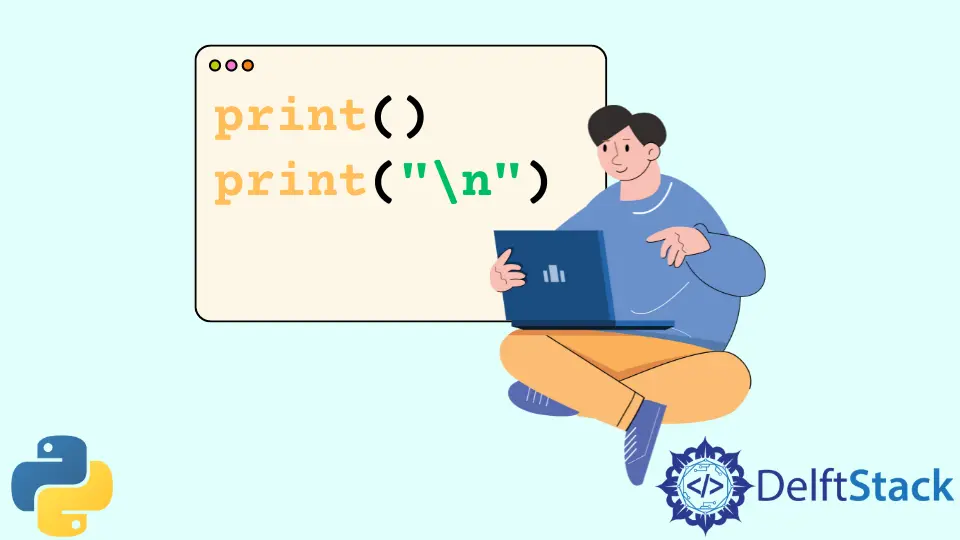
In this tutorial, we will be discussing how to print a blank line in Python.
Before getting to this, it should be noticed that in Python the \n
character represents a newline.
In the print()
function, there is an argument called end
. This argument is \n
by default. It means that this character gets written to the output stream at the end of the print statement.
That is why if we use multiple print statements, the content in each statement will be printed in a different line.
Take the following code as an example.
print("Hello")
print("How are you?")
Output:
Hello
How are you?
We can alter this end
argument to any character we desire.
Taking everything said above into consideration, it can be understood that an empty print
statement can be used to print a blank line by default.
For example,
print("Hello")
print() # Blank Line
print("How are you?")
Output:
Hello
How are you?
Since in Python 2, there was no parenthesis used with the print
statement, so in that version, the print
command will print a blank line. If we print only the \n
character, it will print two blank lines.
However, if the end
parameter is explicitly specified something else, then the \n
character will be required.
For example,
print("Hello", end=" ")
print("\n") # Blank Line
print("How are you?", end=" ")
Output:
Hello
How are you?
In the above code, if we only used the print()
statement instead of print("\n")
, then it would not have printed a blank line.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn