How to Print an Exception in Python
- Method 1: Using the Basic Try-Except Block
- Method 2: Printing Exception Tracebacks
- Method 3: Custom Exception Handling
- Conclusion
- FAQ
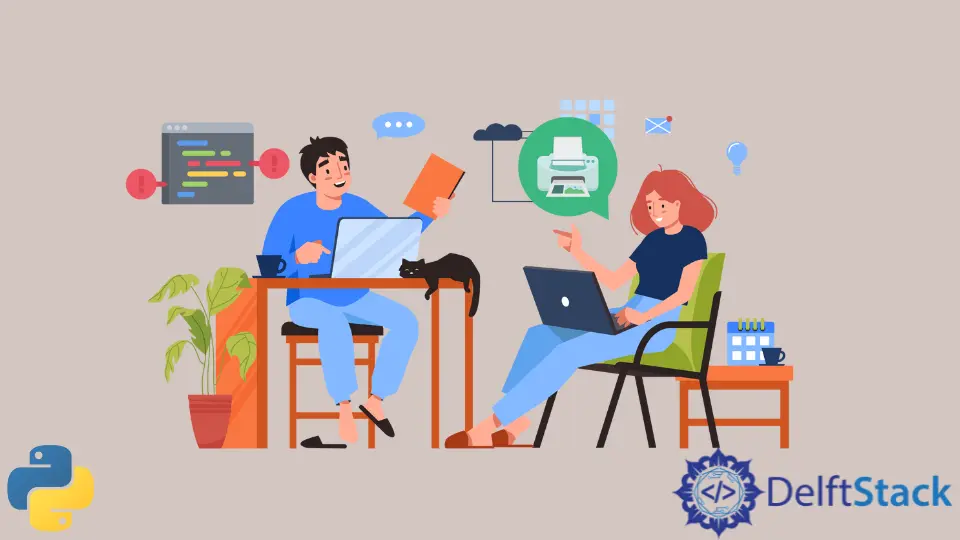
When working with Python, exceptions are a common occurrence, and knowing how to handle and print them effectively is crucial for debugging and maintaining your code. Printing exceptions can provide valuable insights into what went wrong, helping you identify issues and fix them promptly.
In this article, we’ll explore several methods for printing exceptions in Python, ensuring you have the tools you need to manage errors gracefully. Whether you’re a beginner or an experienced developer, understanding how to print exceptions will enhance your coding skills and improve your overall programming experience. Let’s dive in!
Method 1: Using the Basic Try-Except Block
The simplest way to print an exception in Python is by using a try-except block. This method allows you to catch exceptions and handle them gracefully without crashing your program. Here’s how it works:
try:
result = 10 / 0
except Exception as e:
print("An error occurred:", e)
Output:
An error occurred: division by zero
In this example, we attempt to divide by zero, which raises a ZeroDivisionError
. The try
block contains the code that may raise an exception, while the except
block captures the exception. The variable e
holds the exception details, which we print to the console. This method is straightforward and effective for basic error handling.
Using this approach, you can easily identify the type of error and its message. It’s particularly useful during development, as it allows you to see what went wrong without stopping the execution of your program. You can also customize the error message or take different actions based on the type of exception caught. This flexibility makes the try-except block a fundamental tool in Python programming.
Method 2: Printing Exception Tracebacks
For more detailed information about exceptions, you can print the traceback. This method provides a complete stack trace, which is incredibly useful for debugging complex applications. Here’s how you can do it:
import traceback
try:
result = [1, 2][3]
except Exception as e:
print("An error occurred:")
traceback.print_exc()
Output:
An error occurred:
Traceback (most recent call last):
File "script.py", line 4, in <module>
result = [1, 2][3]
IndexError: list index out of range
In this example, we attempt to access an index that doesn’t exist in a list, raising an IndexError
. By importing the traceback
module and calling traceback.print_exc()
, we get a detailed traceback of the error. This includes the file name, line number, and the exact error message.
Using tracebacks is especially beneficial when you’re dealing with larger codebases or when an error occurs deep within nested function calls. It allows you to trace back the steps leading to the exception, making it easier to diagnose the problem. This method is invaluable for developers who need to understand the context of an error fully.
Method 3: Custom Exception Handling
Sometimes, you may want to create your own custom exceptions to handle specific error situations. This approach allows you to provide more context and clarity about the errors in your code. Here’s how to define and print custom exceptions:
class CustomError(Exception):
pass
try:
raise CustomError("This is a custom error message")
except CustomError as e:
print("Caught a custom exception:", e)
Output:
Caught a custom exception: This is a custom error message
In this example, we define a custom exception class named CustomError
. We then raise this exception within the try block. The except block catches our custom exception, and we print a relevant message. This method enhances code readability and allows you to categorize different types of errors more effectively.
Custom exceptions are particularly useful in larger applications where standard exceptions may not provide enough context. By using custom exceptions, you can create specific error messages that make it clear what went wrong, leading to faster debugging and resolution of issues.
Conclusion
Printing exceptions in Python is a vital skill that can significantly improve your programming experience. Whether you’re using basic try-except blocks, printing detailed tracebacks, or defining custom exceptions, each method has its unique advantages. By mastering these techniques, you’ll be better equipped to handle errors and troubleshoot issues in your code. Remember, effective error handling not only enhances your code’s reliability but also makes it easier for others to understand and maintain. Happy coding!
FAQ
-
How do I print the type of an exception in Python?
You can use thetype()
function within the except block to print the type of an exception. For example:print("Error type:", type(e))
. -
Can I catch multiple exceptions in a single except block?
Yes, you can catch multiple exceptions by specifying them as a tuple in the except statement. For example:except (TypeError, ValueError) as e:
.
-
What is the difference between Exception and BaseException in Python?
BaseException
is the base class for all exceptions in Python, whileException
is the base class for most built-in exceptions. It is generally recommended to catchException
to avoid inadvertently catching system-exiting exceptions. -
How can I log exceptions instead of printing them?
You can use thelogging
module to log exceptions. Uselogging.exception("Error occurred")
within the except block to log the error with traceback. -
Is it a good practice to use bare except statements?
No, using bare except statements is generally discouraged because it can catch unexpected exceptions and make debugging difficult. Always specify the exception type you want to catch.